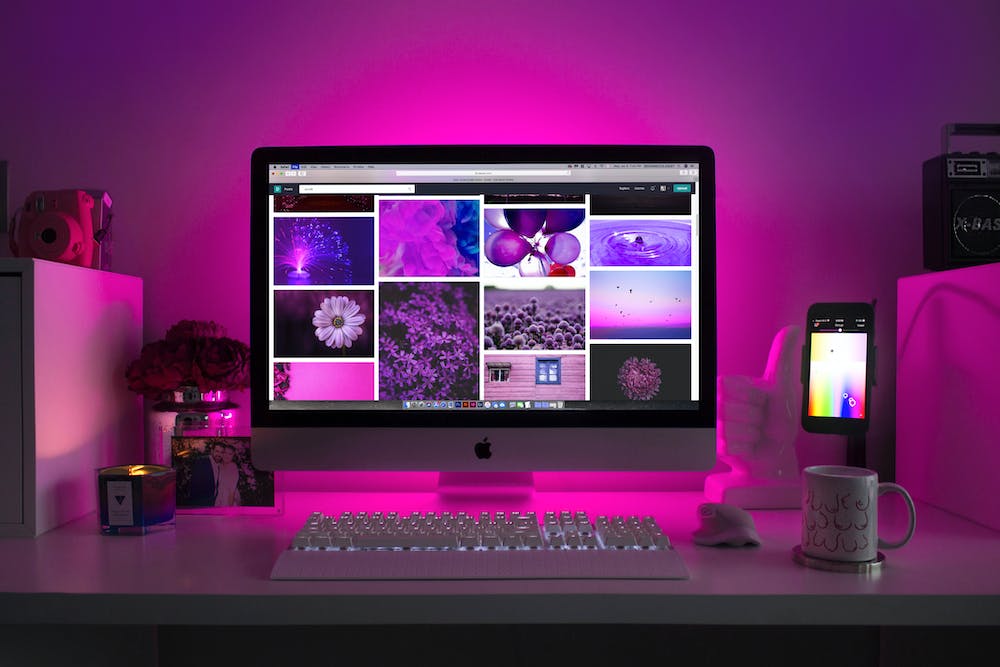
Object-oriented programming (OOP) is a programming paradigm that revolves around the concept of objects. Objects are instances of classes, which can contain both data and methods. OOP allows for the organization of code into reusable and modular components, making IT easier to manage and maintain large codebases. In this guide, we will cover the basics of OOP in PHP for beginners.
Classes and Objects
In PHP, a class is a blueprint for creating objects. IT defines the properties (variables) and methods (functions) that the objects will have. Here’s an example of a simple class in PHP:
class Car {
public $brand;
public $model;
public function displayInfo() {
echo "This is a {$this->brand} {$this->model}.";
}
}
To create an object from this class, we use the new
keyword:
$myCar = new Car();
$myCar->brand = "Toyota";
$myCar->model = "Corolla";
$myCar->displayInfo(); // Output: This is a Toyota Corolla.
Encapsulation
Encapsulation is the concept of bundling the data (properties) and methods that operate on the data into a single unit. In OOP, this is achieved through access modifiers such as public
, private
, and protected
. For example:
class BankAccount {
private $balance;
public function deposit($amount) {
$this->balance += $amount;
}
public function withdraw($amount) {
$this->balance -= $amount;
}
}
In this example, the $balance
property is encapsulated within the BankAccount
class, and can only be modified through the deposit()
and withdraw()
methods.
Inheritance
Inheritance is the concept of creating a new class based on an existing class. The new class, known as a child class or subclass, inherits the properties and methods of the existing class, known as the parent class or superclass. This allows for code reuse and the creation of hierarchical relationships between classes. Here’s an example:
class Animal {
public function makeSound() {
echo "Some sound";
}
}
class Dog extends Animal {
public function makeSound() {
echo "Bark";
}
}
In this example, the Dog
class inherits the makeSound()
method from the Animal
class, but overrides IT to produce a different sound.
Polymorphism
Polymorphism is the concept of using a single interface to represent different types of objects. This allows for the creation of flexible and reusable code. In PHP, polymorphism is often achieved through method overriding. Here’s an example:
interface Shape {
public function calculateArea();
}
class Circle implements Shape {
private $radius;
public function calculateArea() {
return pi() * $this->radius * $this->radius;
}
}
class Square implements Shape {
private $side;
public function calculateArea() {
return $this->side * $this->side;
}
}
In this example, both the Circle
and Square
classes implement the Shape
interface and provide their own implementation of the calculateArea()
method.
Conclusion
Object-oriented programming is a powerful paradigm for organizing and managing code in PHP. By understanding the principles of classes, objects, encapsulation, inheritance, and polymorphism, you can create more efficient, maintainable, and scalable code. As you continue to learn and practice OOP in PHP, you will gain a deeper understanding of how to leverage its capabilities to solve complex problems and build robust applications.
FAQs
What are the benefits of using OOP in PHP?
OOP allows for better code organization, reusability, and maintainability. IT also enables the creation of complex and hierarchical relationships between objects, leading to more efficient and scalable applications.
What is the difference between public, private, and protected access modifiers?
Public: Properties and methods with public access modifier can be accessed from outside the class.
Private: Properties and methods with private access modifier can only be accessed from within the class.
Protected: Properties and methods with protected access modifier can be accessed within the class and its subclasses.