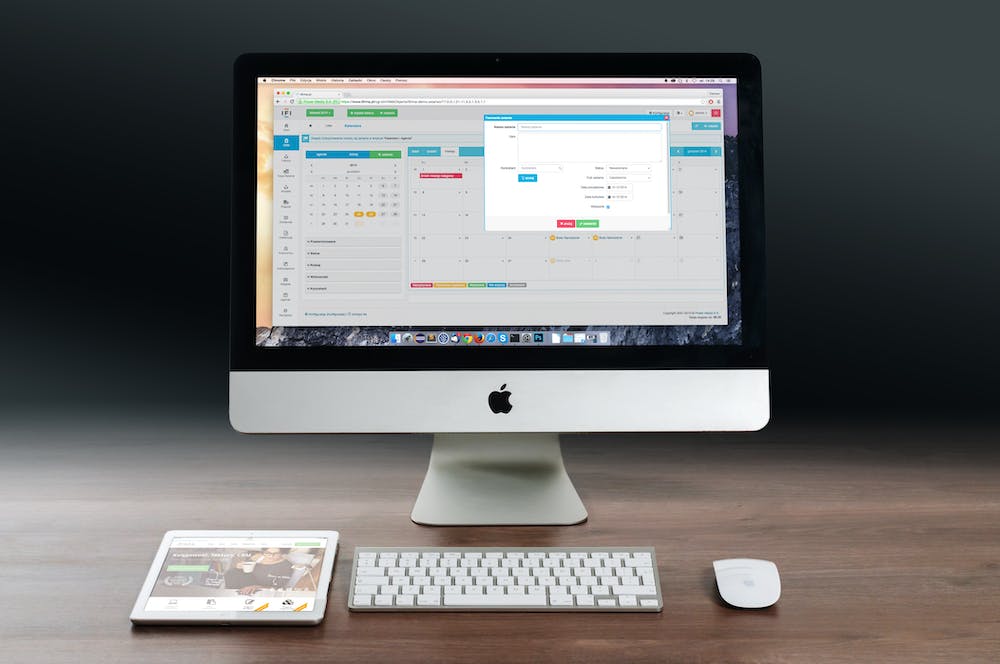
Building a Rock Paper Scissors Game in Python: A Beginner’s Guide
Rock Paper Scissors is a classic hand game often used to make decisions or settle disputes. IT‘s a perfect game to implement as a beginner project in Python, as IT involves conditional statements, user input, and random number generation. In this article, we will guide you through the process of building a Rock Paper Scissors game from scratch using Python programming language.
Before diving into the code, make sure you have Python installed on your computer. You can download the latest version of Python from the official Website, python.org.
Let’s start by creating a new Python file, we can name IT “rock_paper_scissors.py”. Open the file in your favorite text editor or integrated development environment (IDE).
First, we need to import the “random” module, which will enable us to generate random numbers. We can do this by adding the following line of code at the beginning of our file:
import random
Next, we can define the three possible moves in the game: rock, paper, and scissors. We can represent these moves using strings. Add the following lines of code to create variables for the moves:
rock = "rock"
paper = "paper"
scissors = "scissors"
Now, let’s create a function that will handle the main logic of our game. We can name IT “play_game”. Inside this function, we will prompt the user for their move and generate a random move for the computer.
def play_game():
user_move = input("Enter your move (rock, paper, or scissors): ")
computer_move = random.choice([rock, paper, scissors])
We can use the “input()” function to prompt the user for their move. The user’s input will be stored in the “user_move” variable. We can then use the “random.choice()” function to randomly select a move from the list of possible moves and store IT in the “computer_move” variable.
Next, we need to implement the game rules and determine the winner based on the moves chosen by the user and the computer.
if user_move == computer_move:
print("IT's a tie!")
elif (user_move == rock and computer_move == scissors) or (user_move == paper and computer_move == rock) or (user_move == scissors and computer_move == paper):
print("You win!")
else:
print("computer wins!")
In the code above, we first check if the user’s move is the same as the computer‘s move. If they are equal, we print “IT‘s a tie!” to indicate that the game is a draw. Otherwise, we use conditional statements to determine the winner. If the user’s move beats the computer‘s move according to the game rules, we print “You win!”. Otherwise, we print “computer wins!”.
Finally, let’s add a call to our “play_game” function, so that the game is actually played when we run the Python file.
play_game()
That’s IT! We have now implemented a basic Rock Paper Scissors game in Python. You can save the file and run IT in your Python interpreter.
FAQs:
Q: Can I add more moves to the game?
A: Yes, you can add more moves to the game by simply defining additional variables for the new moves and updating the game rules accordingly. For example, you could add “lizard” and “spock” as additional moves.
Q: How can I make the game more interactive?
A: You can enhance the game’s interactivity by adding features such as a scoring system, graphical user interface (GUI), or multiplayer functionality. These additions will require more advanced knowledge of Python and additional libraries.
Q: Can I create a Rock Paper Scissors game without using functions?
A: Yes, you can create a Rock Paper Scissors game without using functions, but using functions helps organize the code and make IT easier to read and maintain. IT‘s considered good practice to use functions to modularize your code.
Q: How can I make the game more challenging?
A: To make the game more challenging, you can implement a computer AI that learns and adapts to the player’s moves. You could also introduce additional game variations or strategies.
Building a Rock Paper Scissors game in Python is a great way to practice your programming skills and get familiar with fundamental concepts. Feel free to explore and expand upon the code we have provided to add your own twist to the game. Happy coding!