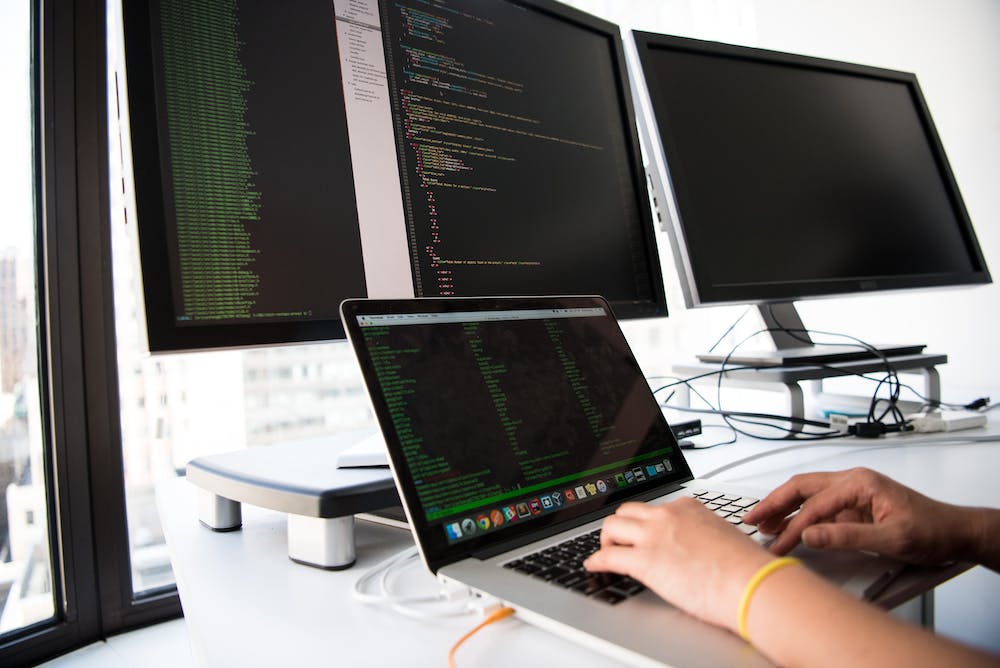
In recent years, Deno.js has gained popularity among developers as a secure and reliable runtime for JavaScript and TypeScript. With its built-in module system and excellent standard library, Deno.js provides an excellent platform for building web applications, including RESTful APIs. In this article, we will explore how to build a RESTful API using Deno.js and the Oak framework.
What is RESTful API?
Before we delve into building a RESTful API with Deno.js and Oak, let’s first understand what a RESTful API is. REST, which stands for Representational State Transfer, is an architectural style for designing networked applications. A RESTful API is an interface that allows systems to communicate with each other over the internet using the HTTP protocol. IT follows the principles of REST, such as stateless interactions, resource-based URLs, and standard HTTP methods (GET, POST, PUT, DELETE).
Getting Started with Deno.js
If you haven’t installed Deno.js yet, you can do so by running the following command in your terminal:
$ curl -fsSL https://deno.land/x/install/install.sh | sh
Once Deno.js is installed, you can verify the installation by running:
$ deno --version
Now that we have Deno.js installed, let’s move on to setting up our project.
Setting Up the Project
To create a new project, we can use the following commands:
$ mkdir deno-oak-api
$ cd deno-oak-api
$ deno --init
Now that our project is set up, let’s install Oak, which is a middleware framework for Deno.js, by running the following command:
$ deno install --allow-net --allow-read https://deno.land/x/oak/mod.ts
Building the RESTful API
Now that we have our project and dependencies set up, let’s start building our RESTful API using Deno.js and Oak.
First, let’s create a file named app.ts
, where we will define our API routes and their corresponding actions.
// app.ts
import { Application, Router } from 'https://deno.land/x/oak/mod.ts';
const app = new Application();
const router = new Router();
// Define routes
router.get('/api/products', (ctx) => {
ctx.response.body = 'List of products';
});
router.get('/api/products/:id', (ctx) => {
const { id } = ctx.params;
ctx.response.body = `Product ${id}`;
});
router.post('/api/products', async (ctx) => {
const body = await ctx.request.body();
ctx.response.body = `Added product: ${body.value.name}`;
});
router.put('/api/products/:id', async (ctx) => {
const { id } = ctx.params;
const body = await ctx.request.body();
ctx.response.body = `Updated product ${id} with ${body.value.name}`;
});
router.delete('/api/products/:id', (ctx) => {
const { id } = ctx.params;
ctx.response.body = `Deleted product ${id}`;
});
// Add the router to the app
app.use(router.routes());
app.use(router.allowedMethods());
// Start the app
await app.listen({ port: 8000 });
In this example, we have defined routes for listing products, getting a specific product by ID, adding a new product, updating an existing product, and deleting a product. We have used the HTTP methods GET, POST, PUT, and DELETE to handle different actions.
Running the API
To run our RESTful API, we can use the following command:
$ deno run --allow-net app.ts
Our API will now be accessible at http://localhost:8000
.
Conclusion
In this article, we have explored how to build a RESTful API using Deno.js and the Oak framework. We have seen how Deno.js provides a secure and reliable platform for building web applications, and how Oak simplifies the process of defining API routes and handling HTTP requests. By following the principles of REST and using the features provided by Deno.js and Oak, developers can create scalable and maintainable APIs for their web applications.
FAQs
Q: Can I use Deno.js for building production-ready APIs?
A: Yes, Deno.js is suitable for building production-ready APIs. It provides a secure runtime environment and a rich standard library that makes it well-suited for building web applications, including RESTful APIs.
Q: What are the advantages of using Oak for building APIs?
A: Oak provides a minimalist and expressive middleware framework for Deno.js, making it easy to define and handle HTTP routes, middleware, and requests. It simplifies the process of building APIs and encourages a clean and structured approach to defining RESTful endpoints.
Q: Is Deno.js compatible with other popular JavaScript frameworks and libraries?
A: Deno.js is compatible with many popular JavaScript frameworks and libraries, thanks to its built-in support for ECMAScript modules. This makes it easy to integrate with existing tools and libraries while building web applications and APIs.
Q: Are there any performance considerations when using Deno.js for building APIs?
A: Deno.js is designed for performance, and its architecture allows for efficient handling of I/O operations and asynchronous code. With its built-in TypeScript support and secure runtime environment, Deno.js provides a performant platform for building APIs that can scale to handle high loads.
Q: Can I use Deno.js and Oak to build APIs for enterprise applications?
A: Yes, Deno.js and Oak are suitable for building APIs for enterprise applications. Their secure and reliable nature, combined with their support for modern JavaScript and TypeScript features, makes them well-suited for building robust and scalable APIs for enterprise-grade web applications.