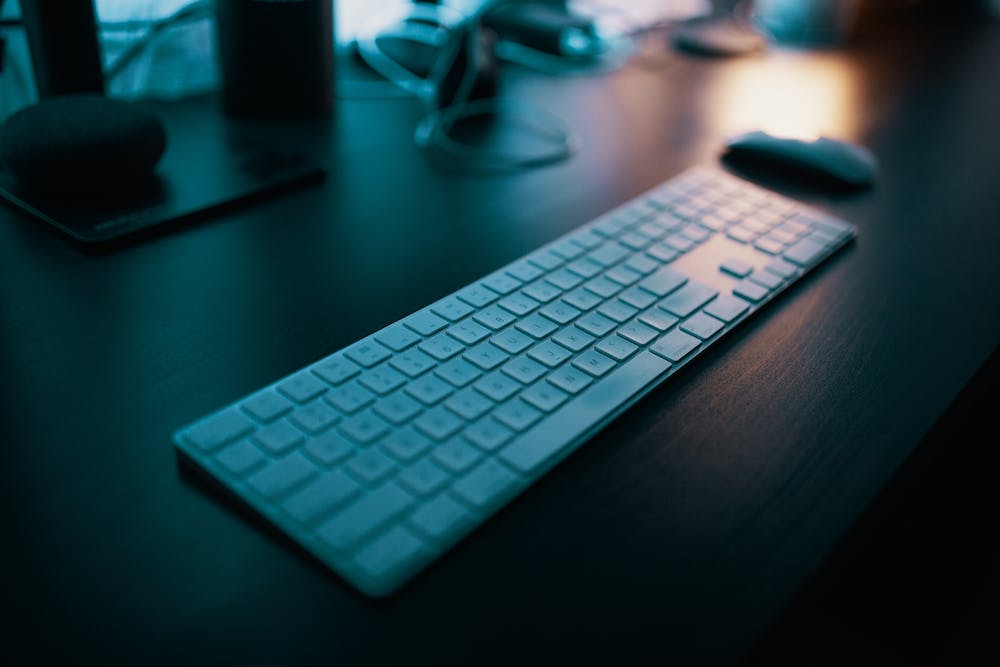
PHP is a powerful server-side scripting language that is widely used for creating dynamic webpages and applications. One of the key features of PHP is its ability to retrieve and manipulate data from URLs. In this beginner’s guide, we will explore how to get URL parameters in PHP and understand the various methods to accomplish this task.
To begin with, IT is essential to understand what URL parameters are. URL parameters are the values that are passed in the URL after a question mark (?). They are used to send data from one page to another. For example, consider the following URL: www.example.com/page.php?id=123&name=John
. Here, the parameters are id=123
and name=John
.
Now, let’s dive into the different methods available in PHP to retrieve these URL parameters:
1. Using the $_GET superglobal
The most straightforward and commonly used method to get URL parameters in PHP is by using the $_GET
superglobal. This superglobal is an associative array that contains key-value pairs of all the parameters present in the URL.
For example, to retrieve the value of the parameter id
from the URL mentioned earlier, you can use the following code:
$id = $_GET['id'];
The value of $id
will now be ‘123’.
IT is important to note that the values obtained using $_GET
are always strings, even if the actual value is an integer or any other data type. If you need to use the value as a specific data type, you can typecast IT accordingly.
2. Using the $_REQUEST superglobal
In addition to $_GET
, PHP provides another superglobal called $_REQUEST
. This superglobal is a combination of $_GET
, $_POST
, and $_COOKIE
arrays, making IT useful in situations where data is sent using different methods.
Retrieving URL parameters using $_REQUEST
is similar to using $_GET
. For example, to retrieve the value of the parameter name
from the earlier URL, you can use the following code:
$name = $_REQUEST['name'];
The value of $name
will now be ‘John’.
3. Using the parse_str() function
If you prefer a more manual approach, you can use the parse_str()
function in PHP to parse the query string of the URL and retrieve the parameters individually.
The parse_str()
function parses the query string and sets the variables in the current scope. For example, consider the following code:
parse_str($_SERVER['QUERY_STRING'], $params);
Now, the values are stored in the associative array $params
. You can access the parameters using their names as keys. For instance:
$id = $params['id'];
FAQs
Q: Can I retrieve multiple parameters at once?
A: Yes, you can retrieve multiple parameters at once by using the appropriate superglobal or the parse_str()
function. Simply access each parameter individually by specifying its name.
Q: What happens if a parameter is not present in the URL?
A: If a parameter is not present in the URL, attempting to access IT using the superglobals will result in a notice or warning. IT is good practice to check if a parameter exists before accessing IT to avoid such errors.
Q: Can I modify or remove URL parameters?
A: While you can retrieve URL parameters using the methods mentioned above, modifying or removing them directly from the URL is not possible through PHP. These parameters are part of the URL itself and can only be manipulated on the client-side using JavaScript or by redirecting to a new URL with the desired parameters.
Overall, understanding how to get URL parameters in PHP is crucial for building dynamic webpages that interact with user input and other data sources. By using the superglobals or the parse_str()
function, you can easily retrieve and utilize these values to enhance the functionality of your PHP applications.