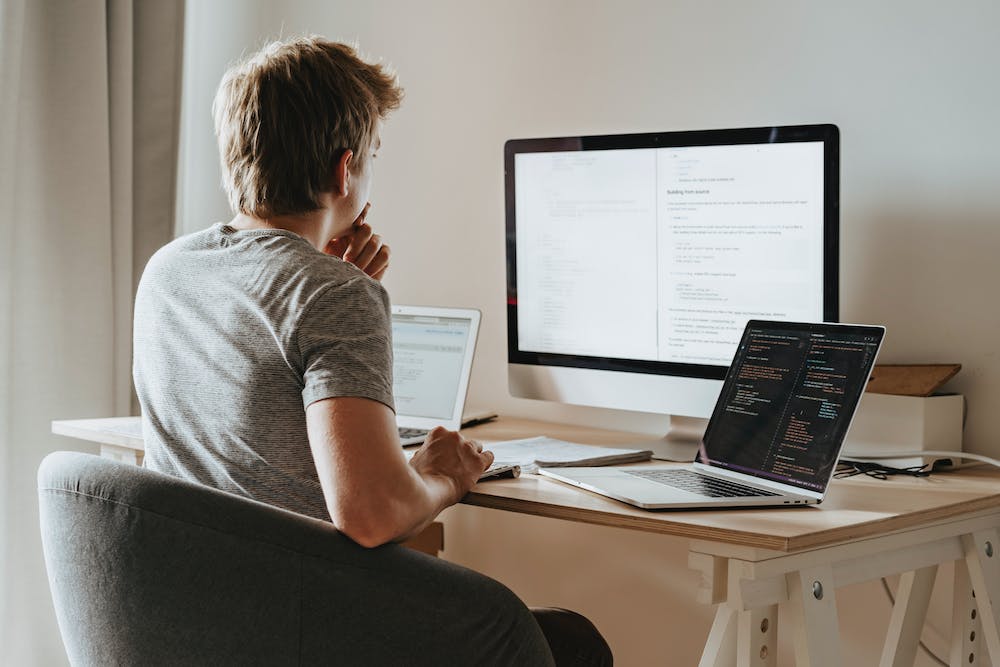
Python is a popular programming language known for its simplicity and readability. However, like any other programming language, Python is prone to coding mistakes. In this article, we will discuss some common Python coding mistakes and provide tips on how to avoid them.
1. Not Using Indentation Properly
One of the most common mistakes in Python is not using proper indentation. Python relies on indentation to define code blocks, so failing to indent code properly can lead to syntax errors. For example:
if x > 5:
print("x is greater than 5")
To avoid this mistake, always use consistent indentation (e.g. 4 spaces or a tab) for code blocks.
2. Forgetting to Import Modules
Python has a wide range of modules and libraries that provide additional functionality. Forgetting to import the necessary modules before using them is a common mistake. For example:
import math
print(math.sqrt(16))
To avoid this mistake, always make sure to import the required modules at the beginning of your script.
3. Using the Wrong Comparison Operators
Another common mistake is using the wrong comparison operators. In Python, the equality operator is ==
, while the assignment operator is =
. Using the wrong operator can lead to unexpected behavior in your code. For example:
x = 5
if x = 5:
print("x is 5")
To avoid this mistake, always pay close attention to the comparison operators you are using in your code.
4. Not Handling Exceptions
Failing to handle exceptions can lead to runtime errors and unexpected termination of your program. Always use try-except blocks to handle potential exceptions in your code. For example:
try:
x = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
By handling exceptions properly, you can prevent your program from crashing unexpectedly.
5. Using Poor Variable Names
Using unclear or ambiguous variable names can make your code difficult to understand and maintain. Always use descriptive and meaningful variable names to make your code more readable. For example:
age = 21
# Avoid using single letters or cryptic abbreviations
Using meaningful variable names can enhance the clarity and maintainability of your code.
6. Not Testing Your Code
One of the most crucial mistakes is not testing your code thoroughly. Testing helps to identify bugs and ensure that your code behaves as expected. Always write and run test cases to verify the functionality of your code.
7. Ignoring PEP 8 Guidelines
PEP 8 is the official style guide for Python code. Ignoring PEP 8 guidelines, such as using descriptive function and variable names, maintaining consistent indentation, and following naming conventions, can lead to messy and hard-to-read code. Always adhere to PEP 8 guidelines to write clean and maintainable Python code.
8. Not Using Comments
Comments are crucial for explaining the functionality of your code. Not using comments can make IT challenging for others (or even yourself) to understand the purpose of your code. Always use comments to explain complex logic, assumptions, or any unusual code.
9. Overusing Global Variables
Overusing global variables can lead to unintended side effects and make your code difficult to test and maintain. Always try to limit the use of global variables and use local variables whenever possible.
10. Using Inefficient Data Structures
Using inefficient data structures, such as lists when sets or dictionaries would be more appropriate, can impact the performance of your code. Always choose the appropriate data structure for your specific use case to optimize the performance of your code.
Conclusion
Avoiding common coding mistakes is essential for writing clean, efficient, and maintainable Python code. By paying attention to proper indentation, module imports, comparison operators, exception handling, variable names, testing, style guidelines, comments, variable scope, and data structures, you can significantly improve the quality of your Python code.
FAQs
Q: Why is proper indentation important in Python?
A: Proper indentation is crucial in Python as it defines the structure and logic of your code. Incorrect indentation can lead to syntax errors and result in unexpected behavior.
Q: How can I test my Python code?
A: You can test your Python code by writing and running test cases using frameworks such as unittest or pytest.
Q: What are some common data structures used in Python?
A: Some common data structures in Python include lists, sets, dictionaries, tuples, and arrays.