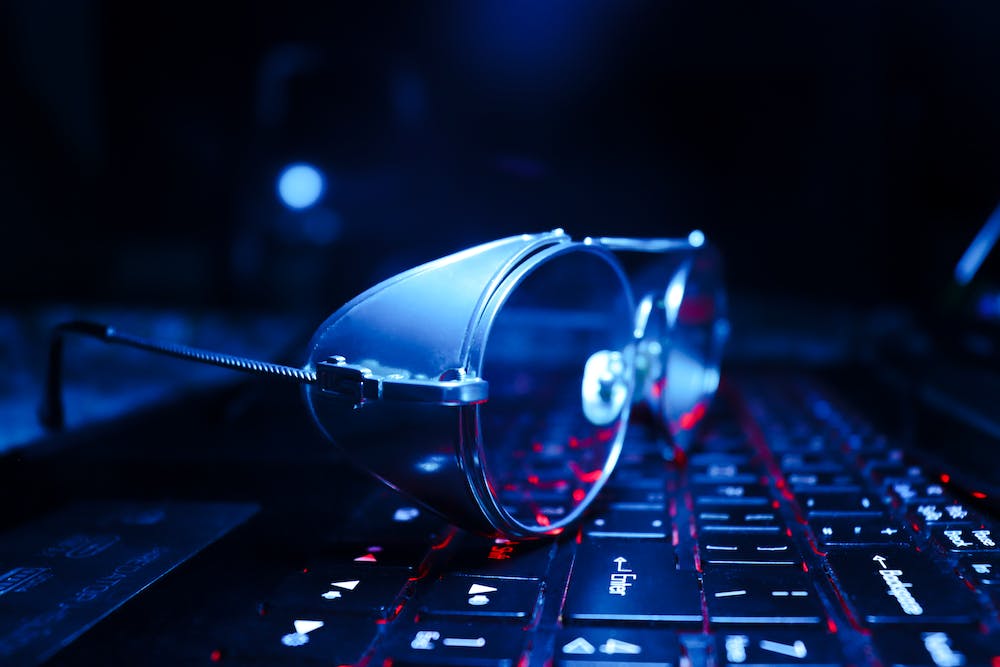
JavaScript is a powerful language that allows developers to create interactive and dynamic web pages. However, there are times when developers need to create a pause or delay in their code. While JavaScript doesn’t have a built-in “wait” or “pause” function, there are ways to achieve this using setTimeout or setInterval methods. In this article, we will explore how to create a one-second wait function in JavaScript and discuss its applications.
Using setTimeout for Pause in JavaScript
The setTimeout method is commonly used to set a timer which executes a function or code snippet after a specified delay. This can be used to create a pause in JavaScript by setting a timeout for one second. Here’s an example of how to create a one-second wait function using setTimeout:
function oneSecondWait() {
setTimeout(function(){
// Your code to be executed after 1 second
}, 1000);
}
Applications of One-Second Wait Function
The one-second wait function can be used in various scenarios to enhance user experience and improve the functionality of web applications. Some common applications include:
- Creating a delay before displaying a pop-up or notification
- Implementing a smooth transition or animation on web elements
- Adding a delay between consecutive API calls to prevent overloading the server
- Triggering an action after a specified time interval
Example: Adding a Pause to an Alert Box
Let’s consider an example where we want to display an alert box after a one-second delay. We can use the one-second wait function to achieve this:
function showAlertWithDelay() {
setTimeout(function(){
alert('Hello, world!');
}, 1000);
}
Conclusion
In conclusion, the one-second wait function in JavaScript can be implemented using the setTimeout method to create a pause or delay in code execution. This can be useful in various scenarios, such as implementing smooth transitions, delaying API calls, or triggering actions after a specified time interval. By understanding how to create a pause in JavaScript, developers can enhance the user experience and add functionality to web applications.
FAQs
Q: Can I use setInterval instead of setTimeout for creating a pause?
A: Yes, setInterval can also be used to create a pause in JavaScript by repeatedly executing a function at specified intervals. However, setTimeout is more suitable for creating a one-time delay.
Q: Is the one-second wait function suitable for all applications?
A: While the one-second wait function can be useful in many scenarios, IT‘s important to consider the specific requirements of your application and choose the appropriate timing for the pause or delay.