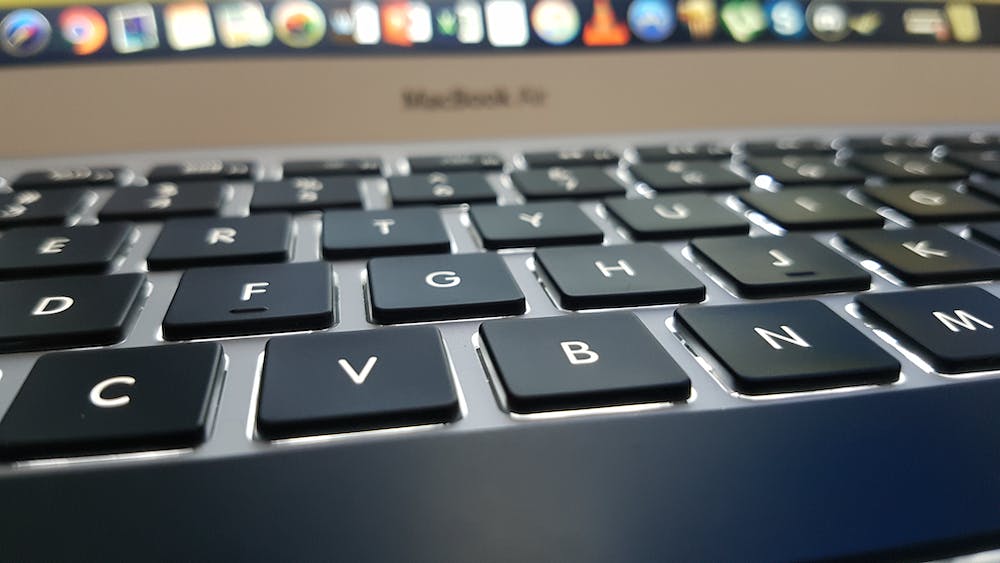
PHP is a popular scripting language that is widely used for web development. Whether you are a beginner who wants to learn PHP or an experienced programmer looking to expand your skillset, this article will provide a comprehensive guide to getting started with the basics of PHP.
Before diving into PHP, IT is essential to have a basic knowledge of HTML and CSS as they form the foundation of web development. Understanding these technologies will help you grasp PHP concepts more effectively.
Now, let’s get started with the fundamentals of PHP:
1. Installing PHP: To begin coding in PHP, you need to have PHP installed on your machine. PHP is a server-side language and runs on web servers such as Apache or Nginx. You can easily find installation instructions for your specific operating system on the official PHP Website (https://www.php.net/manual/en/install.php).
2. writing PHP Code: PHP code is typically embedded within HTML files. To execute PHP code, you need to enclose IT within <?php and ?> tags. For example:
<?php
echo “Hello, World!”;
?>
This code will output “Hello, World!” on the web page. The echo
statement is used to display text or variables.
3. Variables and Data Types: PHP supports various data types, including strings, integers, floats, booleans, arrays, and objects. Variables are used to store and manipulate data. For example:
<?php
$name = “John”;
$age = 25;
echo “My name is ” . $name . ” and I am ” . $age . ” years old.”;
?>
The above code will output “My name is John and I am 25 years old.”
4. Control Structures: Control structures allow you to make decisions and control the flow of your program. PHP provides if-else statements and loops to achieve this. Here’s an example:
<?php
$num = 10;
if ($num > 0) {
echo “The number is positive.”;
} else {
echo “The number is negative.”;
}
?>
The output will be “The number is positive” since the condition ($num > 0) is true.
5. Functions: Functions in PHP are reusable blocks of code that perform specific tasks. They help in organizing your code and making IT more modular. Here’s an example:
<?php
function sayHello($name) {
echo “Hello, ” . $name . “!”;
}
sayHello(“Alice”);
?>
This code will output “Hello, Alice!”. The function sayHello
takes a parameter $name
and displays a greeting using that name.
FAQs:
Q: Can PHP be used for creating dynamic web pages?
A: Yes, PHP is commonly used for creating dynamic web pages. IT allows embedding PHP code within HTML, enabling developers to generate dynamic content based on various factors such as user input, database queries, and more.
Q: Does PHP work on all operating systems?
A: PHP is compatible with most operating systems, including Windows, Linux, macOS, and Unix-based systems.
Q: Can I learn PHP without any programming experience?
A: While prior programming experience can certainly be helpful, PHP is considered relatively easy to learn for beginners. IT has a gentle learning curve and offers extensive documentation and community support.
Q: Is PHP still relevant in the modern web development landscape?
A: Despite the emergence of new programming languages and frameworks, PHP remains a popular choice for web development. IT powers multiple popular content management systems (CMS) like WordPress, Drupal, and Joomla.
Q: How can I enhance my PHP skills?
A: To enhance your PHP skills, consider participating in online forums and communities, exploring advanced PHP concepts, working on PHP projects, and reading books or tutorials dedicated to PHP programming.
By now, you should have a solid foundation in PHP basics. Remember, practice is crucial to mastering any programming language. So, start coding and building projects to strengthen your PHP skills!