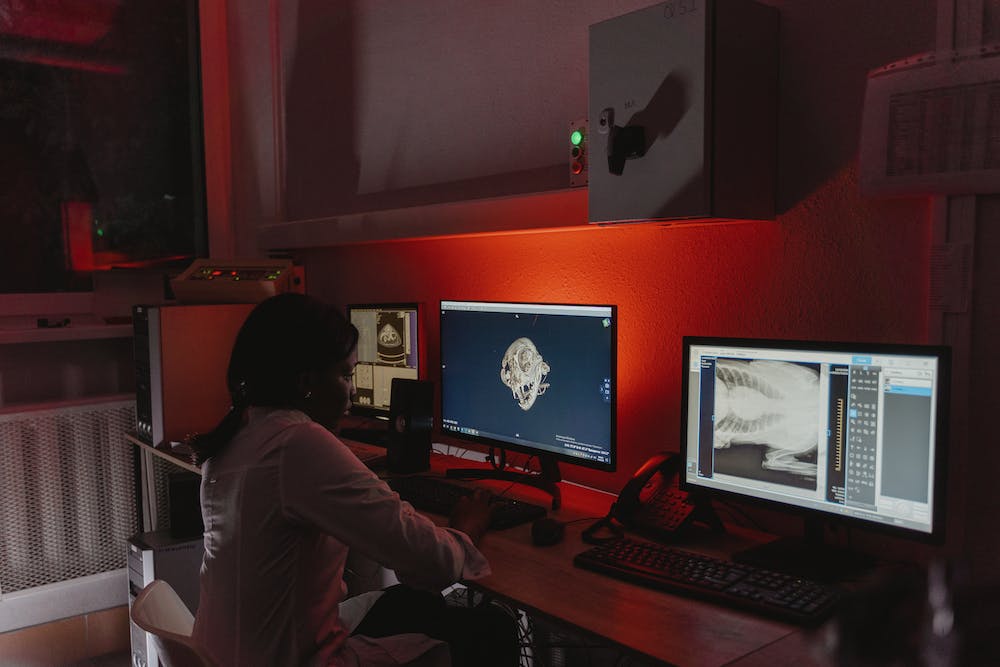
Python is widely regarded as one of the most popular programming languages in the world. Its simplicity, versatility, and robustness make IT an excellent choice for both beginners and experienced developers alike. So, if you’re preparing for a Python interview, IT‘s crucial to have a solid understanding of the language and be able to demonstrate your coding skills effectively.
In this article, we will explore some fundamental Python programs that interviewees must know to ace their coding interviews. These examples cover a range of topics, from basic syntax to algorithms and data structures.
The Basics: Syntax and Control Flow
Let’s start with some basic programs that showcase Python’s syntax and control flow.
Hello World
A classic tradition in programming, the “Hello, World!” program is often the first step in learning a new language. In Python, IT‘s as simple as:
print("Hello, World!")
Prime Numbers
Checking if a given number is prime or not is a common interview question:
def is_prime(num):
if num <= 1:
return False
for i in range(2, int(num ** 0.5) + 1):
if num % i == 0:
return False
return True
Data Structures
Understanding data structures is essential in programming. Here are a few examples:
Lists
Lists are a fundamental data structure in Python. Here’s how you can reverse a list:
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list)
Dictionaries
Dictionaries consist of key-value pairs. To check if a key exists in a dictionary, you can use:
my_dict = {"apple": 1, "banana": 2, "cherry": 3}
if "apple" in my_dict:
print("Key exists!")
Algorithms
Many interview questions test your algorithmic thinking abilities. Here’s an example:
Fibonacci Sequence
The Fibonacci sequence is a classic algorithmic problem.
def fibonacci(n):
if n <= 0:
return []
elif n == 1:
return [0]
elif n == 2:
return [0, 1]
else:
sequence = [0, 1]
while len(sequence) < n:
next_num = sequence[-1] + sequence[-2]
sequence.append(next_num)
return sequence
Conclusion
In this article, we have covered some essential Python programs that every interviewee must know. From basic syntax to data structures and algorithms, having a solid understanding of these concepts will help you tackle coding challenges with confidence during your interviews.
Remember, practicing these programs and understanding the underlying concepts is key to mastering Python. Keep coding, learning, and honing your skills to excel in your Python interview.
FAQs
Q: Why is Python a popular language for interviews?
A: Python’s popularity in interviews is mainly due to its simplicity, readability, and vast library support. Its clean syntax allows candidates to express their ideas concisely, making IT easier for interviewers to understand their thought process and problem-solving skills.
Q: Can I use references or online resources during my interview?
A: IT‘s generally not recommended to rely heavily on external resources during your interview. Interviewers assess your ability to solve problems independently and rely on your coding knowledge. However, IT‘s acceptable to ask clarifying questions or discuss ideas with the interviewer.
Q: How can I prepare for a Python coding interview?
A: Some tips for preparing for a Python coding interview include practicing coding challenges, brushing up on fundamental concepts, understanding data structures and algorithms, and reviewing common interview questions specific to Python. Additionally, mock interviews and coding competitions can boost your confidence and problem-solving abilities.