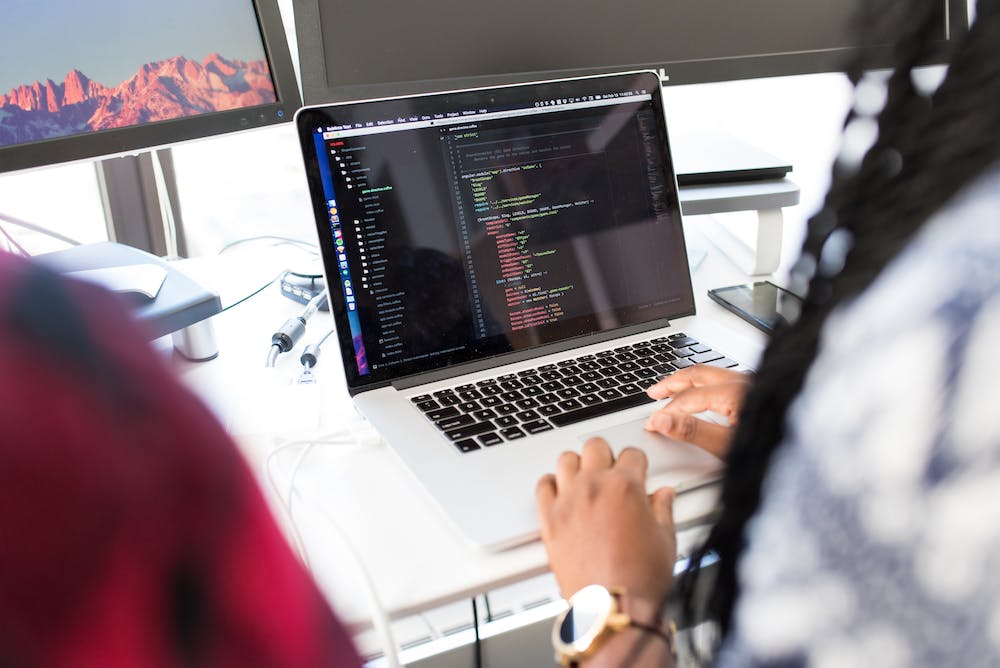
Python is a powerful and popular programming language that is widely used for various purposes, including web development, data analysis, artificial intelligence, and more. With its clean and readable syntax, Python makes IT easy for both beginners and experienced developers to write high-quality code. However, like any other programming language, there are certain best practices that can help you write more efficient, maintainable, and error-free Python code. In this article, we will discuss some of these best practices along with helpful tips and tricks to enhance your Python coding skills.
1. Follow the PEP 8 Style Guide
The Python community has created a style guide called “PEP 8” which outlines the recommended coding conventions for Python. Following this style guide not only makes your code consistent but also makes IT more readable and understandable for others. Some key guidelines include:
- Using 4 spaces for indentation
- Limiting lines to a maximum of 79 characters
- Naming variables and functions using lowercase letters and underscores (_) for multiple words
2. Use Descriptive Variable and Function Names
Choosing meaningful and descriptive names for your variables and functions improves code readability and reduces the need for comments. Avoid using single-letter variable names or generic names that don’t convey their purpose. For example, instead of using x = 5
, use something like num_of_students = 5
to indicate its purpose.
3. Efficiently Import Modules
Importing Python modules can sometimes be slow, especially if you import the entire module when you only need a specific function or class from IT. To improve performance, only import what you need using the from module import name
syntax. Additionally, avoid using wildcard imports (from module import *
) as IT can make IT harder to track down where certain functions or variables come from.
4. Use List Comprehensions and Generators
Python provides powerful features like list comprehensions and generators that allow you to write concise and efficient code. List comprehensions provide a compact way to create lists based on existing lists, while generators are memory-efficient and lazily evaluate items on demand. When appropriate, leverage these features to write cleaner and more efficient code.
5. Avoid Repetition with Functions and Classes
If you find yourself writing the same code multiple times, consider refactoring IT into functions or classes. Functions allow you to encapsulate reusable pieces of code, improving readability and promoting the DRY (Don’t Repeat Yourself) principle. Similarly, classes enable you to group related functions and variables together, making your code more organized and manageable.
6. Handle Exceptions Properly
When writing code that can potentially raise exceptions, IT‘s essential to handle them gracefully. Avoid using bare except
statements, as they catch all exceptions, including those you might not be aware of. Instead, use specific exception types or create custom exception classes to handle different error scenarios. Additionally, provide meaningful error messages to help with debugging and troubleshooting.
7. Write Unit Tests
Unit tests play a critical role in ensuring the correctness of your code. By writing tests for your functions and classes, you can verify that they behave as expected in different scenarios and catch any potential bugs or regressions. There are several frameworks, such as unittest
and pytest
, available for writing and executing unit tests in Python.
Frequently Asked Questions (FAQs)
Q: Why should I follow the PEP 8 style guide?
A: Following the PEP 8 style guide improves code consistency, readability, and makes IT easier for others to understand and maintain your code. IT also promotes a standardized coding style across the Python community.
Q: Can I use CamelCase for variable and function names?
A: According to the PEP 8 style guide, Python variables and functions should be named using lowercase letters with underscores (_) for multiple words. CamelCase is typically used for class names.
Q: What is the difference between a list comprehension and a generator?
A: List comprehensions are used to create new lists based on existing lists by applying certain conditions or transformations. They eagerly evaluate the entire list and return IT as a whole. On the other hand, generators yield items lazily, one at a time, without storing them in memory. Generators are memory-efficient and suitable for dealing with large datasets.
Q: How can I check if my code is working correctly?
A: writing unit tests using frameworks like unittest
or pytest
enables you to automatically verify the correctness of your code. Unit tests help catch bugs and regressions by testing individual components, functions, or classes in isolation.
By following these best practices and utilizing the tips and tricks mentioned above, you can greatly enhance your Python coding skills and produce more robust and maintainable code. Remember, coding is not just about solving the problem at hand but also about writing clean, readable, and efficient code that can be easily understood and maintained by others.