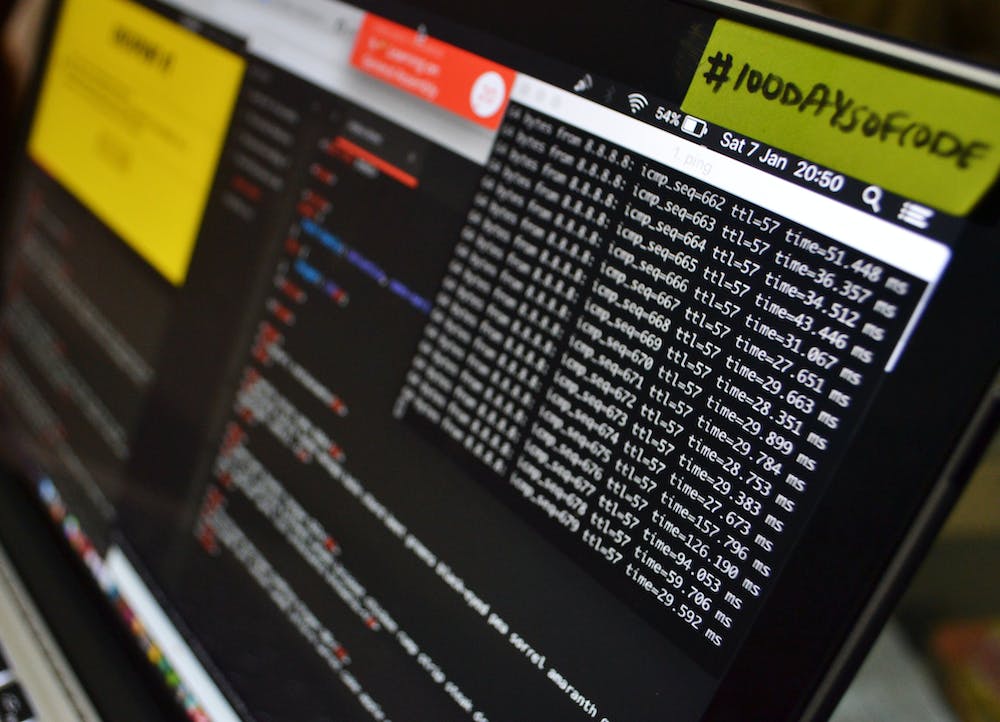
Beginner’s Guide to Downloading Files with JavaScript
Introduction
Downloading files using JavaScript is a common requirement for many web applications. By utilizing the power of JavaScript, you can provide your users with a seamless download experience, enhancing the interaction with your Website. In this article, we will guide you through the basics of downloading files with JavaScript, including various techniques and best practices.
Table of Contents
Basic File Download
One of the simplest ways to initiate a file download in JavaScript is by using the `anchor` element’s `download` attribute. This attribute allows you to specify the name of the file that will be downloaded when the user clicks on the link.
Example:
<a href="path/to/file.pdf" download="my_file.pdf">Download PDF</a>
When the user clicks on the link, the file specified in the `href` attribute will be downloaded with the name provided in the `download` attribute.
Using Blob URLs
Another powerful technique for downloading files with JavaScript is by using Blob URLs. Blobs (Binary Large Objects) allow you to represent data or files as immutable byte sequences. To initiate a file download using Blob URLs, follow these steps:
- Create a Blob object from the file data.
- Create a temporary URL using the `URL.createObjectURL()` method.
- Create an `anchor` element with the temporary URL and a `download` attribute.
- Programmatically click on the `anchor` element to trigger the download.
Example:
function downloadFile(fileData, fileName) {
const blob = new Blob([fileData]);
const url = URL.createObjectURL(blob);
const downloadLink = document.createElement('a');
downloadLink.href = url;
downloadLink.download = fileName;
document.body.appendChild(downloadLink);
downloadLink.click();
document.body.removeChild(downloadLink);
}
With this function, you can pass the file data as an array or an ArrayBuffer and provide a desired file name. This will create a Blob, generate a temporary URL, create an `anchor` element, simulate a click on IT to initiate the download, and finally remove the temporary `anchor` element.
Third-Party Libraries
While the above techniques are more than sufficient for basic file downloads, there are several third-party JavaScript libraries available that can simplify and enhance your file download implementation. These libraries often provide additional features, such as progress tracking, error handling, and more.
Some popular libraries for file downloads in JavaScript include:
These libraries are well-documented with examples and suitable for various use cases. Consider exploring them to determine if they fit your specific requirements.
Advantages and Considerations
Downloading files with JavaScript offers several advantages:
- Enhanced user experience: Users can download files directly from your Website without being redirected to a new page or losing their current context.
- Dynamic file generation: JavaScript allows you to generate files on the fly, providing the ability to download dynamically created content.
- Easy integration: File downloads can be seamlessly integrated into existing web applications, providing a streamlined experience for users.
However, IT is important to consider the following:
- Browser support: Not all browsers fully support the `download` attribute or Blob URLs, so IT is essential to verify the compatibility with your target audience.
- Security concerns: Be cautious when allowing users to download files directly from your Website, as IT may expose your server to potential security risks. Validate user inputs and implement proper security measures.
Conclusion
In this article, we explored the basics of downloading files with JavaScript, covering the traditional method using the `download` attribute and the more powerful approach with Blob URLs. We also mentioned a few popular third-party libraries that can simplify your file download implementation.
Remember to consider browser compatibility and security concerns when implementing file downloads in your web applications. By providing a seamless download experience, you can enhance user satisfaction and make your Website more interactive.
FAQs
Can I trigger a file download without user interaction?
No, most modern browsers require user interaction, such as a click, to initiate a file download for security reasons. This prevents malicious websites from automatically downloading files without the user’s knowledge or consent.
What file formats can be downloaded using JavaScript?
JavaScript can be used to download various file formats, including but not limited to PDF, images (JPG, PNG, etc.), text files (TXT, CSV), and multimedia files (MP3, MP4).
Are there any restrictions on file sizes that can be downloaded with JavaScript?
Both the limitations imposed by the browser and the server hosting the file can affect the maximum file size that can be downloaded. IT is recommended to check the restrictions of your browser and server to ensure a smooth downloading experience for your users.
Can I track the progress of a file download using JavaScript?
Yes, some third-party libraries, like FileSaver.js and Download.js, provide functionality to track the progress of file downloads. These libraries often offer additional features, such as the ability to pause and resume downloads.
Is IT possible to download multiple files simultaneously with JavaScript?
Yes, IT is possible to initiate multiple file downloads simultaneously by generating and triggering multiple download links programmatically.
Can I style the download button or link?
Yes, you can apply custom CSS styles to the download button or link to match the design of your Website or application.