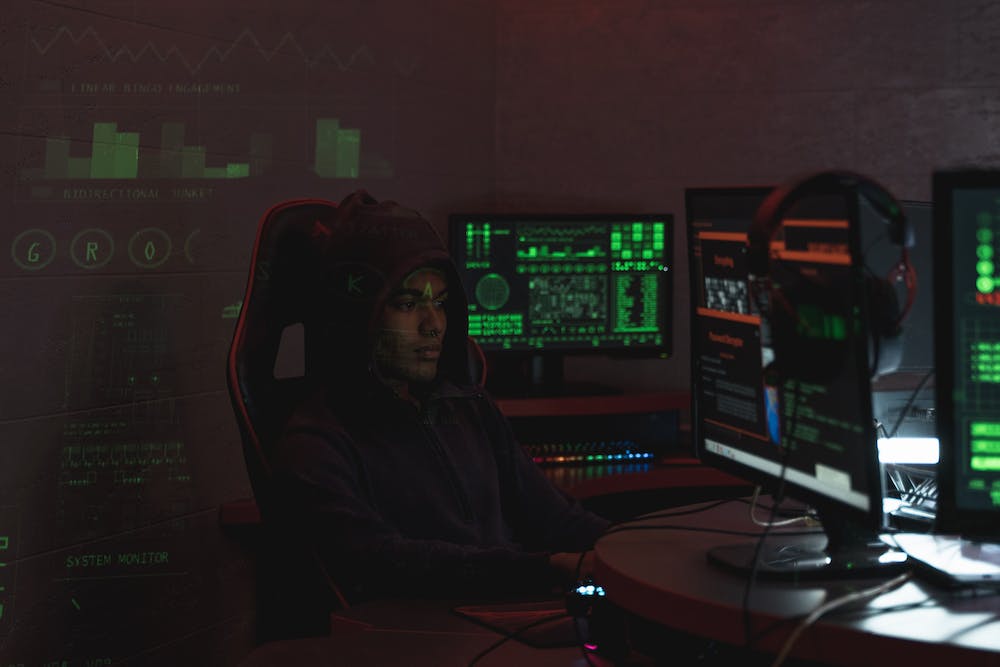
As a programmer, writing clean, readable, and well-structured code is crucial for the success and maintainability of your projects. One way to achieve this is by following a set of coding style guidelines. One such widely used guideline is “PEP 8,” which stands for “Python Enhancement Proposal 8.”
What is PEP 8?
PEP 8 is a style guide for Python code that provides recommendations on how to format and structure your code. IT covers areas such as indentation, line length, naming conventions, import statements, and much more. By adhering to these guidelines, your code will be more readable, consistent, and easier to understand for both yourself and others.
Why is PEP 8 important?
Consistency is essential when working on collaborative projects or when reviewing your own code. PEP 8 helps achieve this consistency by providing a standardized set of rules that everyone can follow. By following these guidelines, you make your code more understandable, which can save time and effort when debugging, maintaining, or extending your codebase.
The Ultimate Online Pep8 Guide
Are you interested in learning how to apply PEP 8 guidelines to your code? Look no further! We have created the ultimate online PEP 8 guide to help you unleash your code’s superpower.
1. Indentation and Line Length
One important aspect of PEP 8 is the proper use of indentation and line length. Following these guidelines will make your code more readable and organized. For example:
# Good indentation
def my_function():
if condition:
print("Indented properly!")
# Bad indentation
def my_function():
if condition:
print("Indented improperly!")
In addition, PEP 8 suggests keeping lines within a maximum limit of 79 characters. If a line exceeds this limit, IT should be split into multiple lines.
2. Naming Conventions
PEP 8 recommends using specific naming conventions for variables, functions, and classes. For example:
# Good naming convention
def calculate_average(numbers):
total = sum(numbers)
return total / len(numbers)
# Bad naming convention
def calcAvg(nums):
sumOfNums = sum(nums)
return sumOfNums / len(nums)
Using descriptive names and adhering to proper naming conventions makes your code more readable and helps others understand your code better.
3. Import Statements
PEP 8 provides guidelines for organizing and formatting import statements. IT suggests grouping and ordering imports in a specific way. For example:
# Good import statements
import os
import sys
from datetime import datetime
# Bad import statements
import sys, os
from datetime import datetime, timedelta
By following these recommendations, you can improve the readability and clarity of your code.
Conclusion
Using PEP 8 guidelines can significantly enhance the quality of your code. IT makes your code more readable, consistent, and maintainable. By understanding and applying these guidelines, you can improve collaboration in programming projects and elevate the overall standard of code within the community.
FAQs
Q: Are the PEP 8 guidelines only applicable to Python?
A: No, while PEP 8 is specifically designed for Python code, the concepts behind IT can be applied to other programming languages as well. Each language might have its own coding style conventions, so make sure to research and adhere to the appropriate guidelines for the language you are using.
Q: Can I use automated tools to check if my code complies with PEP 8 guidelines?
A: Yes, there are various tools available that can help you automatically analyze your code for PEP 8 compliance. Some popular tools include pylint, flake8, and pycodestyle. These tools can save you time and effort by identifying violations and suggesting improvements.
Q: What if I disagree with some of the PEP 8 guidelines?
A: PEP 8 guidelines are recommendations, and IT is possible to have different opinions on some aspects. However, IT‘s generally a good practice to stick to the guidelines to ensure consistency and readability among your codebase. Consistency within a project is crucial, so if you’re working with a team, IT‘s advisable to reach a consensus and follow a unified coding style to avoid confusion.