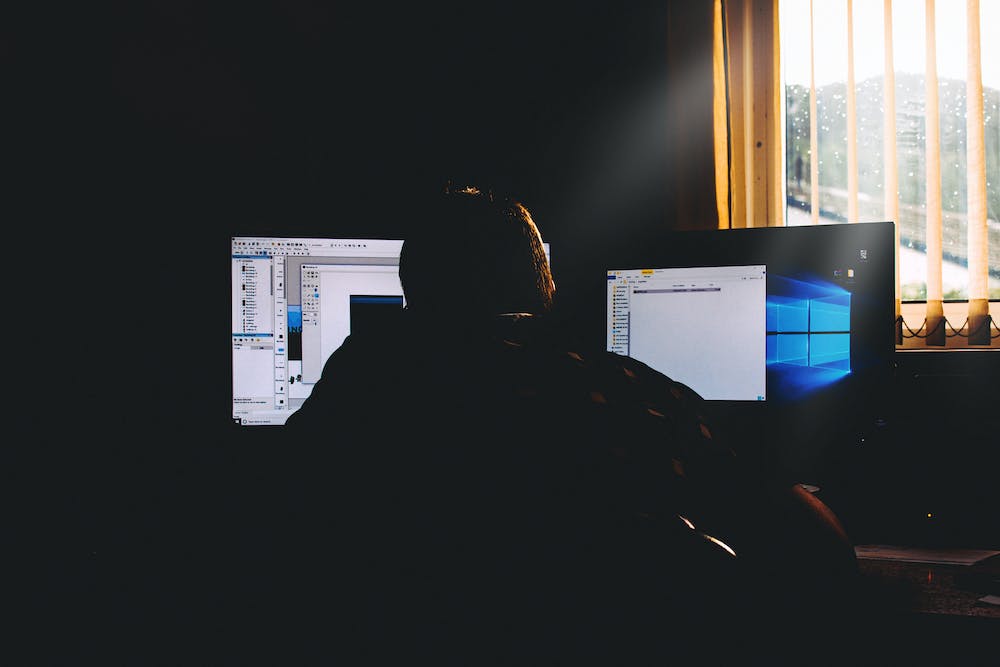
Beginner’s Guide to Node.js: Getting Started with JavaScript Backend Development
Introduction
Node.js has emerged as a popular runtime environment for server-side JavaScript development. IT allows developers to build scalable and efficient web applications using JavaScript on the backend. If you’re new to Node.js and want to explore the world of backend development with JavaScript, you’ve come to the right place.
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment built on Chrome’s V8 JavaScript engine. IT allows developers to execute JavaScript code outside the browser, making IT ideal for server-side and networking applications. With Node.js, you can build fast, scalable, and event-driven web applications.
Setting up Node.js
To get started with Node.js development, you first need to install Node.js on your machine. Visit the official Node.js Website (https://nodejs.org) and download the appropriate installer for your operating system. Follow the installation instructions, and you’ll be ready to start coding in no time.
Basic Building Blocks
Once you have Node.js installed, IT‘s time to get familiar with its basic building blocks:
1. Modules
Modules are reusable pieces of code that can be loaded into your application using the ‘require’ keyword. Node.js provides a vast ecosystem of modules that you can easily integrate into your project. You can also create your own modules and share them with the community.
2. NPM (Node Package Manager)
NPM is the default package manager for Node.js. IT allows you to manage dependencies, install modules, and publish your own packages. With NPM, you can easily add functionality to your application by installing pre-existing modules.
3. Asynchronous Programming
Node.js is designed to handle a large number of simultaneous connections efficiently. IT achieves this by using asynchronous programming, which allows multiple operations to be executed concurrently. This makes Node.js ideal for building real-time applications, chat servers, and streaming applications.
Creating Your First Node.js Application
Now that you have a good understanding of the basic building blocks of Node.js, let’s create your first Node.js application:
1. Initialize Your Project
Open your terminal or command prompt and navigate to the desired directory where you want to create your application. Run the following command to create a new Node.js project:
$ npm init
2. Install Dependencies
To install the necessary dependencies for your project, you can use the ‘npm install’ command followed by the name of the package. For example, to install the Express framework, run:
$ npm install express
3. Create Your Application File
Create a new file with a ‘.js’ extension and add your JavaScript code. For example, let’s create a file called ‘app.js’ and add the following code:
// Import the Express module
const express = require('express');
// Create an Express application
const app = express();
// Define a route
app.get('/', (req, res) => {
res.send('Hello, World!');
});
// Start the server
app.listen(3000, () => {
console.log('Server started on port 3000');
});
4. Run Your Application
To start your Node.js application, simply run the following command:
$ node app.js
Conclusion
Congratulations! You’ve completed the beginner’s guide to Node.js. You’ve learned what Node.js is, how to set IT up, and how to create your first Node.js application. With Node.js, you can leverage your JavaScript skills to build powerful and efficient backend solutions and join the thriving Node.js community of developers.
FAQs
Q: Can I use Node.js for frontend development?
A: No, Node.js is primarily used for server-side development. However, you can use frameworks like React or Angular for frontend development and combine them with Node.js on the backend.
Q: Is Node.js suitable for large-scale applications?
A: Yes, Node.js is scalable and can handle heavy traffic due to its non-blocking architecture. Many large companies, including Netflix and Uber, use Node.js for their backend systems.
Q: Does Node.js support database connectivity?
A: Yes, Node.js has excellent support for interacting with various databases. There are libraries available that allow you to connect to databases like MongoDB, MySQL, and PostgreSQL.
Q: Can I deploy Node.js applications on cloud platforms?
A: Yes, Node.js is widely supported by cloud platforms like AWS, Microsoft Azure, and Google Cloud. You can easily deploy and scale your Node.js applications on these platforms.
Q: Is Node.js only for JavaScript developers?
A: No, while having JavaScript knowledge helps, you can learn Node.js even if you’re not familiar with JavaScript. However, knowing JavaScript will give you a head start in understanding Node.js concepts.