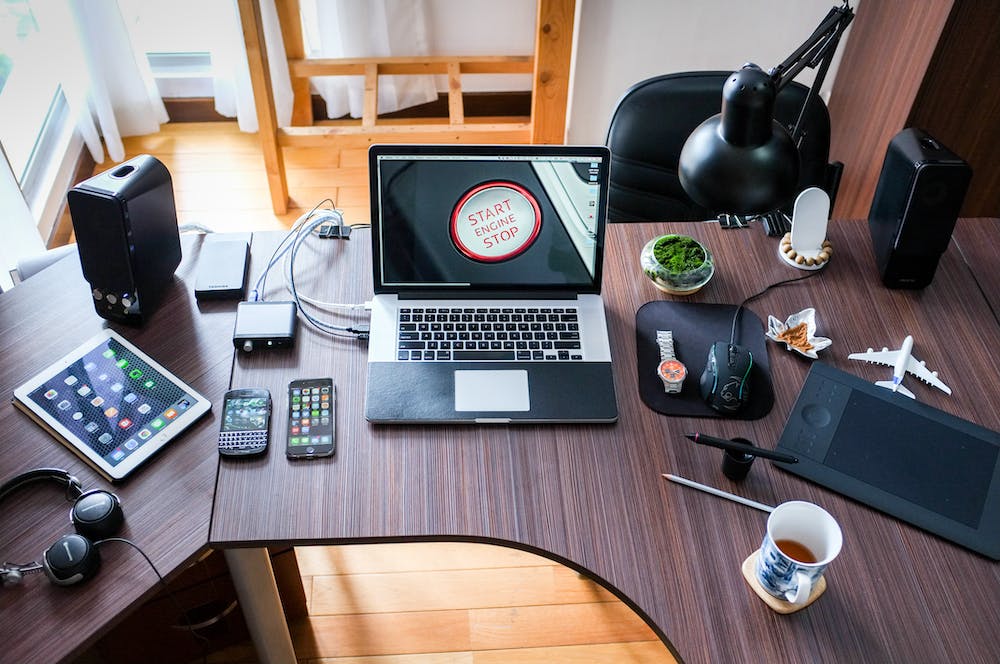
Tic Tac Toe is a classic game that is often used to introduce the concept of object-oriented programming (OOP) in Python. In this article, we will walk through the process of building a simple Tic Tac Toe game using OOP principles. By the end of this tutorial, you will have a better understanding of how to structure your code using classes and objects, as well as how to implement game logic and user interaction.
Setting Up the Project
Before we start coding, let’s set up a new Python project for our Tic Tac Toe game. We can create a new directory for the project and initialize IT with a virtual environment to manage our dependencies. Open your terminal and run the following commands:
$ mkdir tic-tac-toe
$ cd tic-tac-toe
$ python -m venv venv
$ source venv/bin/activate
Next, let’s create a new Python file for our game. You can use any text editor or IDE of your choice. We’ll name our file tic_tac_toe.py
. Open the file and let’s get started with the code!
Building the Game Board
Our Tic Tac Toe game will be played on a 3×3 grid. We can represent the game board as a 2-dimensional list, where each element represents a cell on the grid. To begin, let’s define a class called Board
to manage the game board.
class Board:
def __init__(self):
self.cells = [[' ' for _ in range(3)] for _ in range(3)]
In this code snippet, we define a constructor method __init__
that initializes the cells
attribute as a 3×3 2-dimensional list filled with empty strings. This represents the initial state of the game board.
Next, let’s add a method to our Board
class to display the current state of the game board:
def display(self):
for row in self.cells:
print(' | '.join(row))
print('--+---+--')
The display
method iterates through each row of the game board and prints the cell values separated by vertical bars. It also prints horizontal lines to represent the grid lines between the cells.
Implementing the Game Logic
Now that we have our game board set up, let’s move on to implementing the game logic. We can define a separate class called TicTacToe
to manage the game rules and player moves.
class TicTacToe:
def __init__(self):
self.board = Board()
self.current_player = 'X'
def switch_player(self):
self.current_player = 'O' if self.current_player == 'X' else 'X'
def make_move(self, row, col):
if self.board.cells[row][col] == ' ':
self.board.cells[row][col] = self.current_player
self.switch_player()
else:
print('Invalid move. Please try again.')
In this code snippet, we define a TicTacToe
class with methods to switch players and make moves on the game board. The make_move
method checks if the selected cell is empty, and if it is, it fills the cell with the current player’s symbol and switches to the next player. If the cell is already occupied, it prints a message indicating an invalid move.
Handling User Input
Now that we have the game logic in place, we need a way for players to interact with the game. We can create a simple command-line interface to prompt the players for their moves and display the game board.
def get_input(self):
while True:
try:
row = int(input('Enter the row (0, 1, or 2): '))
col = int(input('Enter the column (0, 1, or 2): '))
if 0 <= row <= 2 and 0 <= col <= 2:
return row, col
else:
print('Invalid input. Please enter a number between 0 and 2.')
except ValueError:
print('Invalid input. Please enter a number.')
In this code snippet, we define a get_input
method to prompt the players for their moves. The method uses a while
loop to continuously ask for input until valid row and column values are provided. It also handles non-numeric inputs with a try-except
block.
Running the Game
With all the pieces in place, we can now run our Tic Tac Toe game by instantiating the TicTacToe
class and running a game loop that prompts the players for their moves and displays the game board.
def play_game(self):
while True:
self.board.display()
print(f"Player {self.current_player}'s turn")
row, col = self.get_input()
self.make_move(row, col)
if self.check_winner():
self.board.display()
print(f"Player {self.current_player} wins!")
break
if self.is_board_full():
self.board.display()
print("It's a tie!")
break
def check_winner(self):
# TODO: Implement win condition check
pass
def is_board_full(self):
# TODO: Implement full board check
pass
if __name__ == '__main__':
game = TicTacToe()
game.play_game()
In the play_game
method, we display the current state of the game board, prompt the current player for their move, and check for a winner or a tie after each move. However, the check_winner
and is_board_full
methods are placeholders for now, as we have yet to implement the win condition and board full check logic.
Conclusion
Congratulations! You have successfully built a simple Tic Tac Toe game using object-oriented programming in Python. By following this tutorial, you have gained hands-on experience in organizing your code using classes and objects, implementing game logic, and handling user input. You can further extend the game by adding features such as a graphical user interface, computer AI, or multiplayer functionality. Keep exploring and have fun coding!
FAQs
Q: Can I use this code to create a web-based Tic Tac Toe game?
A: Yes, you can! You can use frameworks like Django or Flask to build a web application around the Tic Tac Toe game logic we’ve developed. You can also explore Javascript for client-side interactivity.
Q: How can I implement the win condition and board full check?
A: To implement the win condition, you can check for three matching symbols in a row, column, or diagonal on the game board. For the board full check, you can iterate through the cells and check if there are any empty spaces left.
Q: Can I incorporate machine learning to create a smart Tic Tac Toe AI?
A: Absolutely! You can explore machine learning algorithms such as reinforcement learning to train a Tic Tac Toe AI that can learn from playing against itself or human players. This can be a fun project to enhance the game with intelligent opponents.
Q: Are there libraries or frameworks that can help simplify the game development process?
A: Yes, there are libraries and frameworks available for Python game development, such as Pygame and Pyglet, which provide tools and utilities for building interactive games and graphical applications. However, building the game from scratch, as we have done in this tutorial, can help you gain a deeper understanding of the game development process.
Q: Can I use this project as a portfolio piece for showcasing my Python programming skills?
A: Absolutely! Building a Tic Tac Toe game using object-oriented programming in Python showcases your ability to structure code using OOP principles, handle user interactions, and implement game logic. It demonstrates your proficiency in Python programming and can be a valuable addition to your portfolio.