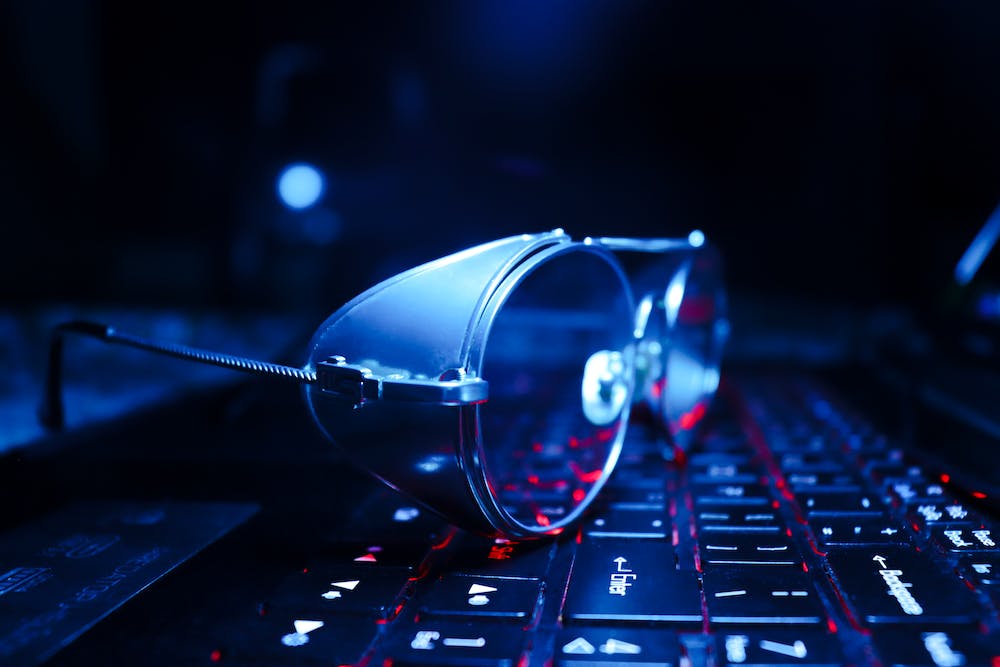
If you are building a Website that requires user login functionality, you will need a reliable and secure PHP login system. One crucial aspect of a login system is the ability to retrieve usernames. In this article, we will explore various methods to retrieve usernames using PHP and discuss some frequently asked questions related to this topic.
Method 1: Retrieving Usernames from a Database
If you store your user information in a database, one way to retrieve usernames is by querying the database. Let’s assume you have a table named “users” with columns “id”, “username”, and “password”. Here’s a code example:
“`php
// Connect to the database
$pdo = new PDO(‘mysql:host=localhost;dbname=your_database’, ‘username’, ‘password’);
// Prepare and execute a SELECT statement to retrieve usernames
$stmt = $pdo->prepare(‘SELECT username FROM users’);
$stmt->execute();
// Fetch all usernames as an array
$usernames = $stmt->fetchAll(PDO::FETCH_COLUMN);
// Loop through the array and display the usernames
foreach ($usernames as $username) {
echo $username . ‘
‘;
}
?>
“`
This code connects to the database using PDO, prepares a SELECT statement to retrieve usernames, executes the statement, and fetches all usernames as an array using `fetchAll`. Finally, IT loops through the array and displays the usernames.
Method 2: Retrieving Usernames from a File
If you store your user information in a file, another method to retrieve usernames is by reading the file. Let’s assume you have a file named “users.txt” where each line represents a user’s information with the format “username;password”. Here’s a code example:
“`php
// Read the file contents into an array
$users = file(‘users.txt’);
// Loop through the array to extract usernames
foreach ($users as $user) {
$userInfo = explode(‘;’, $user);
$username = $userInfo[0];
echo $username . ‘
‘;
}
?>
“`
This code reads the file contents into an array using `file` function, loops through the array, and extracts usernames using `explode` function.
FAQs:
Q: Can I retrieve usernames directly from the HTML form?
A: No, you cannot retrieve usernames directly from the HTML form. The form data is sent to the server, where PHP processes IT and retrieves the usernames using appropriate methods, such as querying a database or reading a file.
Q: How can I display only unique usernames?
A: You can display only unique usernames by using the `array_unique` function in PHP. For example, if you have retrieved the usernames using method 1, you can modify the code as follows:
“`php
$usernames = $stmt->fetchAll(PDO::FETCH_COLUMN);
$usernames = array_unique($usernames);
foreach ($usernames as $username) {
echo $username . ‘
‘;
}
“`
This code removes any duplicate usernames from the array before displaying them.
Q: Are there any security concerns when retrieving usernames?
A: When retrieving usernames, IT is essential to ensure the security of the data. Use appropriate database query methods, such as prepared statements, to prevent SQL injection attacks. If storing user information in a file, make sure the file is not accessible publicly, and consider encrypting sensitive data.
Building a PHP login system requires considering various aspects, including the retrieval of usernames. By utilizing the appropriate methods discussed above and addressing security concerns, you can develop a robust login system for your Website.