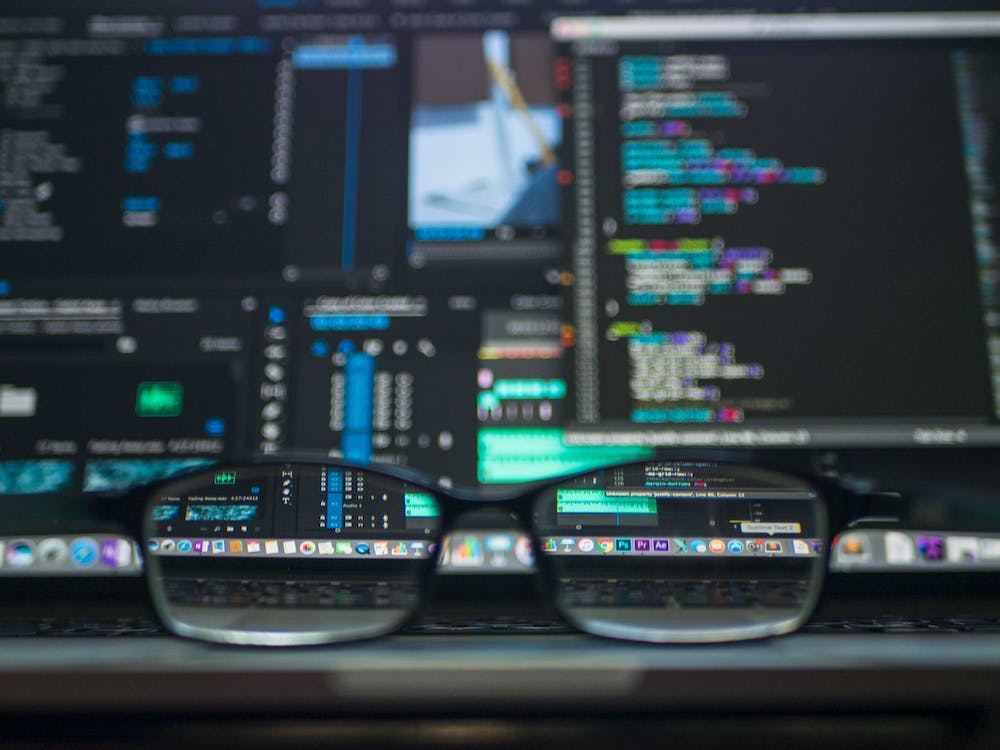
Mobile app development has become an integral part of the modern digital landscape. Whether IT‘s for iOS or Android, businesses and developers are constantly looking for efficient ways to build powerful and scalable mobile applications. One way to achieve this is by creating a Laravel API for mobile app development.
Laravel is a popular PHP framework that provides a robust and flexible environment for building APIs. When creating a Laravel API for mobile app development, developers can leverage the power of Laravel to build secure, scalable, and efficient APIs that can be seamlessly integrated into mobile applications.
Why Create a Laravel API for Mobile App Development?
There are several reasons why creating a Laravel API for mobile app development can be beneficial:
- Scalability: Laravel provides a scalable environment for building APIs, allowing developers to handle a large number of requests and users.
- Security: Laravel offers built-in security features such as encryption, authentication, and authorization, which are crucial for protecting sensitive data in mobile applications.
- Performance: Laravel’s performance optimization features such as caching and database query optimization can help improve the performance of mobile applications.
- Flexibility: Laravel’s modular structure makes it easy to add new features and make changes to the API without affecting the overall functionality of the mobile application.
Steps to Create a Laravel API for Mobile App Development
Creating a Laravel API for mobile app development involves several steps, including setting up a Laravel project, defining routes, creating controllers, and handling authentication. Here’s a step-by-step guide to creating a Laravel API:
Step 1: Set up a Laravel Project
The first step in creating a Laravel API is to set up a new Laravel project. This can be done using the Composer package manager by running the following command in the terminal:
composer create-project --prefer-dist laravel/laravel projectName
Once the project is set up, navigate to the project directory and start the Laravel development server by running the following command:
php artisan serve
Step 2: Define Routes
Routes define the entry points for the API endpoints. In Laravel, routes are defined in the routes/api.php
file. Here’s an example of how routes can be defined:
Route::get('/users', 'UserController@index');
Route::post('/users', 'UserController@store');
Route::get('/users/{id}', 'UserController@show');
Route::put('/users/{id}', 'UserController@update');
Route::delete('/users/{id}', 'UserController@destroy');
Step 3: Create Controllers
Controllers handle the logic for each API endpoint. In Laravel, controllers are created using the php artisan make:controller
command. Here’s an example of a UserController that handles user-related API requests:
php artisan make:controller UserController
Once the controller is created, define the methods to handle the API requests, such as index, store, show, update, and destroy.
Step 4: Handle Authentication
Authentication is a crucial aspect of mobile app development, especially when dealing with user data. Laravel provides built-in authentication features that can be used to secure the API endpoints. This can be done using Laravel’s middleware to protect routes that require authentication.
Conclusion
Creating a Laravel API for mobile app development can provide developers with a powerful and scalable solution for building robust mobile applications. By leveraging Laravel’s features for scalability, security, performance, and flexibility, developers can create APIs that are well-suited for integration with mobile applications.
With the increasing demand for mobile app development, the need for efficient and reliable APIs has also grown. By following the steps outlined in this article, developers can create Laravel APIs that meet the requirements of modern mobile applications, ensuring a seamless and secure user experience.
FAQs
1. Can Laravel APIs be used for both iOS and Android app development?
Yes, Laravel APIs can be used for both iOS and Android app development. The API endpoints can be accessed by mobile applications developed for different platforms, making Laravel a versatile choice for mobile app development.
2. Are there any specific tools or packages recommended for creating Laravel APIs?
There are several tools and packages that can enhance the development of Laravel APIs, such as Laravel Passport for API authentication, Laravel Eloquent for database operations, and Laravel Fractal for transforming API responses. These can help streamline the process of creating and maintaining Laravel APIs for mobile app development.
3. How can I optimize my Laravel API for mobile app performance?
Optimizing a Laravel API for mobile app performance can be achieved by implementing caching mechanisms, optimizing database queries, and minifying and compressing API responses. Additionally, using tools for API performance monitoring and profiling can help identify and address performance bottlenecks.