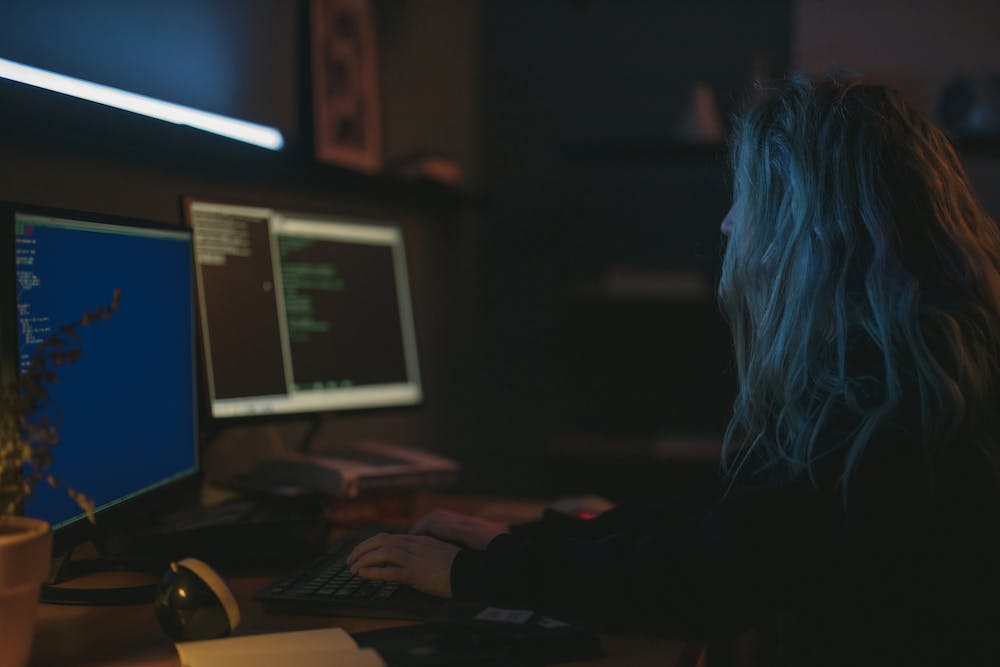
Are you interested in learning how to create your own text-based adventure game in Python? This tutorial will guide you through the process, from setting up your development environment to coding the game mechanics. By the end of this tutorial, you will have a fully functional text-based adventure game that you can share with your friends. So, let’s get started!
Setting up your development environment
Before we begin coding our game, we need to set up our development environment. We’ll be using Python, so make sure you have Python installed on your computer. You can download Python from the official Website and follow the installation instructions for your operating system.
Once you have Python installed, you’ll also need a code editor to write your Python code. There are many code editors available, but some popular choices include Visual Studio Code, Sublime Text, and PyCharm. Choose a code editor that you’re comfortable with and install IT on your computer.
Creating the game structure
Now that we have our development environment set up, let’s start by defining the structure of our game. A text-based adventure game typically consists of a series of interconnected locations, each with its own description and possible actions for the player to take. We can represent these locations using a graph, where each node represents a location and each edge represents a possible transition between locations.
For example, consider a simple game with three locations: a forest, a cave, and a castle. We can represent the connections between these locations as follows:
forest --(path 1)--> cave
cave --(path 2)--> castle
In this game, the player starts in the forest and can choose to go to the cave or take some other action in the forest. From the cave, the player can choose to go to the castle or take some other action in the cave. And so on.
Coding the game mechanics
Now that we have our game structure in mind, we can start coding the game mechanics. We’ll use Python’s object-oriented programming features to represent the game locations and the player’s actions.
First, let’s create a class to represent the game locations:
“`python
class Location:
def __init__(self, name, description):
self.name = name
self.description = description
self.connections = {}
def add_connection(self, location, action):
self.connections[action] = location
“`
In this class, we have a constructor that initializes the location with a name and a description. We also have a method for adding connections to other locations, which takes another location and an action as its parameters.
Next, let’s create a class to represent the player:
“`python
class Player:
def __init__(self, current_location):
self.current_location = current_location
def move(self, action):
if action in self.current_location.connections:
self.current_location = self.current_location.connections[action]
else:
print(“You can’t go that way.”)
“`
This class has a constructor that initializes the player with a starting location, and a method for moving the player to a new location based on the player’s chosen action.
Putting it all together
With our classes defined, we can now create our game world and start the game loop. Here’s a simple example of how to do this:
“`python
# Create the game locations
forest = Location(“Forest”, “You are in a dark, eerie forest.”)
cave = Location(“Cave”, “You are in a damp, dimly lit cave.”)
castle = Location(“Castle”, “You are in a majestic, towering castle.”)
# Connect the locations
forest.add_connection(cave, “go to the cave”)
cave.add_connection(castle, “go to the castle”)
# Create the player
player = Player(forest)
# Game loop
while True:
print(player.current_location.description)
action = input(“What do you want to do? “)
player.move(action)
“`
In this example, we create the game locations, connect them together, create the player, and then enter a loop where we repeatedly print the player’s current location and ask the player for their next action. The player’s chosen action determines their next location, and the game loop continues until the player decides to quit the game.
Conclusion
Creating a text-based adventure game in Python is a fun and rewarding exercise for beginners. By following this tutorial, you’ve learned how to set up your development environment, define the game structure, code the game mechanics, and put it all together into a playable game. I hope you’ve enjoyed this tutorial and that it has inspired you to explore more game development in Python.
FAQs
Q: What resources can I use to learn more about Python game development?
A: There are many resources available online for learning Python game development, including tutorials, online courses, and community forums. Some popular resources include the Python Game Development subreddit, the Python Game Development Wiki, and the book “Invent Your Own Computer Games with Python” by Al Sweigart.
Q: Can I add more complex features to my text-based adventure game, like combat or inventory management?
A: Yes, you can definitely add more complex features to your game as you become more comfortable with Python programming. Combat mechanics, inventory management, and other RPG elements are common additions to text-based adventure games and can make your game more engaging for players.
Q: How can I make my game more visually appealing?
A: While text-based adventure games are inherently simple in terms of graphics, you can still enhance the visual appeal of your game by using ASCII art, color-coding, and text formatting. You can also explore libraries like curses or Pygame for creating more visually interactive text-based games.
Q: Can I publish and share my text-based adventure game with others?
A: Yes, you can share your game with others by creating a standalone executable using tools like PyInstaller. You can also share your game online through platforms like itch.io or Game Jolt to reach a wider audience.
Now that you’ve completed this beginner’s tutorial, you have the foundation to create your own text-based adventure game in Python. Take this knowledge and let your creativity flow as you embark on your game development journey!