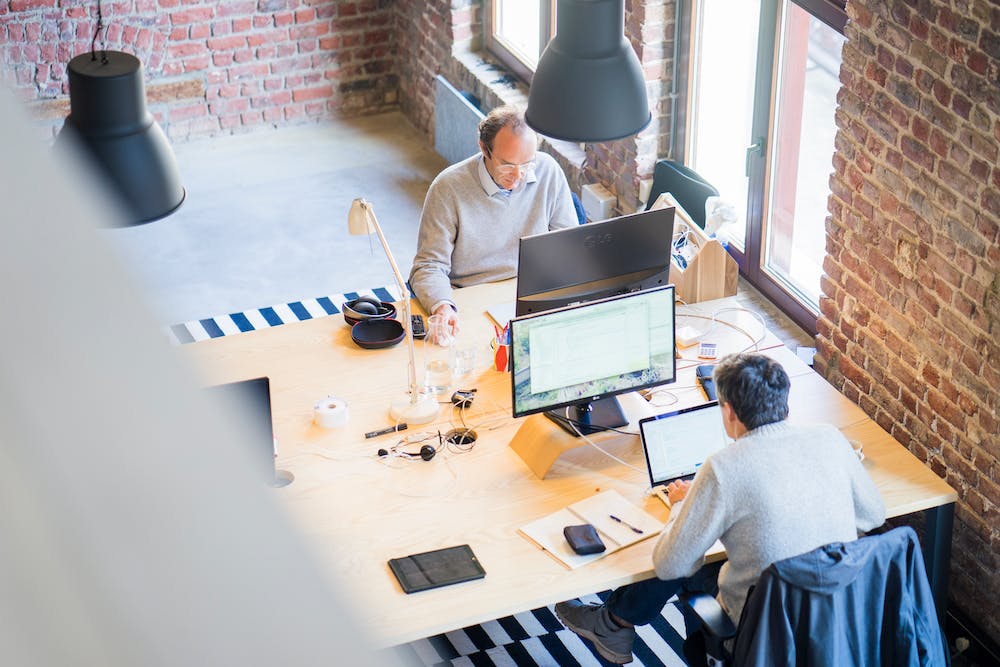
PHP is a powerful and popular scripting language that is used to create dynamic websites. With PHP, developers can add functionality to websites, interact with databases, and create dynamic content. Understanding the fundamentals of PHP is essential for anyone looking to build dynamic websites. In this article, we will explore the basics of PHP and how IT is used to create dynamic websites.
The Basics of PHP
PHP is a server-side scripting language that is used to create dynamic web pages. IT is embedded within HTML code and is executed on the server, generating HTML to be sent to the client’s web browser. This allows for the creation of dynamic, interactive web pages that can respond to user input and interact with databases.
One of the key features of PHP is its ability to interact with databases. Using PHP, developers can connect to a database, retrieve data, and display IT on a web page. This makes PHP a powerful tool for creating dynamic websites that can store and display large amounts of data.
Basic Syntax
PHP code is enclosed within tags, allowing IT to be embedded within HTML. PHP statements end with a semicolon (;) and can span multiple lines. Here is an example of a simple PHP script that outputs “Hello, World!”:
echo "Hello, World!";
?>
Variables and Data Types
PHP supports a variety of data types, including strings, integers, floats, and arrays. Variables in PHP are preceded by a dollar sign ($), and their data type is determined dynamically based on the value assigned to them. Here is an example of variable declaration and assignment in PHP:
$name = "John Doe";
$age = 30;
?>
Creating Dynamic content with PHP
One of the most common uses of PHP is to create dynamic content on web pages. This can be accomplished using PHP to generate HTML based on user input or database queries. For example, a simple PHP script can be used to create a dynamic greeting based on the time of day:
$time = date("H");
if ($time < "12") {
echo "Good morning!";
} elseif ($time < "18") {
echo "Good afternoon!";
} else {
echo "Good evening!";
}
?>
Another common use of PHP is to create forms that allow users to interact with a Website. PHP can process form data and use IT to generate dynamic content or interact with a database. Here is an example of a simple contact form using PHP:
In this example, the form data is sent to a PHP script called “process_form.php” which can process the input and take appropriate action, such as sending an email or saving IT to a database.
Interacting with Databases
PHP is often used to interact with databases, allowing for the storage and retrieval of data on a Website. PHP provides a variety of database extensions, such as MySQLi and PDO, that allow for the connection and interaction with databases. Here is an example of connecting to a MySQL database using PHP:
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDB";
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
?>
Incorporating PHP into HTML
PHP can be embedded within HTML code, allowing for dynamic content to be generated and displayed on a web page. This can be done using PHP tags to enclose PHP code within an HTML document. Here is an example of using PHP to create a simple dynamic web page:
Dynamic Page