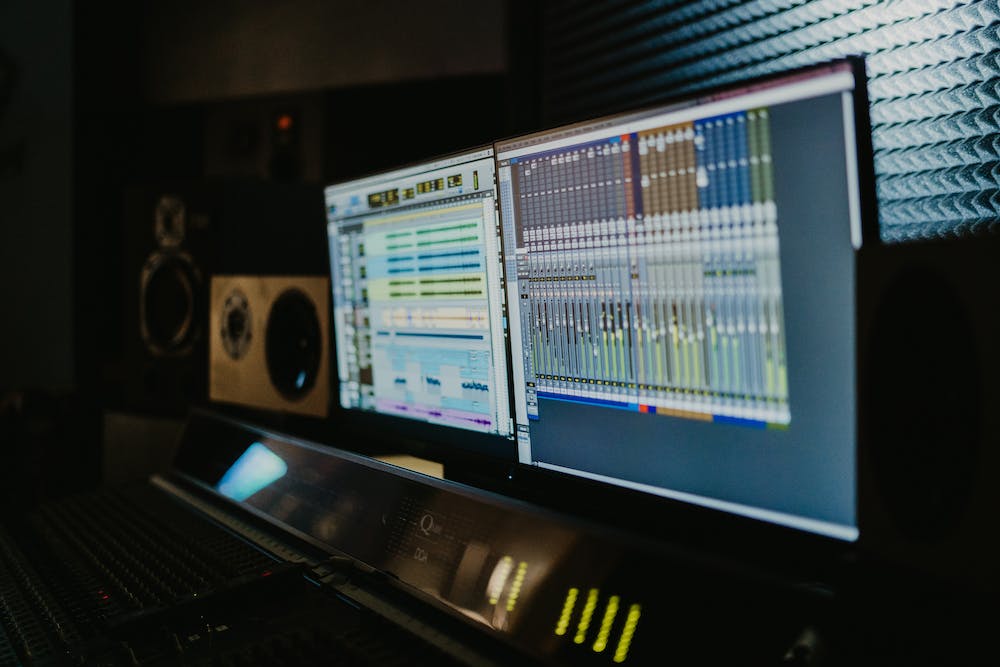
Introduction
Graphical User Interfaces (GUIs) play a crucial role in the usability and user experience of software applications. Python, with its simplicity and versatility, is an excellent choice for building GUI applications. Tkinter, the standard GUI toolkit for Python, provides a fast and easy way to create desktop applications with a graphical interface.
Prerequisites
Before diving into building a simple GUI application with Python and Tkinter, make sure you have Python installed on your system. You can download the latest version of Python at python.org and follow the installation instructions. Tkinter is included with most Python installations, so there’s no need for a separate installation.
Creating a Simple GUI Application
Let’s start by creating a simple GUI application that displays a window with a button. We’ll use this as the foundation to understand the basics of building GUI applications with Python and Tkinter.
Step 1: Importing the Tkinter Module
To begin, open your favorite text editor or integrated development environment (IDE) and import the Tkinter module at the top of your Python script:
import tkinter as tk
Step 2: Creating a Window
Now, let’s create the main window for our application:
root = tk.Tk()
Step 3: Adding a Button
We’ll add a button to the window to demonstrate user interaction in our GUI application:
button = tk.Button(root, text="Click Me")
Step 4: Packing the Button
To display the button in the window, we need to pack IT:
button.pack()
Step 5: Running the Application
Finally, we’ll run the application by entering the main event loop:
root.mainloop()
Enhancing the GUI Application
Now that we have a basic understanding of creating a simple GUI application with Python and Tkinter, let’s enhance our application by adding more elements such as labels, entry widgets, and event handling.
Adding Labels and Entry Widgets
We can add labels and entry widgets to our GUI application to take user input:
label = tk.Label(root, text="Enter your name:")
entry = tk.Entry(root)
label.pack()
entry.pack()
Handling Button Clicks
We can define a function to handle button clicks and display a message based on the user input:
def greet():
name = entry.get()
message = "Hello, " + name + "!"
greeting = tk.Label(root, text=message)
greeting.pack()
button = tk.Button(root, text="Greet", command=greet)
button.pack()
Styling the GUI Application
Tkinter allows us to style our GUI application using various options such as fonts, colors, and layout management. By customizing the appearance of our application, we can improve its visual appeal and user experience.
Customizing Fonts and Colors
We can change the font and color of text elements in our GUI application:
label = tk.Label(root, text="Customized Label", font=("Arial", 12), fg="blue")
label.pack()
Using Layout Management
Layout management in Tkinter allows us to position and organize the elements of our GUI application in a desired manner:
label1 = tk.Label(root, text="Label 1")
label2 = tk.Label(root, text="Label 2")
label1.pack(side="top")
label2.pack(side="bottom")
Conclusion
Building a simple GUI application with Python and Tkinter is a great way to get started with graphical programming. With the ability to create user-friendly interfaces and handle user interactions, Tkinter empowers developers to build interactive desktop applications efficiently.
FAQs
Q: Can I build complex GUI applications with Python and Tkinter?
A: Yes, Python and Tkinter can be used to build complex GUI applications with advanced features such as menus, dialogs, and canvas drawing.
Q: Is Tkinter the only GUI toolkit available for Python?
A: No, there are other GUI toolkits for Python such as PyQt, Kivy, and wxPython. Tkinter is the standard GUI toolkit included with Python installations.
Q: Can I create responsive design layouts with Tkinter?
A: Yes, Tkinter provides options for creating responsive design layouts by using grid and pack geometry managers.