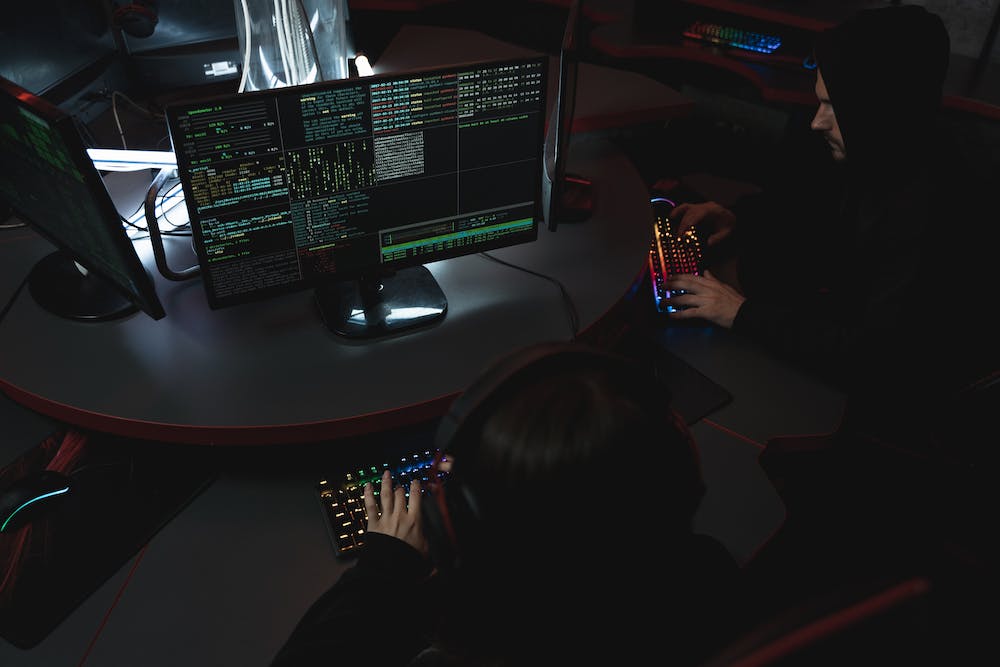
If you’re interested in learning Python programming and want to create a fun game, you’re in the right place! In this article, we’ll show you how to create a simple Python game using just a few lines of code. Even if you’re a beginner, you’ll be able to follow along and understand how the game works.
The Game: Rock, Paper, Scissors
The game we’ll be creating is the classic Rock, Paper, Scissors. In this game, the player chooses either rock, paper, or scissors, and the computer also makes a choice. The winner is determined based on the rules: rock beats scissors, scissors beats paper, and paper beats rock.
The Code
Here’s the simple Python code for the Rock, Paper, Scissors game:
“`python
import random
def get_user_choice():
user_choice = input(“Enter rock, paper, or scissors: “).lower()
return user_choice
def get_computer_choice():
options = [“rock”, “paper”, “scissors”]
computer_choice = random.choice(options)
return computer_choice
def determine_winner(user_choice, computer_choice):
if user_choice == computer_choice:
return “IT‘s a tie!”
elif (user_choice == “rock” and computer_choice == “scissors”) or (user_choice == “scissors” and computer_choice == “paper”) or (user_choice == “paper” and computer_choice == “rock”):
return “You win!”
else:
return “You lose!”
def main():
user_choice = get_user_choice()
computer_choice = get_computer_choice()
print(“You chose:”, user_choice)
print(“computer chose:”, computer_choice)
print(determine_winner(user_choice, computer_choice))
main()
“`
Simply copy and paste the above code into a Python IDE or text editor, and you’ll have a working Rock, Paper, Scissors game ready to go!
How IT Works
Let’s break down the code to understand how the game works:
- We start by importing the random module, which we’ll use to make the computer‘s choice random.
- We then define a function
get_user_choice
to get the user’s input for their choice of rock, paper, or scissors. - Next, we define a function
get_computer_choice
to randomly select the computer‘s choice from the options list. - We then define a function
determine_winner
to compare the user’s choice with the computer‘s choice and determine the winner based on the game rules. - Finally, in the
main
function, we call the other functions and print the user’s choice, computer‘s choice, and the outcome of the game.
Conclusion
Creating a fun Python game doesn’t have to be complicated. With just a few lines of code, you can build a simple yet entertaining game like Rock, Paper, Scissors. This is a great way to practice your Python skills and have fun at the same time. Feel free to modify the code and add your own features to make the game even more exciting!
FAQs
Q: I’m new to Python. Can I still follow along with this tutorial?
A: Absolutely! This tutorial is designed for beginners, and the code is simple and easy to understand. You’ll be able to create the game even if you’re just starting out with Python.
Q: Can I add more options to the game, like lizard and Spock?
A: Yes, you can definitely expand the game to include additional options. You’ll need to modify the code to account for the new rules of the game, but IT‘s a great way to challenge yourself and learn more about Python programming.
Q: How can I make the game more interactive with a graphical user interface?
A: Adding a graphical user interface (GUI) to the game is a great idea for enhancing the user experience. You can use libraries like Tkinter or Pygame to create a visual interface for the game, allowing users to click buttons to make their choices and see the results on the screen.