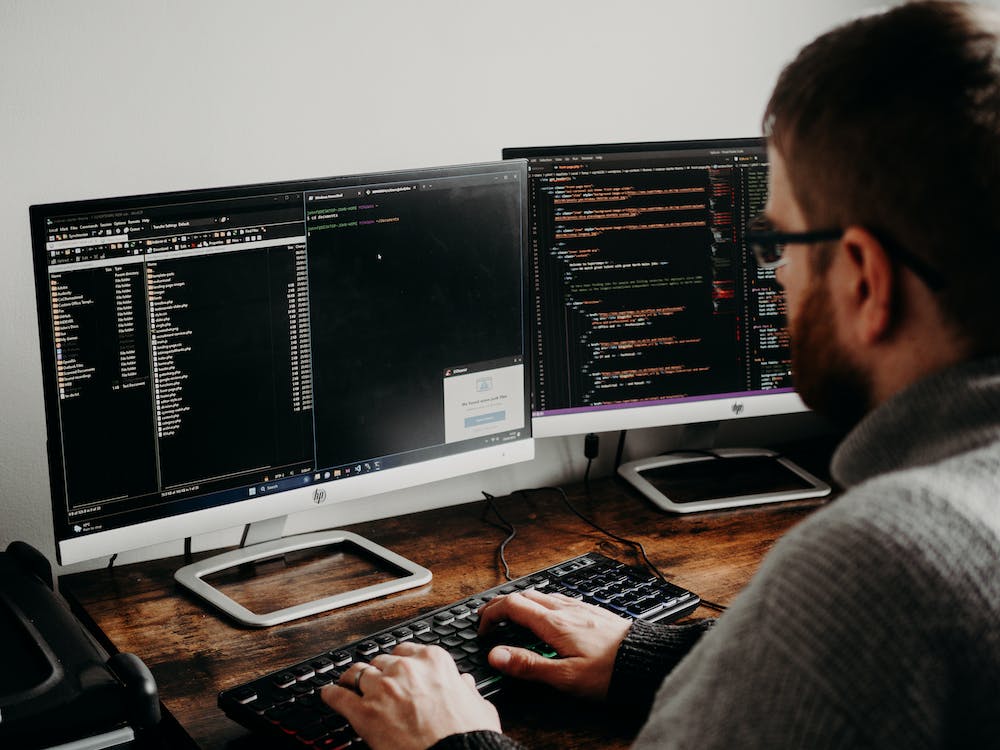
When IT comes to building modern web applications, developers are constantly looking for ways to create dynamic and responsive user interfaces. One technology that has gained significant popularity for building such UIs is Java React. In this article, we will explore the fundamentals of building dynamic UIs with Java React, including its key concepts, best practices, and examples.
Understanding Java React
Java React is a powerful front-end library for building user interfaces. It allows developers to create interactive UIs that can quickly respond to user actions and data changes. Java React accomplishes this through its efficient rendering and component-based architecture.
At the core of Java React is its virtual DOM (Document Object Model), which is a lightweight representation of the actual DOM. When a component’s state or props change, Java React efficiently updates the virtual DOM and then reconciles it with the actual DOM to reflect these changes. This process ensures that only the necessary components are re-rendered, making UI updates fast and efficient.
Building Dynamic UIs with Java React
When building dynamic UIs with Java React, developers follow a component-based approach. Each UI element is encapsulated within a component, and these components can be composed together to form complex user interfaces. This modular structure not only makes the codebase more organized and maintainable but also enables reusability and extensibility.
One of the key concepts in Java React is state management. Components can have their own state, which determines how they render and behave. When the state of a component changes, Java React automatically re-renders the component to reflect these changes. This makes it easy to create UIs that respond to user interactions and data updates.
Another important concept in building dynamic UIs with Java React is handling user input. Java React provides a simple and consistent API for capturing user input and updating the component’s state accordingly. This allows developers to create interactive forms, controls, and other UI elements that respond to user actions in real-time.
Best Practices for Building Dynamic UIs with Java React
When building dynamic UIs with Java React, it’s important to follow best practices to ensure a scalable, maintainable, and performant codebase. Here are some best practices to consider:
- Component Reusability: Break down the UI into reusable components to promote code reusability and maintainability.
- State Management: Use stateful components judiciously and consider using state management libraries like Redux for managing complex application state.
- Immutability: Follow the principle of immutability to prevent unexpected side effects when updating component state.
- Performance Optimization: Optimize rendering performance by minimizing unnecessary re-renders and leveraging techniques like memoization and virtualization.
Example: Building a Dynamic Todo List App with Java React
Let’s walk through a simple example of building a dynamic todo list application with Java React. We’ll create a TodoItem component to represent individual todo items and a TodoList component to display a list of todo items.
const TodoItem = ({ text, completed, onToggle }) => {
return (
{text}
);
};
const TodoList = ({ todos, onTodoToggle }) => {
return (
{todos.map((todo) => (
text={todo.text}
completed={todo.completed}
onToggle={() => onTodoToggle(todo.id)}
/>
))}
);
};
In this example, the TodoItem component represents an individual todo item with a checkbox to mark it as completed. The TodoList component receives a list of todo items and renders them using the TodoItem component. When a todo item is toggled, the onTodoToggle function is called to update its completion status.
Conclusion
Building dynamic UIs with Java React offers a powerful and efficient way to create modern web applications. By following best practices and leveraging Java React’s component-based architecture, state management, and performance optimizations, developers can build responsive and interactive user interfaces that provide a seamless user experience.
FAQs
Q: What is Java React?
A: Java React is a front-end library for building user interfaces in Java. It uses a component-based architecture and efficient rendering to create dynamic and responsive UIs.
Q: Is Java React similar to ReactJs?
A: While Java React and ReactJS share similar concepts and APIs, they are separate technologies developed for different ecosystems. Java React is specifically designed for building user interfaces in Java applications.
Q: Can I use Java React with other front-end technologies?
A: Yes, Java React can be integrated with other front-end technologies and libraries to create a comprehensive and feature-rich UI for web applications.
Q: How can I optimize the performance of dynamic UIs built with Java React?
A: To optimize performance, developers can minimize unnecessary re-renders, leverage memoization and virtualization techniques, and use state management libraries like Redux to manage complex application state.
Q: Are there any resources for learning more about Java React?
A: Yes, there are plenty of tutorials, documentation, and community resources available for learning Java React, including official documentation, online courses, and developer forums.