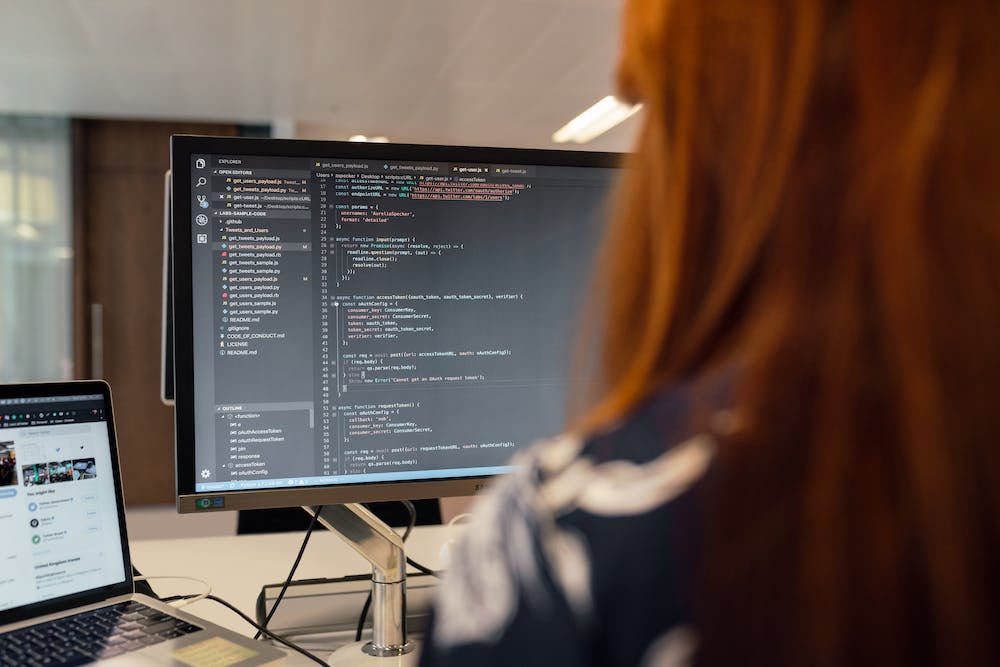
Laravel is a popular PHP framework known for its elegant syntax and powerful features. IT provides a solid foundation for building RESTful APIs that are performant, scalable, and maintainable. In this article, we will explore best practices for building RESTful APIs with Laravel and provide examples to demonstrate the implementation of these practices. Whether you are a beginner or an experienced developer, you will find valuable insights that can help you build high-quality APIs with Laravel.
Best Practices for Building RESTful APIs with Laravel
1. Use Resource Controllers
Resource controllers in Laravel provide a convenient way to organize your API endpoints and handle CRUD operations for resources. By leveraging resource controllers, you can ensure that your API endpoints follow RESTful conventions and are easy to maintain. Here’s an example of defining a resource controller for a “books” resource in Laravel:
Route::resource('books', 'BookController');
With this single line of code, Laravel will generate all the necessary routes for handling CRUD operations on the “books” resource, including endpoints for creating, reading, updating, and deleting books. This approach not only simplifies the API implementation but also promotes consistency and clarity in the API design.
2. Implement Authentication and Authorization
Security is a critical aspect of building RESTful APIs, and Laravel offers robust features for implementing authentication and authorization. You can utilize Laravel’s built-in authentication scaffolding to quickly set up user authentication mechanisms such as registration, login, and password reset. Additionally, Laravel’s middleware provides a flexible way to handle authorization logic for protected API endpoints.
For example, you can use the “auth” middleware to ensure that only authenticated users can access certain endpoints:
Route::get('profile', 'UserController@profile')->middleware('auth');
By incorporating authentication and authorization into your API, you can enforce access controls and protect sensitive resources from unauthorized usage.
3. Validate Input Data
Input validation is crucial for ensuring the consistency and integrity of data in your API. Laravel provides a convenient way to define validation rules for incoming requests using FormRequest classes. By creating custom FormRequest classes for your API endpoints, you can specify the validation rules and error messages in a structured manner.
Here’s an example of a FormRequest class for validating the input data when creating a new book:
class CreateBookRequest extends FormRequest
{
public function authorize()
{
return true;
}
public function rules()
{
return [
'title' => 'required|string',
'author' => 'required|string',
'published_at' => 'required|date',
];
}
}
By validating input data at the entry point of your API endpoints, you can ensure that the incoming data meets the expected criteria and prevent invalid or malicious input from compromising the integrity of your API.
4. Handle Errors Gracefully
Error handling is an essential aspect of building a robust API. In Laravel, you can leverage exception handling to gracefully handle errors and provide informative responses to API consumers. By defining custom exception handlers, you can control the way various types of errors are handled and formatted in the API responses.
For example, you can create a custom exception handler for handling API-specific exceptions and returning consistent error responses:
class ApiExceptionServiceProvider extends ServiceProvider
{
public function boot()
{
$this->renderable(function (ApiException $e, $request) {
return response()->json([
'error' => $e->getMessage()
], $e->getStatusCode());
});
}
}
By centralizing error handling in your API, you can ensure that error responses are uniform and informative, which can improve the developer experience and facilitate easier troubleshooting.
5. Optimize Query Performance
Efficient query performance is crucial for the responsiveness and scalability of your API. Laravel’s Eloquent ORM provides powerful tools for interacting with the database, but it’s important to be mindful of potential performance bottlenecks when querying large data sets. To optimize query performance, you can utilize techniques such as eager loading, caching, and query optimization.
For example, you can use eager loading to retrieve related data along with the primary resource to minimize the number of database queries:
$books = Book::with('author')->get();
By proactively addressing query performance considerations in your API, you can ensure that it delivers high responsiveness and can handle a growing volume of requests without degradation in performance.
Examples of Building RESTful APIs with Laravel
Let’s walk through some examples that demonstrate the implementation of the best practices mentioned above in building RESTful APIs with Laravel.
Example 1: Building a “books” Resource API
In this example, we’ll create a RESTful API for managing a collection of books using Laravel’s resource controllers, authentication middleware, and input validation.
First, let’s define the routes for our “books” resource and apply the “auth” middleware to protect the endpoints:
Route::middleware('auth:api')->group(function () {
Route::resource('books', 'BookController');
});
Next, we’ll create a FormRequest class for validating the input data when creating or updating a book:
class BookRequest extends FormRequest
{
public function authorize()
{
return true;
}
public function rules()
{
return [
'title' => 'required|string',
'author' => 'required|string',
'published_at' => 'required|date',
];
}
}
With these foundational components in place, we can now implement the BookController to handle CRUD operations for the “books” resource, ensuring that the API endpoints are secured and the input data is validated.
By following this example, you can build a well-structured and secure API for managing books, adhering to RESTful principles and best practices.
Example 2: Optimizing Query Performance for a “users” API
In this example, we’ll focus on optimizing query performance for a RESTful API that provides user-related endpoints. We’ll utilize Laravel’s eager loading and caching mechanisms to improve the efficiency of handling user data retrieval and manipulation.
Suppose we have an API endpoint for fetching user profiles along with their associated posts. We can use eager loading to retrieve the related posts alongside the user data in a single database query:
$users = User::with('posts')->get();
In addition to eager loading, we can implement caching to store frequently accessed user data and reduce the database load for repeated requests:
$users = Cache::remember('users', $minutes, function () {
return User::with('posts')->get();
});
By incorporating these optimization techniques into our API implementation, we can enhance the responsiveness and scalability of user-related operations, ultimately delivering a more efficient and performant API.
Conclusion
Building a RESTful API with Laravel involves adhering to best practices that emphasize security, performance, and maintainability. By leveraging Laravel’s powerful features for routing, authentication, validation, error handling, and query optimization, you can construct high-quality APIs that meet the demands of modern application development. Furthermore, incorporating these best practices into your API development workflow can result in APIs that are well-structured, secure, and efficient, ultimately providing a superior experience for API consumers and developers alike.
FAQs
Q: How does Laravel facilitate the implementation of authentication in RESTful APIs?
A: Laravel provides authentication scaffolding that includes preconfigured controllers, middleware, and routes for user authentication, allowing developers to quickly set up robust authentication mechanisms in their APIs.
Q: What are the benefits of using resource controllers in Laravel for building RESTful APIs?
A: Resource controllers in Laravel streamline the organization and implementation of CRUD operations for API resources, promoting consistency and clarity in the API design while reducing the effort required for endpoint setup and maintenance.
Q: How can Laravel’s query optimization features enhance the performance of RESTful APIs?
A: By leveraging techniques such as eager loading, caching, and query optimization, developers can improve the efficiency of database interactions in their APIs, resulting in reduced query latency and enhanced scalability.