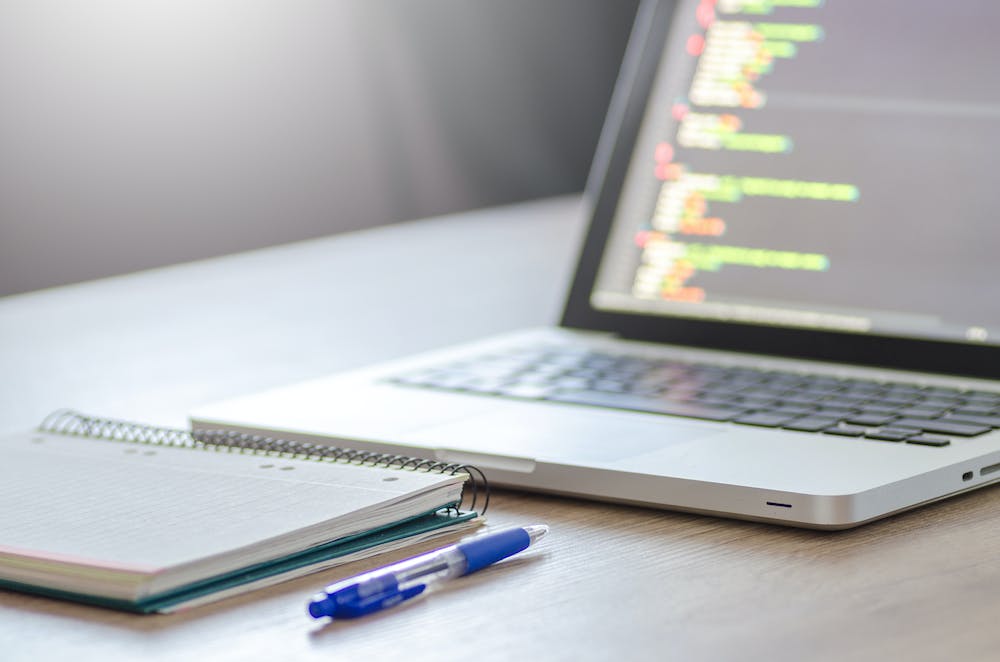
In today’s world of web development, building a RESTful API has become an essential skill for any programmer. A RESTful API allows you to create applications that can communicate with each other over the internet, opening up a world of possibilities for developers. In this beginner’s guide, we will walk through the process of building a RESTful API with PHP, one of the most popular programming languages for web development.
Setting up the Development Environment
Before we dive into building the RESTful API, let’s first set up the development environment. In order to follow along with this guide, make sure you have PHP installed on your machine. You can download the latest version of PHP from the official Website.
Once PHP is installed, we will also need a web server to run our API. The most common choice is Apache, but you can also use other web servers like Nginx. Install and configure the web server of your choice.
Creating a New Project
Now that our development environment is set up, let’s create a new project and set IT as the document root of our web server. Open your favorite code editor and create a new directory for your project. Inside that directory, create a new file called index.php
.
This index.php
file will serve as the entry point for our API. We will handle all the requests and responses within this file.
Routing and Handling Requests
In order to build a RESTful API, we need to define routes for different endpoints and handle incoming requests accordingly. PHP provides various methods to handle HTTP requests.
Let’s start by defining a route for the /users
endpoint. We will handle two types of requests for this endpoint – GET
to retrieve all users and POST
to create a new user.
$route = $_SERVER['REQUEST_URI'];
if ($route === '/users' && $_SERVER['REQUEST_METHOD'] === 'GET') {
// Handle GET request to retrieve all users
} elseif ($route === '/users' && $_SERVER['REQUEST_METHOD'] === 'POST') {
// Handle POST request to create a new user
} else {
// Handle invalid routes or methods
}
Connecting to a Database
In a real-world application, you would likely need to store user data in a database. Let’s connect our API to a MySQL database for this guide.
First, make sure you have MySQL installed on your machine. After that, create a new database and a table called users
to store user data.
$user = 'username';
$password = 'password';
$database = 'database_name';
$mysqli = new mysqli('localhost', $user, $password, $database);
if ($mysqli->connect_errno) {
die('Failed to connect to MySQL: ' . $mysqli->connect_error);
}
Retrieving and Creating Users
Now that we have a database connection, let’s implement the logic to retrieve and create users. For the GET
request to retrieve all users, we will execute a SQL query to fetch the data from the users
table.
$result = $mysqli->query('SELECT * FROM users');
$users = [];
while ($row = $result->fetch_assoc()) {
$users[] = $row;
}
echo json_encode($users);
For the POST
request to create a new user, we will retrieve the user data from the request body and insert IT into the database.
$data = json_decode(file_get_contents('php://input'), true);
$name = $data['name'];
$email = $data['email'];
$mysqli->query("INSERT INTO users (name, email) VALUES ('$name', '$email')");
echo 'User created successfully';
FAQs
What is a RESTful API?
A RESTful API is an architectural style for designing networked applications. IT uses HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources. A well-designed RESTful API follows a set of conventions and principles to create a scalable and maintainable application.
Why use PHP for building a RESTful API?
PHP is a widely-used server-side scripting language, especially suited for web development. IT has a large and active community, extensive documentation, and a wide range of frameworks and libraries. PHP is known for its ease of use and the ability to handle web requests efficiently, making IT a popular choice for building RESTful APIs.
What are some best practices for building a RESTful API?
Some best practices for building a RESTful API include using meaningful and semantically correct URLs, supporting multiple response formats (such as JSON and XML), providing proper error handling and status codes, using authentication and authorization mechanisms, and versioning the API to maintain backward compatibility. IT is also important to follow the principles of REST, such as statelessness and uniform interface.
How can I secure my RESTful API?
Securing a RESTful API involves implementing proper authentication and authorization mechanisms. You can use methods like token-based authentication, OAuth, or JSON Web Tokens (JWT) to authenticate users and control access to protected resources. IT is essential to validate and sanitize all user input to prevent common security vulnerabilities like SQL injection and cross-site scripting (XSS).
Congratulations! You have successfully built a RESTful API with PHP. This beginner’s guide covered the basics of setting up the development environment, handling requests, connecting to a database, and implementing CRUD operations. As you continue your journey in web development, remember to keep learning and exploring new possibilities with RESTful APIs!