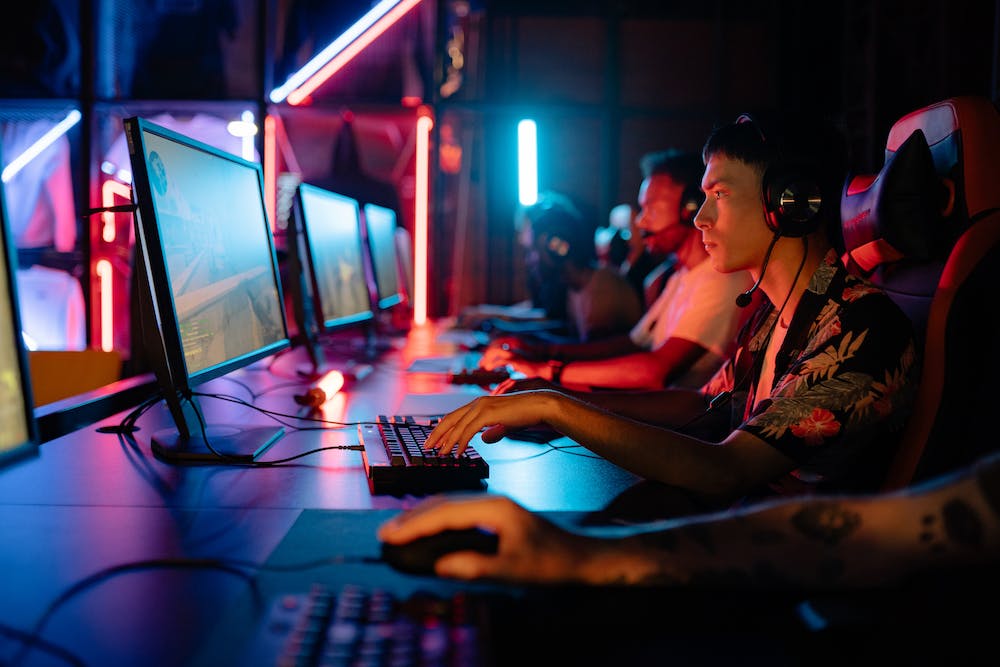
Python is a versatile and powerful programming language that is widely used in various fields, including web development, data analysis, artificial intelligence, and more. If you’re new to programming, learning Python can be a great way to start your journey in the world of coding. In this beginner’s guide, we will walk you through the basics of writing basic Python programs.
Setting Up Your Environment
Before you start writing Python programs, you need to set up your environment. The first step is to install Python on your computer. You can download the latest version of Python from the official Website and follow the installation instructions. Once Python is installed, you can use a text editor or an integrated development environment (IDE) to write and run your Python code. Some popular text editors and IDEs for Python include Sublime Text, Atom, PyCharm, and Visual Studio Code.
Writing Your First Python Program
Now that your environment is set up, let’s write your first Python program. Open your text editor or IDE and create a new file. In this file, you can write a simple “Hello, World!” program, which is a traditional first program for beginners in any programming language. Here’s the code to print “Hello, World!”:
“`python
print(“Hello, World!”)
“`
Once you’ve written the code, save the file with a .py extension, such as hello_world.py. You can then run the program by opening a terminal or command prompt, navigating to the directory where the file is saved, and typing python hello_world.py. You should see “Hello, World!” printed to the console.
Basic Syntax and Concepts
As you start writing more complex Python programs, IT‘s important to familiarize yourself with the basic syntax and concepts of the language. Python uses indentation to indicate blocks of code, so proper indentation is crucial for the program to run correctly. In addition, Python is a dynamically typed language, which means you don’t have to declare the data type of a variable when you create it.
Python also has a rich standard library that provides built-in functions and modules for various tasks, such as working with files, handling strings, and performing mathematical operations. Understanding how to use the standard library can save you time and effort when writing Python programs.
Variables and Data Types
In Python, variables are used to store data that can be referenced and manipulated in your programs. When you create a variable, you can assign a value to it using the assignment operator (=). Python supports various data types, including integers, floats, strings, lists, tuples, dictionaries, and more. Here are some examples of how to use variables and data types in Python:
“`python
# Integer
age = 25
# Float
height = 1.75
# String
name = “Alice”
# List
fruits = [“apple”, “banana”, “orange”]
# Tuple
coordinates = (3, 4)
# Dictionary
person = {“name”: “Bob”, “age”: 30}
“`
Understanding how to use variables and data types will allow you to manipulate and process different kinds of data in your Python programs.
Control Flow and Loops
Control flow statements, such as if-else and switch-case, allow you to make decisions and execute different blocks of code based on certain conditions. Loops, such as for and while, enable you to repeat a block of code multiple times. Here are some examples of how to use control flow and loops in Python:
“`python
# if-else statement
x = 10
if x > 0:
print(“Positive”)
elif x < 0:
print(“Negative”)
else:
print(“Zero”)
# for loop
fruits = [“apple”, “banana”, “orange”]
for fruit in fruits:
print(fruit)
# while loop
i = 0
while i < 5:
print(i)
i += 1
“`
Understanding control flow and loops is essential for writing programs that can make decisions and perform repetitive tasks.
Functions
Functions allow you to encapsulate a block of code that can be reused throughout your program. In Python, you can define a function using the def keyword, followed by the function name and its parameters. Here’s an example of how to define and call a function in Python:
“`python
def greet(name):
print(“Hello, ” + name)
greet(“Alice”)
“`
Functions are a fundamental building block of Python programming and are essential for writing modular and maintainable code.
Conclusion
Congratulations! You’ve completed the beginner’s guide to writing basic Python programs. With the knowledge and skills you’ve gained, you’re ready to start exploring more advanced topics in Python programming, such as object-oriented programming, file handling, and working with external libraries. Keep practicing and experimenting with Python, and soon you’ll be able to create powerful and innovative programs.
FAQs
1. What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. It is widely used in various fields, including web development, data analysis, artificial intelligence, and more.
2. What are the basic data types in Python?
Python supports various data types, including integers, floats, strings, lists, tuples, dictionaries, and more.
3. What is the best text editor for writing Python code?
There are several text editors and IDEs that are popular for writing Python code, including Sublime Text, Atom, PyCharm, and Visual Studio Code. The best one for you will depend on your preferences and workflow.
4. How can I learn more about Python programming?
There are many online resources, tutorials, and courses available for learning Python programming. You can also join online communities and forums to connect with other Python enthusiasts and seek help with your programming challenges.