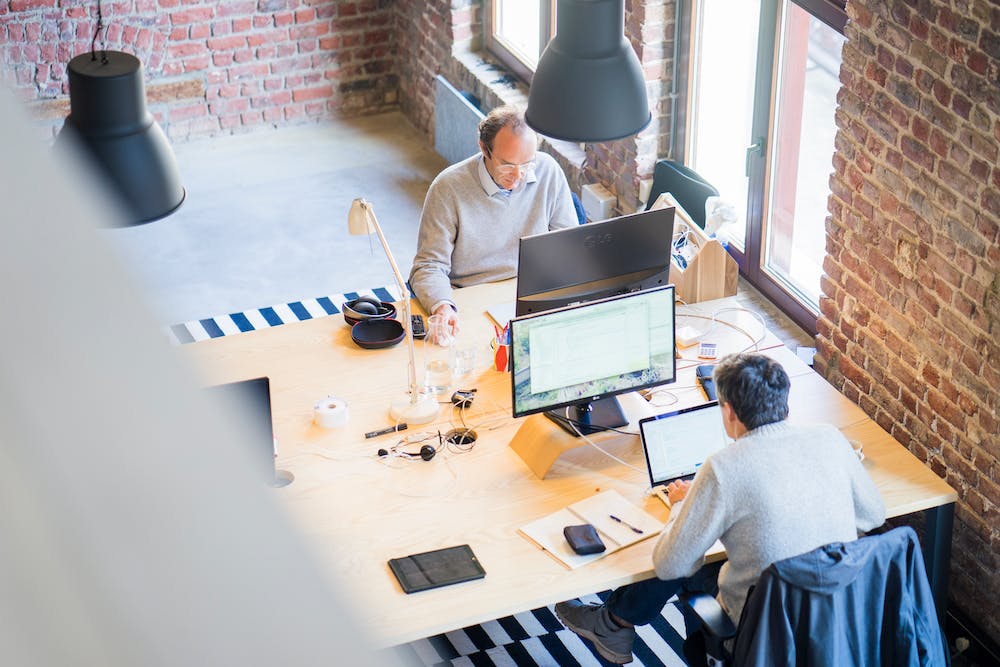
In today’s digital world, the demand for APIs (Application Programming Interfaces) has increased significantly. APIs allow different software systems to communicate and work together, enabling developers to create seamless integrations between different platforms. Laravel, a popular PHP framework, provides a powerful and elegant way to build APIs. In this tutorial, we will guide you through the process of building APIs with Laravel, step by step.
Prerequisites
Before we dive into building APIs with Laravel, you need to have a basic understanding of PHP and Laravel. If you are new to Laravel, we recommend going through the official Laravel documentation and completing the basic setup and installation. Additionally, you should have a good understanding of RESTful APIs and how they work.
Step 1: Setting Up Laravel
The first step in building APIs with Laravel is setting up a new Laravel project. If you already have a Laravel project set up, you can skip this step. To create a new Laravel project, open your terminal and run the following command:
composer create-project --prefer-dist laravel/laravel api-tutorial
This command will create a new Laravel project named “api-tutorial” in the current directory. Once the project is created, navigate to the project directory and start the Laravel development server using the following command:
php artisan serve
Now, you should be able to access your Laravel project at “http://localhost:8000” in your web browser.
Step 2: Creating a Model
Now that we have our Laravel project set up, IT‘s time to create a model for our API. For the purpose of this tutorial, let’s create a simple “Product” model. To create the “Product” model, run the following command in your terminal:
php artisan make:model Product -m
This command will create a new model file named “Product.php” in the “app” directory and a new migration file in the “database/migrations” directory. Open the migration file and define the schema for the “products” table. For example:
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->decimal('price', 8, 2);
$table->timestamps();
});
}
Once you have defined the schema, run the migration to create the “products” table in your database:
php artisan migrate
Step 3: Creating a Controller
Next, we need to create a controller for our “Product” model. Controllers in Laravel handle the logic for processing incoming requests and returning responses. To create a new controller for the “Product” model, run the following command:
php artisan make:controller ProductController
This command will create a new controller file named “ProductController.php” in the “app/Http/Controllers” directory. Open the controller file and define methods for retrieving, creating, updating, and deleting products. For example:
namespace App\Http\Controllers;
use App\Product;
use Illuminate\Http\Request;
class ProductController extends Controller
{
public function index()
{
$products = Product::all();
return response()->json($products);
}
public function show($id)
{
$product = Product::find($id);
return response()->json($product);
}
public function store(Request $request)
{
$product = new Product;
$product->name = $request->name;
$product->description = $request->description;
$product->price = $request->price;
$product->save();
return response()->json($product, 201);
}
public function update(Request $request, $id)
{
$product = Product::find($id);
$product->name = $request->name;
$product->description = $request->description;
$product->price = $request->price;
$product->save();
return response()->json($product);
}
public function destroy($id)
{
$product = Product::find($id);
$product->delete();
return response()->json(null, 204);
}
}
Step 4: Defining API Routes
With our model and controller in place, we now need to define API routes to handle incoming requests. In Laravel, routes are defined in the “routes/api.php” file. Open the “api.php” file and define the routes for our “Product” API. For example:
use App\Http\Controllers\ProductController;
use Illuminate\Support\Facades\Route;
Route::get('/products', [ProductController::class, 'index']);
Route::get('/products/{id}', [ProductController::class, 'show']);
Route::post('/products', [ProductController::class, 'store']);
Route::put('/products/{id}', [ProductController::class, 'update']);
Route::delete('/products/{id}', [ProductController::class, 'destroy']);
With these routes defined, our API is now ready to handle requests for listing, creating, updating, and deleting products.
Step 5: Testing the API
Now that we have our API set up, it’s time to test it. You can use a tool like Postman or curl to make HTTP requests to your API and test the endpoints. For example, you can use Postman to make a GET request to “http://localhost:8000/api/products” to retrieve a list of products, or a POST request to “http://localhost:8000/api/products” to create a new product.
Step 6: Authentication and Security
When building APIs, it’s important to consider security and authentication. Laravel provides a powerful authentication system that can be easily integrated into your API. You can use Laravel’s built-in authentication middleware to restrict access to certain endpoints, or you can implement token-based authentication using Laravel Passport or JSON Web Tokens (JWT).
Conclusion
Building APIs with Laravel is a straightforward and elegant process. With the power of Laravel’s Eloquent ORM, routing, and controllers, you can quickly create robust APIs to power your applications. By following the steps outlined in this tutorial, you should now have a good understanding of how to build APIs with Laravel and how to integrate them into your projects.
FAQs
Q: What is Laravel?
A: Laravel is a popular PHP framework for building web applications. It provides a rich set of features including routing, controllers, Eloquent ORM, and authentication, making it an excellent choice for building APIs.
Q: What is an API?
A: An API (Application Programming Interface) is a set of rules and protocols that allows different software applications to communicate with each other. APIs are commonly used to enable integrations between different systems and to expose functionality to external developers.
Q: Is Laravel a good choice for building APIs?
A: Yes, Laravel is a great choice for building APIs due to its elegant syntax, powerful features, and robust ecosystem. With Laravel, you can quickly build and deploy APIs that are secure, scalable, and easy to maintain.
Q: How can I secure my Laravel API?
A: You can secure your Laravel API by implementing authentication and authorization mechanisms. Laravel provides built-in support for token-based authentication using Laravel Passport or JSON Web Tokens (JWT). Additionally, you can use Laravel’s middleware to restrict access to certain endpoints based on user roles and permissions.
Q: Can I integrate third-party APIs with Laravel?
A: Yes, you can integrate third-party APIs with Laravel using packages and libraries. Laravel provides a clean and elegant way to make HTTP requests and handle API responses, making it easy to integrate with external APIs.