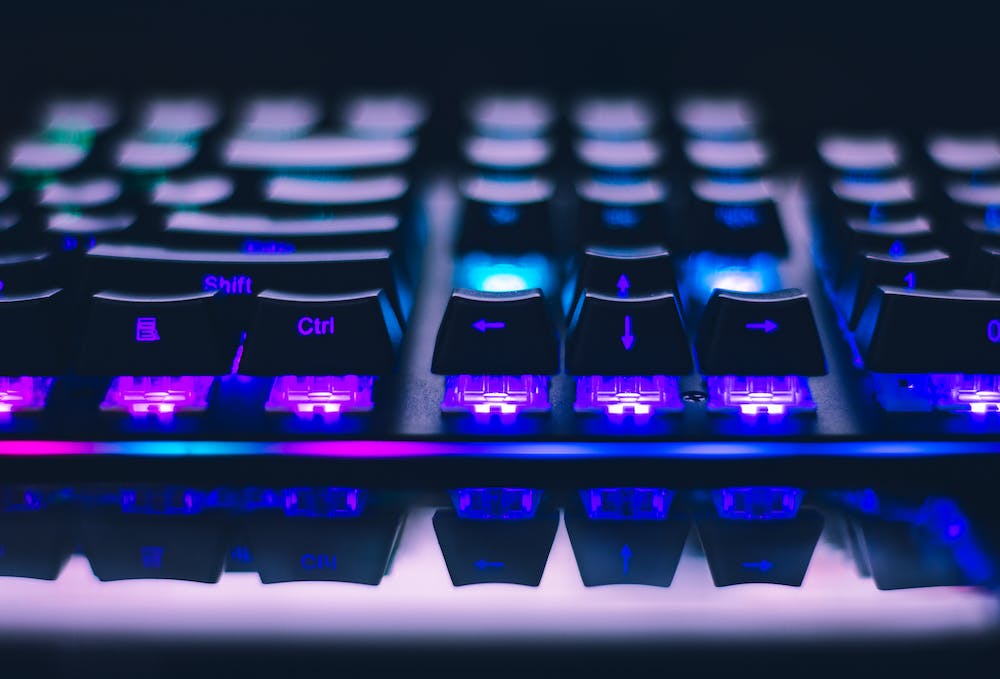
Laravel is a powerful PHP framework known for its elegant syntax and expressive code. IT provides various built-in features that make it easier for developers to create web applications with complex functionalities. One common requirement in web applications is to implement custom filter functionality to allow users to search and filter data according to their specific criteria. In this article, we will explore how to build custom filter functionality in Laravel applications to enhance the user experience and simplify data retrieval.
Understanding the Requirements
Before we start building custom filter functionality, it’s important to understand the requirements of the application. What are the data elements that need to be filtered? What are the filter criteria? What type of data needs to be displayed to the user? These questions will help us determine the structure and functionality of the custom filter.
Setting up the Laravel Project
If you don’t have a Laravel project set up, you can create a new project using the following command:
composer create-project --prefer-dist laravel/laravel custom-filter-app
Once the project is set up, we can proceed to define the data models and routes for the custom filter functionality.
Defining the Data Models
For the purpose of this article, let’s assume we have a “Products” table in our database that contains information about different products. We can define a “Product” model in Laravel to represent the data structure:
php artisan make:model Product
This will create a Product.php file in the “app\Models” directory, which we can use to define the properties and relationships of the product data.
Creating Filter Routes
We need to define routes that will handle the filter functionality. Let’s create a new route in the “web.php” file:
Route::get('/products', 'ProductController@index');
This route will point to the “index” method of the ProductController, which will be responsible for displaying the products and handling the filter requests.
Building the Filter Functionality
Now that we have set up the basic structure of our Laravel project, we can start building the custom filter functionality. The filter functionality can be implemented in the “index” method of the ProductController. We can start by fetching all the products from the database:
“`php
public function index(Request $request)
{
$products = Product::all();
// Implement filter logic here
return view(‘products.index’, compact(‘products’));
}
“`
Next, we need to implement the logic to apply filters based on the user’s input. We can use the “Request” object to access the filter criteria submitted by the user:
“`php
public function index(Request $request)
{
$products = Product::query();
if ($request->has(‘name’)) {
$products->where(‘name’, ‘like’, ‘%’ . $request->input(‘name’) . ‘%’);
}
if ($request->has(‘price’)) {
$products->where(‘price’, ‘>=’, $request->input(‘price’));
}
// More filter criteria can be added here
$products = $products->get();
return view(‘products.index’, compact(‘products’));
}
“`
In this example, we are checking if the request contains a “name” parameter and filtering the products based on the name criteria. Similarly, we are applying a filter for the “price” parameter. You can add more filter criteria based on the requirements of your application.
Displaying the Filtered Data
Once the filter criteria are applied, we need to display the filtered data to the user. We can do this by passing the filtered products to the view and rendering them accordingly:
“`php
// In the ProductController
return view(‘products.index’, compact(‘products’));
// In the “products.index” view
@foreach ($products as $product)
{{ $product->name }}
Price: ${{ $product->price }}
@endforeach
“`
This code snippet demonstrates how we can loop through the filtered products and display their information to the user. You can customize the view according to your application’s design and requirements.
Conclusion
Building custom filter functionality in Laravel applications can significantly improve the user experience and make data retrieval more efficient. By understanding the requirements, setting up the Laravel project, defining data models, creating filter routes, and implementing the filter functionality, developers can create powerful and user-friendly web applications. Custom filter functionality can be applied to various data elements, such as products, users, orders, and more, to enhance the overall usability of the application.
FAQs
Q: Can custom filter functionality be applied to multiple data models in Laravel?
A: Yes, custom filter functionality can be implemented for multiple data models in Laravel. You can follow similar steps to define data models, routes, and implement filter functionality for each data model.
Q: How can I handle complex filter criteria, such as date ranges and sorting in Laravel applications?
A: Laravel provides various tools and libraries to handle complex filter criteria, such as date ranges and sorting. You can use Laravel’s query builder to build dynamic queries based on the user’s input and apply sorting and date range filters accordingly.
Q: Are there any third-party packages or libraries that can simplify the implementation of custom filter functionality in Laravel?
A: Yes, there are several third-party packages and libraries available for Laravel that can simplify the implementation of custom filter functionality. One popular package is “Laravel Nova” which provides a powerful administration panel with built-in filter functionality for data models.
Overall, building custom filter functionality in Laravel applications requires a clear understanding of the requirements, effective use of Laravel’s features, and a focus on creating a seamless user experience. By following the steps outlined in this article, developers can create robust and efficient filter functionality to meet the needs of various web applications.