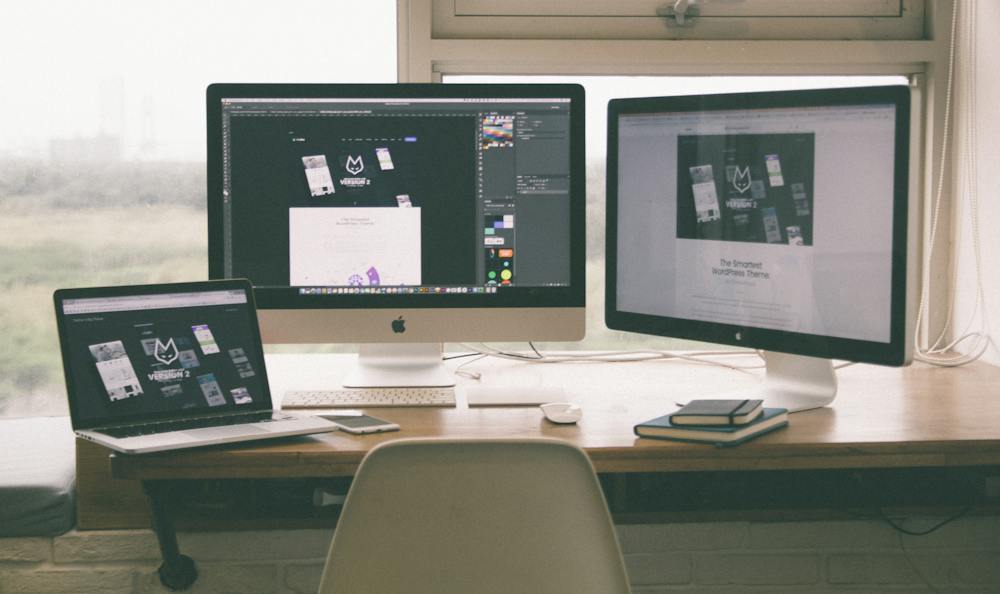
JavaScript is a powerful and versatile programming language that is widely used in web development. One of the many things that can be accomplished with JavaScript is getting the current year. In this article, we will explore different methods of getting the current year using JavaScript, and you may be surprised at how easy IT is!
Method 1: Using the Date Object
The Date object in JavaScript provides a convenient way to work with dates and times. To get the current year using the Date object, you can simply create a new Date object and then use the getFullYear() method to retrieve the current year.
const currentDate = new Date();
const currentYear = currentDate.getFullYear();
console.log(currentYear);
When you run the above code, it will output the current year to the console. This method is simple and straightforward, making it a popular choice for getting the current year in JavaScript.
Method 2: Using the Intl Object
The Intl object in JavaScript provides a way to perform internationalization and localization operations, including getting the current year in a locale-specific format. You can use the DateTimeFormat object within the Intl object to achieve this.
const currentYear = new Intl.DateTimeFormat('en', {year: 'numeric'}).format(new Date());
console.log(currentYear);
With the above code, you can get the current year in the “en” locale format, which stands for English. You can replace “en” with other locale codes to get the current year in different formats. This method is useful for applications that require displaying the current year in a specific locale.
Method 3: Using the UTC Methods
If you need to work with dates and times in UTC format, JavaScript provides the getUTCFullYear() method to get the current year in UTC. This method returns the four-digit year as a number, which can be useful for handling date and time calculations in a consistent manner.
const currentDateUTC = new Date();
const currentYearUTC = currentDateUTC.getUTCFullYear();
console.log(currentYearUTC);
By using the getUTCFullYear() method, you can ensure that the current year is obtained in the UTC time zone, regardless of the local time zone of the user’s device. This can be essential for global applications and websites that need to work with standardized date and time values.
Conclusion
In conclusion, getting the current year using JavaScript is incredibly easy and straightforward. Whether you need the current year for display purposes, date calculations, or internationalization, JavaScript provides a variety of methods to accomplish this task. By using the Date object, Intl object, or UTC methods, you can retrieve the current year with just a few lines of code.
FAQs
Q: Are there any limitations to using JavaScript to get the current year?
A: While JavaScript provides convenient methods for getting the current year, it’s important to be aware of potential issues with date and time manipulation, such as time zones, daylight saving time, and leap years. It’s recommended to use reputable libraries or built-in language features to handle complex date and time operations.
Q: Can I use the current year in JavaScript for SEO purposes?
A: Using the current year in JavaScript for SEO (Search Engine Optimization) purposes can be beneficial for dynamic content that needs to be updated regularly. For example, displaying the current year in the footer of a Website can indicate to search engines that the content is up-to-date. However, it’s essential to ensure that the current year is implemented correctly to avoid SEO penalties.
Q: Is it recommended to use external libraries for date and time operations in JavaScript?
A: Depending on the complexity of your date and time requirements, using reputable date and time libraries in JavaScript, such as Moment.js or Luxon, can provide additional features and robustness for handling date and time operations. It’s important to evaluate the specific needs of your application and choose the appropriate tools for working with dates and times.