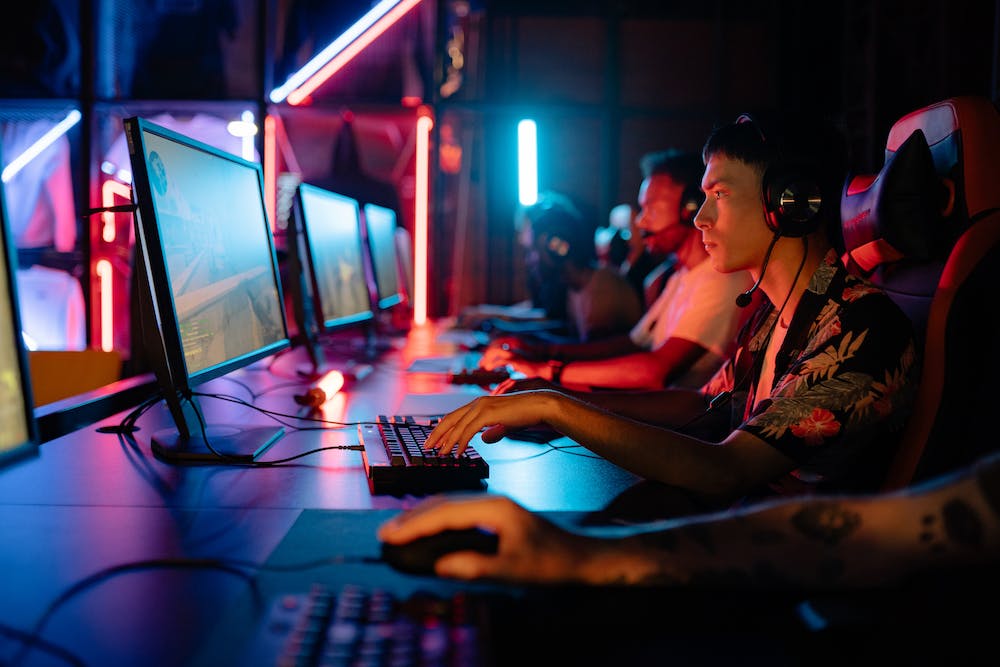
Laravel is a popular PHP framework known for its simplicity and efficiency in building web applications. One of the key features of web applications is user authentication, which allows users to securely access and interact with the application. In this article, we will walk through the process of building user authentication in Laravel, step-by-step.
Step 1: Setting Up Laravel
The first step in building user authentication in Laravel is to set up a new Laravel project. If you haven’t installed Laravel, you can do so using Composer by running the following command in your terminal:
composer create-project --prefer-dist laravel/laravel project-name
Once your project is set up, navigate to the project directory and start the development server by running the following command:
php artisan serve
Step 2: Database Configuration
Next, you’ll need to configure your database connection in the .env
file. Open the .env
file and update the following variables with your database credentials:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=database_username
DB_PASSWORD=database_password
Save the changes and run the following command to create the migration for the users table:
php artisan make:migration create_users_table --create=users
Open the generated migration file and define the schema for the users table:
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
Run the migration to create the users table in your database:
php artisan migrate
Step 3: User Model
Next, you’ll need to create a User model which represents the users table in your application. Run the following command to generate the User model:
php artisan make:model User
Once the model is generated, you can define the fillable attributes and any relationships in the model file. By default, the User model’s fillable property will include the name, email, password, and remember_token attributes.
Step 4: Authentication Scaffolding
Laravel provides an authentication scaffolding that includes login, registration, and password reset functionality out of the box. Run the following command to generate the authentication scaffolding:
php artisan make:auth
This command will generate the necessary routes, controllers, and views for user authentication. You can customize the views and controllers as needed to fit the design and functionality of your application.
Step 5: Protecting Routes
Once the authentication scaffolding is generated, you can protect routes that require authentication by using the auth
middleware. For example, to protect a route in your web.php
file, use the following syntax:
Route::get('/dashboard', 'DashboardController@index')->middleware('auth');
This will ensure that only authenticated users can access the ‘/dashboard’ route.
Step 6: Conclusion
Congratulations! You’ve successfully built user authentication in your Laravel application. By following these steps, you’ve set up user registration, login, and password reset functionality, as well as protected routes that require authentication. With user authentication in place, you can now securely manage user access and interactions within your application.
FAQs
Q: What is Laravel?
A: Laravel is a PHP framework known for its elegant syntax and powerful features that make web development a breeze.
Q: Can I customize the authentication views in Laravel?
A: Yes, you can customize the authentication views by modifying the generated blade files in the resources/views/auth
directory.
References
1. Laravel Documentation – https://laravel.com/docs/8.x/authentication
2. “Laravel 8 From Scratch” by Laracasts – https://laracasts.com/series/laravel-8-from-scratch