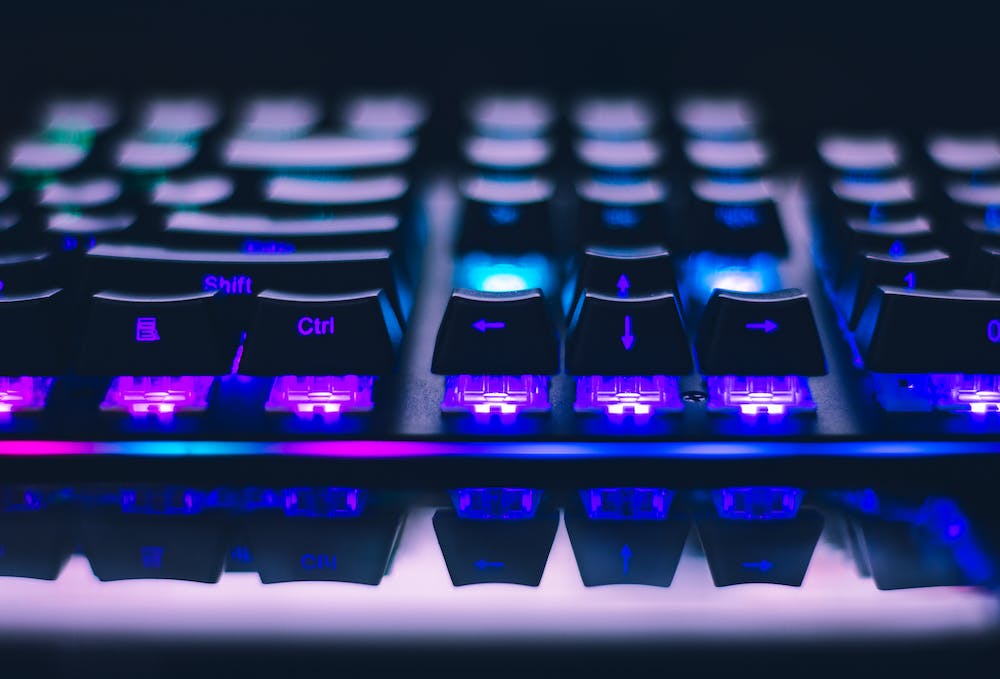
PHP is a popular programming language used for creating dynamic web pages. However, like any other programming language, PHP is prone to syntax errors which can be frustrating for developers. In this article, we will discuss some common syntax errors in PHP and how to fix them.
Missing Semicolon
One of the most common syntax errors in PHP is the missing semicolon at the end of a statement. For example:
<?php
$message = "Hello, World"
echo $message;
?>
In the above example, the missing semicolon at the end of the $message variable assignment will result in a syntax error. To fix this error, simply add a semicolon at the end of the statement:
<?php
$message = "Hello, World";
echo $message;
?>
Missing Parenthesis
Another common syntax error is the missing parenthesis in function calls. For example:
<?php
function greet() {
echo "Hello, World";
?>
In this example, the missing closing parenthesis at the end of the greet function will result in a syntax error. To fix this error, simply add the closing parenthesis:
<?php
function greet() {
echo "Hello, World";
}
?>
Undefined Variables
Using undefined variables in PHP will also result in syntax errors. For example:
<?php
echo $name;
?>
In this example, the $name variable is not defined, which will result in a syntax error. To fix this error, make sure to define the variable before using IT:
<?php
$name = "John";
echo $name;
?>
Missing Quotes
Forgetting to add quotes around strings can also lead to syntax errors. For example:
<?php
echo Hello, World!;
?>
In this example, the missing quotes around the string “Hello, World!” will result in a syntax error. To fix this error, simply add quotes around the string:
<?php
echo "Hello, World!";
?>
Conclusion
In conclusion, common syntax errors in PHP can be frustrating, but they are easily preventable and fixable. By paying attention to details such as semicolons, parentheses, variable definitions, and quotes, developers can avoid these errors and write clean, error-free PHP code.
FAQs
Q: Why is it important to fix syntax errors in PHP?
A: Fixing syntax errors in PHP is important because they can cause the code to break and not function as intended. It is essential to write clean and error-free code to ensure the proper functioning of the web application.
Q: Can syntax errors in PHP affect the performance of a Website?
A: Yes, syntax errors can affect the performance of a website as they can cause the code to break, leading to errors and malfunctions. This can result in a poor user experience and impact the overall performance of the website.
Q: Are there any tools available to help identify and fix syntax errors in PHP?
A: Yes, there are various IDEs (Integrated Development Environments) and code editors available that provide syntax highlighting and error checking for PHP code. Additionally, there are online tools and linters that can help identify and fix syntax errors in PHP.