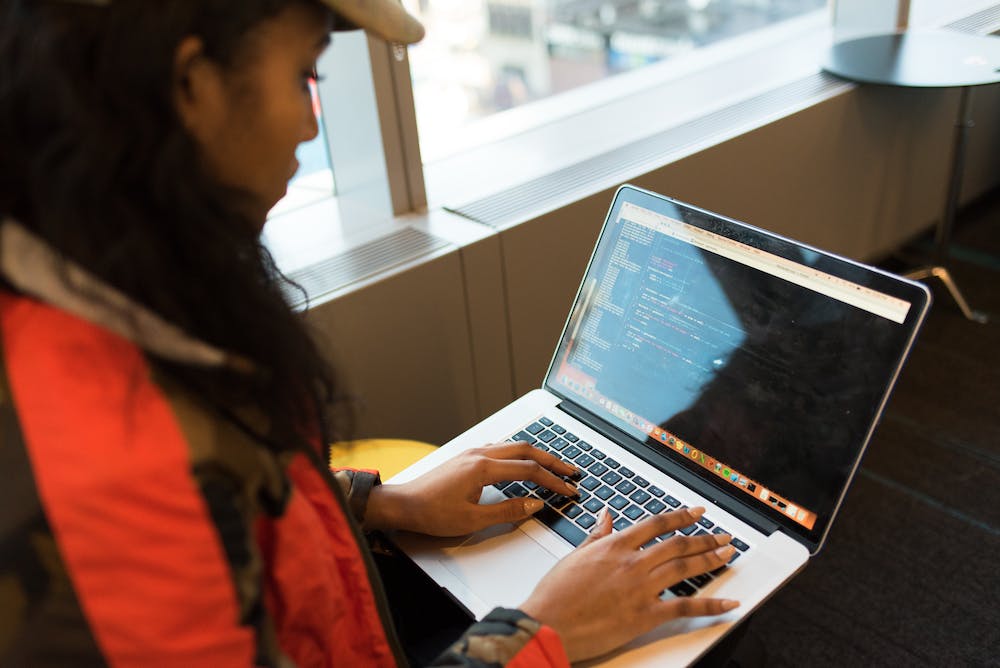
Welcome to this step-by-step guide on building a simple Python program! Python is a high-level programming language known for its simplicity and readability. Whether you’re a beginner or an experienced programmer, this article will walk you through the process of creating a basic Python program from scratch. So let’s get started!
Prerequisites
Before we begin, make sure you have Python installed on your computer. You can download the latest version of Python from the official Website. Once the installation is complete, you can open the Python interpreter or an Integrated Development Environment (IDE) to start writing your program.
Step 1: Defining the Problem
Before diving into coding, IT‘s important to have a clear understanding of the problem you want to solve. Clearly define the input and output requirements, as well as any specific constraints or conditions.
Step 2: Breaking Down the Solution
Once you have a clear understanding of the problem, break IT down into smaller subproblems. This will help you tackle the task in a more organized and manageable way. Consider the different steps or calculations required to reach the desired output.
Step 3: writing the Code
Now IT‘s time to start writing the actual code. Open your Python IDE or text editor and create a new Python file with a .py extension. Begin by defining any necessary variables, functions, or classes based on the subproblems identified earlier.
Let’s say our problem is to create a program that calculates the area of a rectangle. We can define a function named calculate_area()
which takes the length and width of the rectangle as inputs and returns the area as output.
def calculate_area(length, width):
area = length * width
return area
You can add comments to your code to make IT more understandable. In Python, comments are denoted by the #
symbol. For example:
# This function calculates the area of a rectangle
def calculate_area(length, width):
area = length * width
return area
Step 4: Testing the Program
IT‘s always a good practice to test your code before moving forward. You can create a few test cases to verify that your program is producing the expected results. In our example, we can call the calculate_area()
function with different lengths and widths to ensure the area is calculated correctly.
print(calculate_area(5, 10)) # Output: 50
print(calculate_area(3, 8)) # Output: 24
Run your program and check if the output matches the expected values. If the results are correct, you can proceed to the next step. Otherwise, you may need to review and debug your code for any errors.
Step 5: Packaging and Sharing
Once your program is working as expected, you may want to package IT to make IT more user-friendly or share IT with others. There are various ways to distribute Python programs, such as creating an executable file or publishing IT on package repositories. Research the most suitable method based on your specific requirements.
FAQs
Q: Why should I learn Python?
A: Python is one of the most popular programming languages due to its simplicity, versatility, and extensive library support. IT is widely used in various fields including web development, data analysis, machine learning, and scripting. Learning Python can open up numerous opportunities for you in the software development industry.
Q: Can I run Python programs on any operating system?
A: Yes, Python programs are designed to be platform-independent. You can run Python code on Windows, macOS, Linux, and even mobile operating systems like Android and iOS, as long as the necessary Python interpreter is installed.
Q: Do I need a fancy IDE to write Python programs?
A: No, you can simply use a text editor, like Notepad or Sublime Text, to write Python code. However, using an IDE, such as PyCharm or Visual Studio Code, can provide a more enhanced development environment with features like syntax highlighting, code completion, and easy debugging.
Q: Is Python only for beginners or can experienced programmers benefit from IT as well?
A: Python is suitable for beginners due to its easy-to-read syntax, but IT is also highly valued by experienced programmers. Python’s extensive libraries, such as NumPy, Pandas, and TensorFlow, make IT a powerful tool for scientific computing, data analysis, and machine learning.
Q: Can I build GUI (Graphical User Interface) applications with Python?
A: Yes, Python offers several libraries to build GUI applications, including Tkinter, PyQt, and wxPython. These libraries provide tools and widgets to create interactive, user-friendly interfaces for desktop applications.
That concludes our step-by-step guide on building a simple Python program. We hope this article has provided you with a solid foundation to start writing your own Python programs. Happy coding!