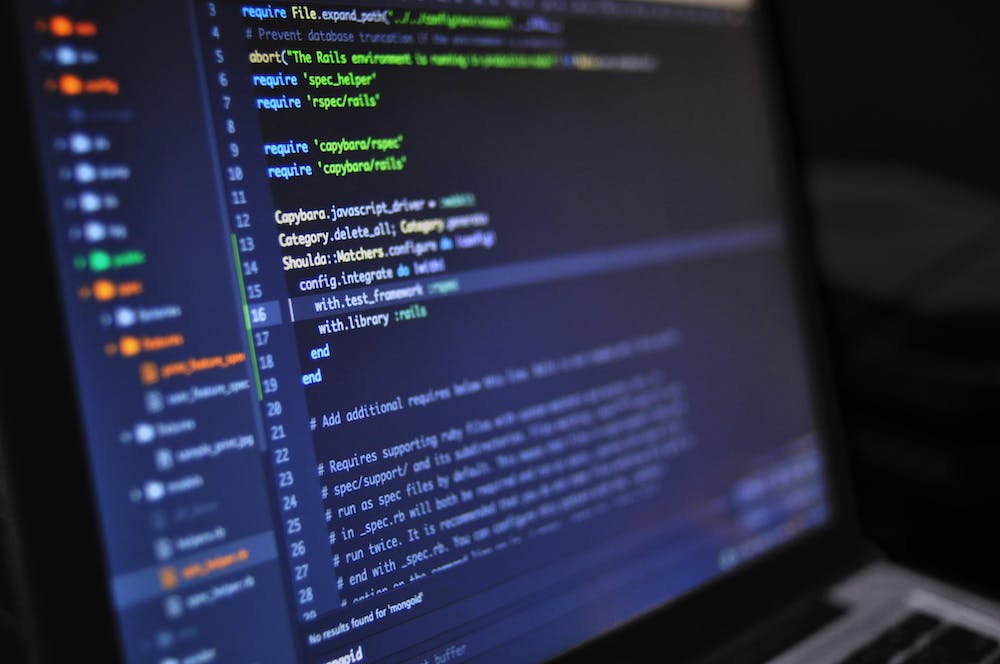
Node.js is a popular platform for building scalable server-side applications. IT provides a rich set of features for creating web APIs, making it an excellent choice for building RESTful APIs. In this tutorial, we will walk through the process of building a RESTful API with Node.js, step by step.
Step 1: Setting Up Your Development Environment
The first step in building a RESTful API with Node.js is to set up your development environment. You will need to have Node.js and npm (Node Package Manager) installed on your machine. You can download and install Node.js from the official Website (https://nodejs.org/). Once Node.js is installed, npm will also be available for use.
Next, you will need a code editor to write your Node.js code. Some popular code editors for Node.js development include Visual Studio Code, Sublime Text, and Atom. Choose the one that best suits your preferences and install it on your machine.
Step 2: Creating a New Node.js Project
Once your development environment is set up, you can create a new Node.js project for building your RESTful API. Open a terminal or command prompt and navigate to the directory where you want to create your project. Then, run the following command to initialize a new Node.js project:
npm init -y
This command will create a new package.json file in your project directory, which will store information about your project and its dependencies.
Step 3: Installing Required Dependencies
Next, you will need to install the necessary dependencies for building a RESTful API with Node.js. The two main dependencies you will need are Express and Body-Parser. Express is a web application framework for Node.js, while Body-Parser is a middleware for parsing incoming request bodies.
To install these dependencies, run the following commands in your terminal:
npm install express body-parser
Step 4: Creating Your First Endpoint
With your project set up and dependencies installed, you can now start building your RESTful API. Create a new file in your project directory called server.js, and write the following code to create your first API endpoint:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.get('/api/hello', (req, res) => {
res.json({ message: 'Hello, World!' });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this code, we are using the Express framework to create a new web server. We then define a GET endpoint at /api/hello, which responds with a JSON message. Finally, we start the server and listen for incoming requests on port 3000.
Step 5: Testing Your API Endpoint
With your API endpoint created, it’s time to test it to ensure that it’s working as expected. Open a web browser or use a tool like Postman to send a GET request to http://localhost:3000/api/hello. You should see a JSON response with the message “Hello, World!”
Step 6: Adding CRUD Operations
So far, we’ve created a simple GET endpoint. To build a complete RESTful API, you will need to add support for CRUD (Create, Read, Update, Delete) operations. Here’s an example of how to add support for creating a new resource:
let data = [];
app.post('/api/data', (req, res) => {
const newData = req.body;
data.push(newData);
res.json(newData);
});
In this code, we are defining a new POST endpoint at /api/data, which accepts a JSON object in the request body and adds it to the data array. The endpoint then responds with the added data.
Step 7: Adding Database Integration
In a real-world scenario, you will likely want to store your data in a database rather than in-memory. Node.js provides several libraries for working with databases, such as MongoDB, MySQL, and PostgreSQL. You can integrate your API with a database using one of these libraries to persist your data.
Step 8: Securing Your API
Security is an essential aspect of building web APIs. You can secure your RESTful API by implementing authentication and authorization mechanisms. There are many ways to do this, including using JSON Web Tokens (JWT), OAuth, or integrating with third-party authentication providers.
Step 9: Documenting Your API
Documenting your API is crucial for developers who want to consume it. Tools like Swagger and Postman provide robust solutions for documenting and testing APIs. By documenting your API, you make it easier for others to understand and use your endpoints.
Step 10: Deploying Your API
Once your RESTful API is complete, you can deploy it to a production environment for public consumption. You can host your API on cloud platforms like AWS, Azure, or Google Cloud, or use PaaS (Platform as a Service) solutions like Heroku or backlink works.
Conclusion
Building RESTful APIs with Node.js is a straightforward and powerful process. By following the steps outlined in this tutorial, you can create a robust and scalable API for your applications. Whether you are developing a small personal project or a large enterprise system, Node.js provides the tools and capabilities to build high-quality web APIs.
FAQs
Q: What is a RESTful API?
A: A RESTful API is an architectural style for designing networked applications. It stands for Representational State Transfer and is based on the principles of using standard HTTP methods for communication and stateless communication between client and server.
Q: Why use Node.js for building RESTful APIs?
A: Node.js is well-suited for building RESTful APIs due to its non-blocking I/O model, event-driven architecture, and rich ecosystem of modules. It allows for fast and scalable development of web APIs and is widely used in the industry for this purpose.
Q: Can I use other frameworks besides Express for building RESTful APIs with Node.js?
A: Yes, there are other frameworks like Hapi, Koa, and Sails which can be used to build RESTful APIs with Node.js. However, Express is the most popular and widely used framework for this purpose due to its simplicity and flexibility.
Q: How can I handle errors in my RESTful API?
A: You can handle errors in your API by using try-catch blocks, using middleware for error handling, and returning appropriate HTTP status codes with error messages in your responses.
Q: What are the best practices for designing and developing RESTful APIs?
A: Some best practices for designing and developing RESTful APIs include using meaningful resource URIs, following the principles of REST, providing clear and consistent error messages, and using proper HTTP status codes for responses.