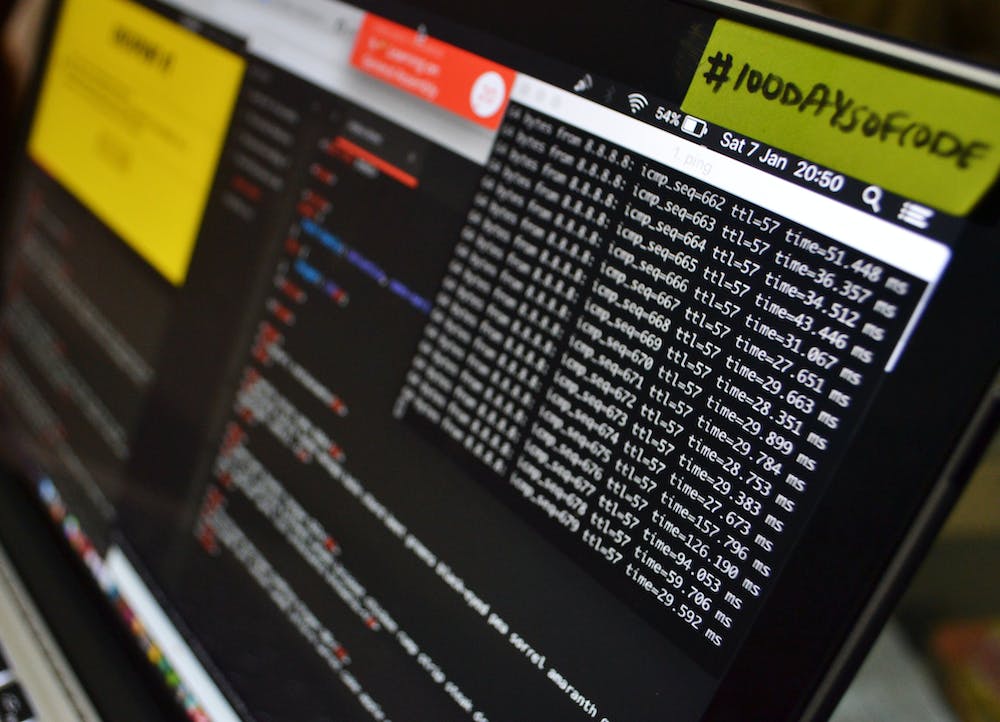
Beginner’s Guide to JavaScript: Getting Started
Introduction
JavaScript is a popular and powerful programming language that is essential for building dynamic and interactive websites. Whether you are a beginner or have some experience with other programming languages, this guide will help you get started with JavaScript. In this article, we will cover the basics of JavaScript, including its syntax, variables, data types, operators, and functions. So, let’s dive into the world of JavaScript and get started!
Understanding JavaScript
JavaScript is a scripting language that is primarily used to add interactivity and behavior to web pages. IT allows you to create dynamic Website elements, handle user interactions, validate forms, and much more. In recent years, JavaScript has become an essential language for both front-end and back-end web development.
Setting Up Your Development Environment
Before you can start coding in JavaScript, you need to set up your development environment. All you need is a text editor and a web browser. You can use any text editor of your choice, such as Visual Studio Code, Sublime Text, or Atom. The web browser will be used to test your JavaScript code.
writing Your First JavaScript Code
Let’s start by writing a simple JavaScript code that displays a message in an alert box. Open your text editor and create a new file with the extension “.html”. Add the following code to your HTML file:
“`html
“`
Save the file and open IT in a web browser. You will see an alert box displaying the message “Hello, JavaScript!”. Congratulations! You have written your first JavaScript code. Now let’s explore some fundamental concepts in JavaScript.
JavaScript Basics
Syntax
JavaScript code is written in plain text and uses a specific syntax. Each statement in JavaScript should end with a semicolon (;). Let’s take a look at a simple JavaScript statement:
“`javascript
console.log(“Hello, World!”);
“`
The “`console.log()“` function is used to print messages to the browser’s console. Open the browser’s developer console (usually by pressing F12) and run the code above. You should see the message “Hello, World!” displayed in the console.
Variables
In JavaScript, variables are used to store data. They are declared using the “`var“`, “`let“`, or “`const“` keywords. Here’s an example:
“`javascript
var greeting = “Hello, JavaScript!”;
console.log(greeting);
“`
The variable “`greeting“` is assigned the value “Hello, JavaScript!” and then printed to the console. You can change the value of a variable at any time. However, variables declared with “`const“` cannot be reassigned.
Data Types
JavaScript has several data types, including strings, numbers, booleans, arrays, and objects. Here are a few examples:
“`javascript
var name = “John”;
var age = 25;
var isStudent = true;
var hobbies = [“reading”, “painting”, “coding”];
var person = {
name: “John”,
age: 25
};
“`
You can use the “`typeof“` operator to check the data type of a variable. For example:
“`javascript
console.log(typeof name); // Output: string
console.log(typeof age); // Output: number
console.log(typeof isStudent); // Output: boolean
console.log(typeof hobbies); // Output: object
console.log(typeof person); // Output: object
“`
Operators
JavaScript provides various operators to perform operations on variables and values. Here are some commonly used operators:
- Arithmetic Operators: “`+“`, “`-“`, “`*“`, “`/“`, “`%“`
- Comparison Operators: “`==“`, “`!=“`, “`>“`, “`<```, ```>=“`, “`<=```
- Logical Operators: “`&&“` (AND), “`||“` (OR), “`!“` (NOT)
- Assignment Operators: “`=“`, “`+=“`, “`-=“`, “`*=“`, “`/=“`
Here’s an example that demonstrates the use of operators:
“`javascript
var x = 10;
var y = 5;
console.log(x + y); // Output: 15
console.log(x > y); // Output: true
console.log(!(x == y)); // Output: true
x += 5;
console.log(x); // Output: 15
“`
Functions
Functions are reusable blocks of code that perform a specific task. They allow you to divide your code into smaller, manageable chunks. Here’s a simple function in JavaScript:
“`javascript
function sayHello() {
console.log(“Hello, JavaScript!”);
}
sayHello(); // Output: Hello, JavaScript!
“`
You can pass arguments to functions and return values using the “`return“` keyword. Functions make your code more organized, easier to read, and reusable.
Conclusion
Congratulations on completing the beginner’s guide to JavaScript! In this article, we covered the basics of JavaScript, including its syntax, variables, data types, operators, and functions. With this knowledge in hand, you can start exploring more advanced concepts, such as objects, arrays, and DOM manipulation. JavaScript is a versatile language that is continuously evolving, so keep practicing and experimenting with different coding challenges to strengthen your skills.
FAQs
Q: Can I run JavaScript code directly in my browser?
A: Yes, you can run JavaScript code directly in your browser by adding IT to an HTML file inside a script tag. You can also use the browser’s developer console to execute JavaScript code.
Q: What is the difference between “`var“`, “`let“`, and “`const“` in JavaScript?
A: “`var“` is function-scoped, while “`let“` and “`const“` are block-scoped. The value of a “`let“` variable can be reassigned, but a “`const“` variable cannot be changed once assigned.
Q: How can I learn more about JavaScript?
A: There are several resources available to learn JavaScript, including online tutorials, documentation, and interactive coding platforms. You can also consider taking an online course or joining a coding bootcamp to accelerate your learning process.
Q: Is JavaScript the same as Java?
A: No, JavaScript and Java are two different programming languages. JavaScript is primarily used for web development, while Java is a general-purpose programming language used for various applications, including desktop, mobile, and web development.