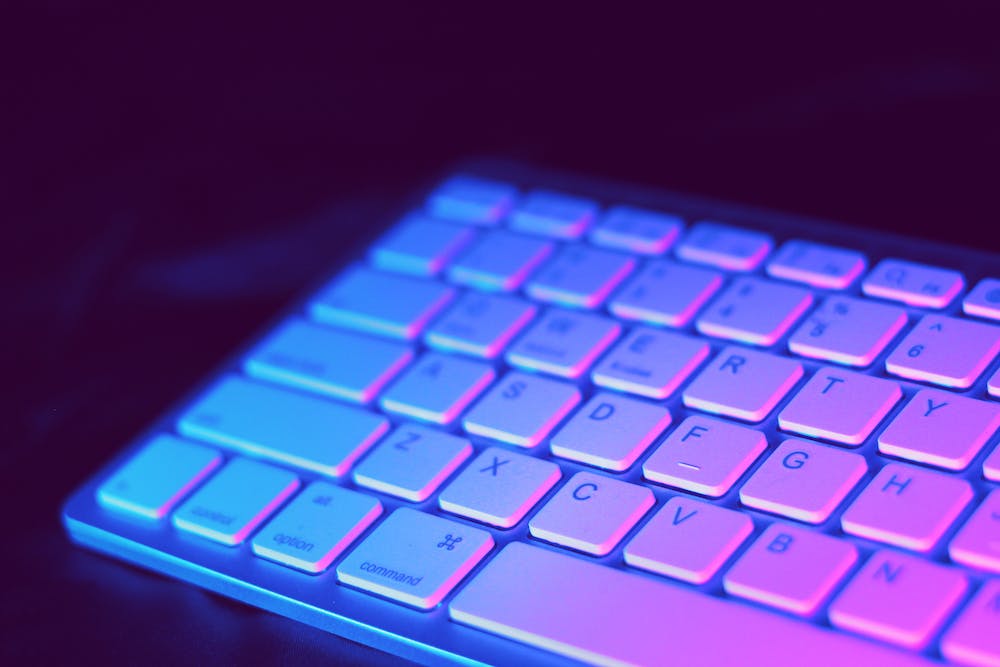
PHP is a powerful scripting language that is commonly used to build dynamic websites. When combined with HTML, PHP can create dynamic web pages that can interact with databases, handle form submissions, and generate dynamic content. In this article, we will explore the process of building dynamic websites with PHP and HTML, and how this combination can be used to create dynamic and interactive web applications.
Getting Started with PHP and HTML
Before we begin building dynamic websites with PHP and HTML, IT‘s important to have a basic understanding of both languages. HTML is the standard markup language used to create web pages, while PHP is a server-side scripting language that can be embedded directly into HTML code. This means that PHP code is executed on the server before the resulting HTML page is sent to the user’s browser.
Setting Up a Development Environment
To get started with building dynamic websites using PHP and HTML, you will need a local development environment. You can use a software stack such as XAMPP, MAMP, or WAMP, which provides a local server, PHP interpreter, and database server. Once you have your development environment set up, you can start to create dynamic web pages using PHP and HTML.
Using PHP to Generate Dynamic Content
One of the primary uses of PHP in web development is to generate dynamic content. This can include pulling data from a database, processing form submissions, or creating dynamic web pages based on user input. Let’s take a look at how we can use PHP to generate dynamic content in a web page.
Connecting to a Database
PHP can be used to connect to a database, such as MySQL, and retrieve data to be displayed on a web page. For example, you can use PHP to execute SQL queries and then use the results to populate an HTML table with dynamic data. Here’s an example of how you can connect to a MySQL database using PHP:
“`php
$servername = “localhost”;
$username = “username”;
$password = “password”;
$dbname = “myDB”;
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection
if ($conn->connect_error) {
die(“Connection failed: ” . $conn->connect_error);
}
echo “Connected successfully”;
?>
“`
Handling Form Submissions
Another common use of PHP in web development is handling form submissions. When a user submits a form on a web page, the form data is sent to the server, where it can be processed using PHP. For example, you can use PHP to validate form input, sanitize data, and store the form data in a database. Here’s an example of how you can handle a form submission using PHP:
“`php
if ($_SERVER[“REQUEST_METHOD”] == “POST”) {
// Process form data
$name = $_POST[“name”];
$email = $_POST[“email”];
// Validate and sanitize form data
// Store form data in the database
}
?>
“`
Creating Dynamic Web Pages with PHP and HTML
PHP can be used to create dynamic web pages by embedding PHP code directly into HTML. This allows you to generate dynamic content, perform conditional logic, and iterate over data to create dynamic web pages. Let’s take a look at how we can create dynamic web pages using PHP and HTML.
Embedding PHP in HTML
To embed PHP code in an HTML page, you simply need to use the tags to enclose your PHP code. For example, you can use PHP to display dynamic content, such as database records, within an HTML table:
“`html
Name |
---|
“`
Using Conditional Logic
PHP can be used to perform conditional logic within an HTML page. For example, you can use PHP to display different content based on user input, database queries, or other conditions. Here’s an example of how you can use PHP to create conditional logic in an HTML page:
“`html
if ($userLoggedIn) {
echo “Welcome, $username!”;
} else {
echo “Please log in to access this content.”;
}
?>
“`
Enhancing User Interaction with PHP and HTML
In addition to generating dynamic content and creating dynamic web pages, PHP and HTML can be used to enhance user interaction on a Website. This can include creating user accounts, managing sessions, and handling user input to create interactive web applications.
Creating User Accounts
PHP can be used to create user accounts and manage user authentication on a website. You can use PHP to handle user registration, login, and password management to create a secure and interactive user experience. Here’s an example of how you can use PHP to create a user registration form:
“`html
“`
Managing Sessions
PHP can also be used to manage user sessions and track user activity on a website. You can use PHP to create session variables, track user login status, and personalize content based on user preferences. Here’s an example of how you can use PHP to manage user sessions:
“`php
session_start();
if (isset($_SESSION[“username”])) {
echo “Welcome back, ” . $_SESSION[“username”] . “!”;
} else {
echo “Please log in to access this content.”;
}
?>
“`
Conclusion
Building dynamic websites with PHP and HTML allows web developers to create interactive and personalized web applications. By leveraging the power of PHP to generate dynamic content, create dynamic web pages, and enhance user interaction, developers can create engaging and interactive websites for their users. With the right skills and knowledge, PHP and HTML can be used to create dynamic and functional web applications that meet the needs of today’s web users.
FAQs
What is PHP and how does it work with HTML?
PHP is a server-side scripting language that can be embedded directly into HTML code. This allows PHP to generate dynamic content, perform conditional logic, and interact with databases to create dynamic web pages.
Can I use PHP and HTML to create user accounts and manage sessions?
Yes, PHP can be used to create user accounts, manage user authentication, and track user sessions on a website. This allows you to create interactive and personalized web applications for your users.
Are there any specific tools or software required to start building dynamic websites with PHP and HTML?
You will need a local development environment to get started with building dynamic websites using PHP and HTML. Software stacks such as XAMPP, MAMP, or WAMP can provide a local server, PHP interpreter, and database server for your development needs.