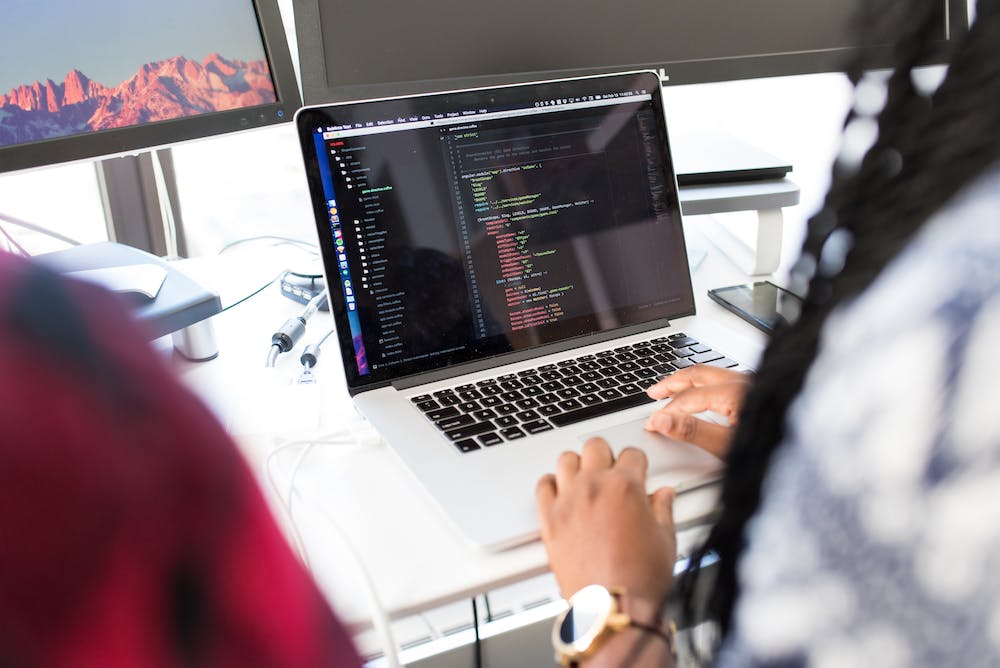
Creating Excel reports is a common task for many businesses and organizations. IT allows for easy data analysis, visualization, and sharing of important information. In this article, we will discuss how to use PHPExcel, a powerful PHP library, to build Excel reports and enhance your reporting capabilities.
What is PHPExcel?
PHPExcel is a set of PHP classes that allows for the reading and writing of Excel files. It provides a wide range of features and functionalities for creating and manipulating Excel spreadsheets, making it a popular choice for developers looking to generate dynamic Excel reports from their PHP applications.
Setting Up PHPExcel
To get started with PHPExcel, you’ll need to download the library from its official Website or use a package manager such as Composer to install it. Once you have the library set up in your project, you can start using its classes and methods to build Excel reports.
Creating a Basic Excel Report
Let’s walk through a simple example of how to create a basic Excel report using PHPExcel. In this example, we’ll create a spreadsheet with some sample data and save it to a file.
“`php
require ‘vendor/autoload.php’;
// Create a new PHPExcel object
$objPHPExcel = new \PhpOffice\PhpSpreadsheet\Spreadsheet();
// Set the title of the spreadsheet
$objPHPExcel->getProperties()->setTitle(‘Sample Report’);
// Add some data to the spreadsheet
$objPHPExcel->setActiveSheetIndex(0)
->setCellValue(‘A1’, ‘Name’)
->setCellValue(‘B1’, ‘Age’)
->setCellValue(‘A2’, ‘John Doe’)
->setCellValue(‘B2’, 30);
// Save the spreadsheet to a file
$writer = \PhpOffice\PhpSpreadsheet\IOFactory::createWriter($objPHPExcel, ‘Xlsx’);
$writer->save(‘sample_report.xlsx’);
?>
“`
In this example, we create a new PHPExcel object, set the title of the spreadsheet, add some data to it, and then save it to a file in XLSX format. This is a very basic example, but it gives you an idea of how easy it is to start creating Excel reports with PHPExcel.
Enhancing Excel Reports with PHPExcel
While the basic example above demonstrates how to create a simple Excel report, PHPExcel provides a wide range of features and functionalities for enhancing your reports. Some of the key capabilities of PHPExcel include:
- Formatting cells and ranges
- Creating charts and graphs
- Adding formulas and functions
- Working with multiple worksheets
- Reading existing Excel files and manipulating their contents
By leveraging these features, you can build professional-looking and dynamic Excel reports that meet your specific requirements and provide valuable insights for your stakeholders.
Best Practices for Building Excel Reports with PHPExcel
When working with PHPExcel to build Excel reports, it’s important to follow best practices to ensure the quality and performance of your reports. Some best practices to consider include:
- Organize your code and logic to generate reports in a modular and reusable manner
- Optimize the performance of your reports by minimizing unnecessary calculations and file operations
- Handle errors and exceptions gracefully to provide a smooth user experience
- Keep your reports secure by validating input data and preventing potential security vulnerabilities
By adhering to these best practices, you can ensure that your Excel reports are reliable, efficient, and maintainable in the long run.
Conclusion
Building Excel reports with PHPExcel can greatly enhance your reporting capabilities and provide valuable insights for your stakeholders. By leveraging the features and functionalities of PHPExcel, you can create dynamic and professional-looking Excel reports that meet your specific requirements.
FAQs
Q: Is PHPExcel still actively maintained?
A: As of writing, PHPExcel has been deprecated in favor of PhpSpreadsheet, which is a next-generation version of the library. PhpSpreadsheet provides all the features and functionalities of PHPExcel while addressing some of its limitations and introducing new capabilities.
Q: Can I use PHPExcel for commercial projects?
A: Yes, PHPExcel is distributed under the LGPL license, which allows for its use in both open-source and commercial projects. However, it’s important to review the license terms and ensure compliance with any third-party dependencies that PHPExcel may have.
Q: Are there any alternatives to PHPExcel for building Excel reports?
A: Yes, there are other PHP libraries and tools available for creating and manipulating Excel files, such as PHPOffice/PhpSpreadsheet, Spout, and ExcelBundle for Symfony. It’s recommended to evaluate the features, performance, and community support of each option to choose the best fit for your specific requirements.