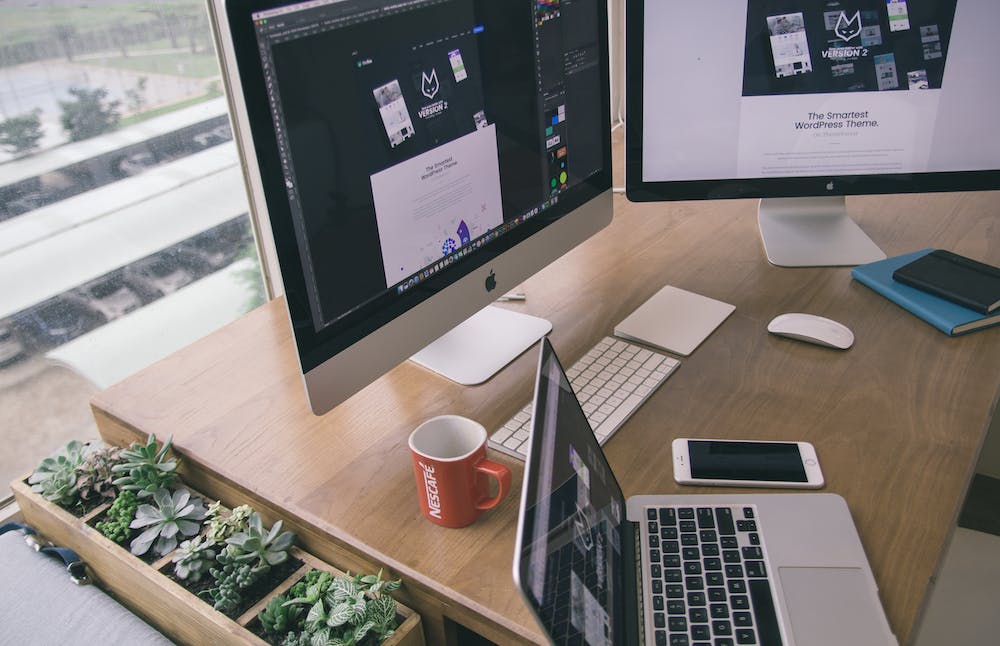
Building a RESTful API with Laravel can be a daunting task, especially if you are new to the framework. However, with a step-by-step guide, you can easily navigate through the process and create a powerful API for your web or mobile applications. In this article, we will walk you through the process of building a RESTful API using Laravel, from setting up your development environment to testing and deploying your API. So, let’s get started!
Frequently Asked Questions:
Q1. What is a RESTful API?
A1. RESTful API stands for Representational State Transfer Application Programming Interface. IT is an architectural style that allows communication between different software systems over the internet. REST APIs are stateless, meaning that each request from a client to a server contains all the information needed to understand and process the request.
Q2. Why use Laravel for building a RESTful API?
A2. Laravel is a PHP web framework known for its simplicity and elegance. IT provides a solid foundation for building robust and scalable web applications, including RESTful APIs. Laravel offers a wide range of features and tools specifically designed for API development, such as routing, serialization, authentication, and testing.
Q3. What are the steps to build a RESTful API with Laravel?
A3. Here are the steps to build a RESTful API with Laravel:
- Set up your development environment.
- Create a new Laravel project.
- Design your API routes.
- Create API controllers.
- Implement CRUD operations for your API.
- Handle authentication and authorization.
- Add validation and error handling.
- Test your API.
- Deploy your API.
Q4. How do I set up my development environment?
A4. To set up your development environment, you will need to install PHP, Composer, Laravel, and a web server like Apache or Nginx. You can follow the official Laravel documentation for detailed instructions on setting up your environment.
Q5. How do I create a new Laravel project?
A5. Once you have Laravel installed, open your command line interface and navigate to the directory where you want to create your project. Then, run the following command:
composer create-project --prefer-dist laravel/laravel your-project-name
Replace “your-project-name” with the desired name for your project.
Q6. How do I design my API routes?
A6. Laravel provides a convenient way to define API routes using the routes/api.php file. Open the file and define your API routes using the available route methods, such as get, post, put, patch, and delete. Don’t forget to specify the corresponding controller method for each route.
Q7. How do I create API controllers?
A7. Laravel offers a command-line tool called Artisan, which can be used to generate API controllers. Open your command line interface, navigate to your project directory, and run the following command to create a new API controller:
php artisan make:controller APIController --api
This command will generate a new APIController class in the app/Http/Controllers directory with the necessary boilerplate code for API development.
Q8. How do I implement CRUD operations for my API?
A8. Laravel provides resourceful routing, which allows you to define routes that handle the default CRUD operations (create, read, update, delete) for a resource. To implement CRUD operations for your API, define a resourceful route in routes/api.php and specify the corresponding controller methods in your API controller.
Q9. How do I handle authentication and authorization?
A9. Laravel offers built-in authentication and authorization functionality. You can use Laravel’s authentication middleware to protect your API routes and restrict access to authenticated users. Additionally, Laravel provides various authorization methods, such as gates and policies, to control access to specific API resources based on user roles and permissions.
Q10. How do I add validation and error handling to my API?
A10. Laravel provides a powerful validation system that allows you to define validation rules for incoming API requests. You can use Laravel’s validation middleware to automatically validate incoming requests before they reach your controller methods. Additionally, Laravel offers various error handling mechanisms, such as exception handling and error responses, to gracefully handle validation errors and other exceptions.
Q11. How do I test my API?
A11. Laravel provides a testing framework that allows you to write automated tests for your API. You can use Laravel’s built-in testing methods and assertions to test the functionality and behavior of your API routes and controllers. writing tests for your API ensures that IT works as expected and prevents regressions when making changes or adding new features.
Q12. How do I deploy my API?
A12. Deploying a Laravel API is similar to deploying any other Laravel application. You can use a hosting service or set up your own server environment. Laravel provides documentation on deploying Laravel applications, which you can follow to deploy your API to a production environment.
With this step-by-step guide and the power of Laravel, you can confidently build a RESTful API for your web or mobile applications. Remember to follow best practices, test your API thoroughly, and continuously improve its performance and security. Happy coding!