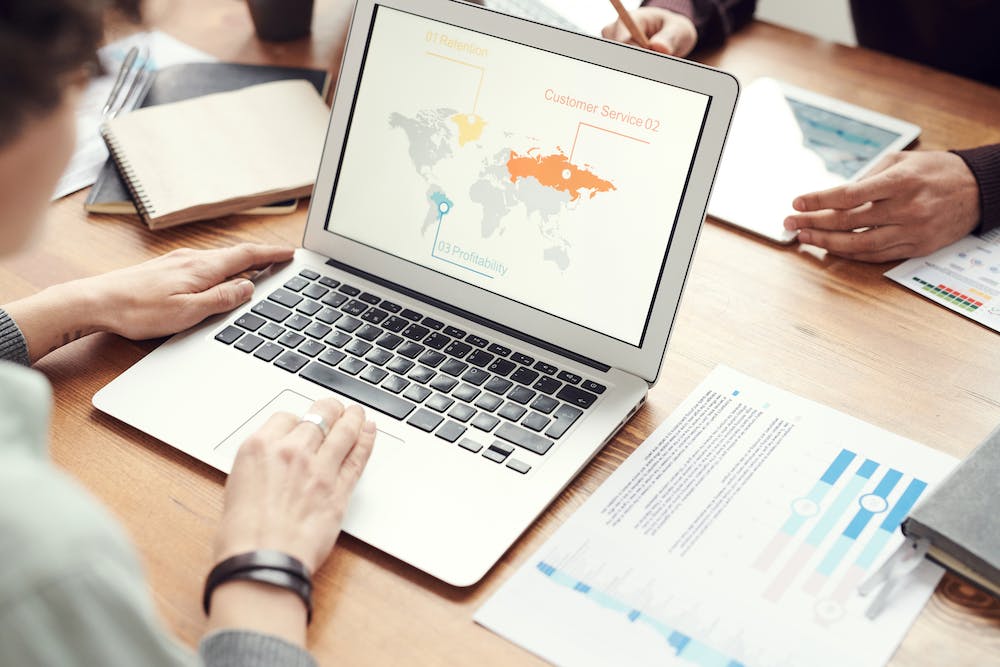
MD5 hashing is a widely-used cryptographic hash function that produces a 128-bit (16-byte) hash value. IT is commonly used to store passwords securely in databases and to verify the integrity of files. In this article, we will explore the best practices for implementing MD5 hashing in PHP to ensure security and efficiency.
1. Use PHP’s Built-in MD5 Function
PHP provides a built-in function md5()
to calculate the MD5 hash of a string. It is recommended to use this function for MD5 hashing as it is optimized for performance and security. Here’s an example of how to use the md5()
function:
$hash = md5('hello world');
echo $hash; // output: 5eb63bbbe01eeed093cb22bb8f5acdc3
2. Salted Hashing
Salted hashing is a technique to add additional random data (known as a salt) to the input before hashing. This makes it harder for attackers to use precomputed tables (rainbow tables) to crack the hashed values. Here’s an example of how to use salted hashing with MD5 in PHP:
$password = 'hello world';
$salt = 'random_salt';
$hash = md5($password . $salt);
echo $hash; // output: 8476ee4631b9b30ba06dd3ca838e16b5
3. Use Modern Hashing Algorithms
While MD5 hashing is widely used, it is considered to be cryptographically broken and unsuitable for further use. It is recommended to use more secure hashing algorithms such as SHA-256 or bcrypt for storing passwords. However, MD5 hashing can still be used for non-security-critical applications such as file integrity checks.
4. Protect Against Collision Attacks
MD5 hashing is vulnerable to collision attacks, where two different input strings produce the same hash value. To protect against collision attacks, it is important to validate the input data and use a secure hashing algorithm for security-critical applications.
5. Use Strong Input Validation
Before hashing any input data, it is important to perform strong input validation to prevent any malicious or malformed input from causing security vulnerabilities. Input validation can include checking data types, length, and format to ensure that only valid data is being hashed.
6. Secure Storage of Hashed Values
When storing hashed values in a database, it is important to ensure that the database is secure and protected against unauthorized access. Additionally, it is recommended to use a secure hashing algorithm for storing passwords, such as bcrypt, which is designed to be slow and computationally intensive to make brute-force attacks more difficult.
7. Periodic Review and Updates
Security best practices are continuously evolving, and it is important to periodically review and update the hashing algorithms and security measures in your PHP application. Keeping up to date with the latest security guidelines and recommendations can help ensure the continued security of your application.
Conclusion
In conclusion, implementing MD5 hashing in PHP requires careful consideration of best practices to ensure security and efficiency. By using PHP’s built-in md5()
function, implementing salted hashing, using modern hashing algorithms, protecting against collision attacks, employing strong input validation, securing the storage of hashed values, and periodically reviewing and updating security measures, developers can effectively implement MD5 hashing in their PHP applications.
FAQs
Q: Is MD5 hashing secure?
A: While MD5 hashing is widely used, it is considered to be cryptographically broken and unsuitable for further use in security-critical applications. It is recommended to use more secure hashing algorithms such as SHA-256 or bcrypt for storing passwords.
Q: Can I use MD5 hashing for non-security-critical applications?
A: Yes, MD5 hashing can still be used for non-security-critical applications such as file integrity checks. However, it is important to be aware of its vulnerabilities and limitations.
Q: What is salted hashing?
A: Salted hashing is a technique to add additional random data (known as a salt) to the input before hashing. This makes it harder for attackers to use precomputed tables (rainbow tables) to crack the hashed values.
Q: How often should I update the hashing algorithms in my PHP application?
A: It is recommended to periodically review and update the hashing algorithms and security measures in your PHP application to keep up to date with the latest security guidelines and recommendations.
Q: Can MD5 hashing be used for verifying file integrity?
A: Yes, MD5 hashing can be used for verifying the integrity of files. However, it is important to be aware of its vulnerabilities and limitations, particularly in the context of collision attacks.