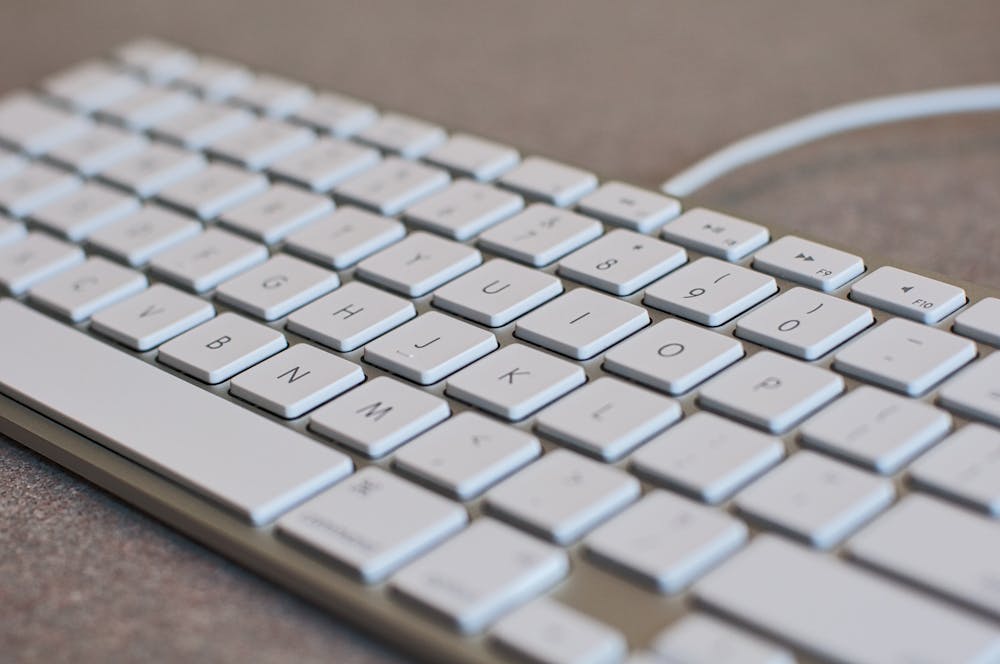
In today’s fast-paced world, real-time web applications are becoming increasingly important. Users expect instant updates and interactive experiences, and building such applications requires a solid understanding of both backend and frontend technologies. Laravel and Vue.js are two popular tools that can be used together to create real-time web applications efficiently and effectively.
Laravel: A Powerful Backend Framework
Laravel is a PHP framework known for its elegant syntax and powerful features. IT provides a solid foundation for building web applications with features such as routing, middleware, and Eloquent ORM for working with databases. Laravel also includes support for real-time applications through tools like Laravel Echo and Pusher, making it an excellent choice for building real-time web applications.
Vue.js: A Dynamic Frontend Library
Vue.js is a progressive JavaScript framework that is known for its simplicity and flexibility. It allows developers to build user interfaces and single-page applications with ease. Vue.js also has a reactivity system that makes it easy to handle real-time updates, making it a perfect fit for building real-time web applications.
Integrating Laravel and Vue.js for Real-Time Applications
When building a real-time web application, it’s essential to have a seamless integration between the backend and frontend. Laravel and Vue.js work extremely well together, and using them in tandem can result in a powerful and efficient real-time application.
One way to integrate Laravel and Vue.js is by using Laravel’s API routes to create endpoints for data retrieval and manipulation. Vue.js can then consume these endpoints to display real-time updates to the user. This allows for a clean separation of concerns, with Laravel handling the backend logic and Vue.js handling the frontend presentation.
Setting up Laravel for Real-Time
To get started with real-time web applications in Laravel, you can use Laravel Echo and Pusher. Laravel Echo is a JavaScript library that makes it easy to subscribe to channels and listen for events, while Pusher is a hosted service that provides a real-time data infrastructure.
First, you need to install the Laravel Echo and Pusher libraries:
npm install --save laravel-echo pusher-js
Next, you can configure your Laravel application to use Pusher as the broadcast driver in the config/broadcasting.php
file:
'pusher' => [
'driver' => 'pusher',
'key' => env('PUSHER_APP_KEY'),
'secret' => env('PUSHER_APP_SECRET'),
'app_id' => env('PUSHER_APP_ID'),
'options' => [
'cluster' => env('PUSHER_APP_CLUSTER'),
'encrypted' => true,
],
],
Once the configuration is set up, you can use Laravel’s event broadcasting feature to broadcast events to your frontend application. For example, you can create an event class and broadcast it when a certain action occurs:
class OrderShipped implements ShouldBroadcast
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public $order;
public function __construct($order)
{
$this->order = $order;
}
public function broadcastOn()
{
return new Channel('orders');
}
}
On the Vue.js side, you can set up a listener to receive the broadcasted event and update the user interface accordingly:
Echo.channel('orders')
.listen('OrderShipped', (e) => {
console.log(e.order);
// Update the UI with the new order data
});
Using Vue.js for Real-Time Updates
Vue.js makes it easy to handle real-time updates in your frontend application. By using the reactivity system, Vue.js can automatically update the UI when the underlying data changes. This makes it straightforward to display real-time updates to the user without having to manually manipulate the DOM.
For example, you can use Vue.js to create a real-time chat application where new messages are displayed instantly as they are sent. Vue.js can handle the reactivity of the message data, and new messages can be added to the UI without needing to refresh the entire page.
Conclusion
Building real-time web applications with Laravel and Vue.js is a powerful combination that allows for efficient and effective development. By using Laravel’s event broadcasting and Vue.js’s reactivity system, you can create seamless real-time experiences for your users. With the right tools and techniques, real-time web applications can be built with ease, providing users with the instant updates and interactive experiences they expect.
FAQs
Q: Can I use other frontend frameworks with Laravel for real-time web applications?
A: Yes, you can use other frontend frameworks such as React or Angular with Laravel for real-time web applications. Laravel’s API routes can be consumed by any frontend framework to create real-time experiences.
Q: Is it necessary to use Pusher for real-time applications with Laravel?
A: No, Pusher is not necessary, but it is a convenient and reliable solution for real-time applications. You can use other solutions or even build your own real-time infrastructure if you prefer.