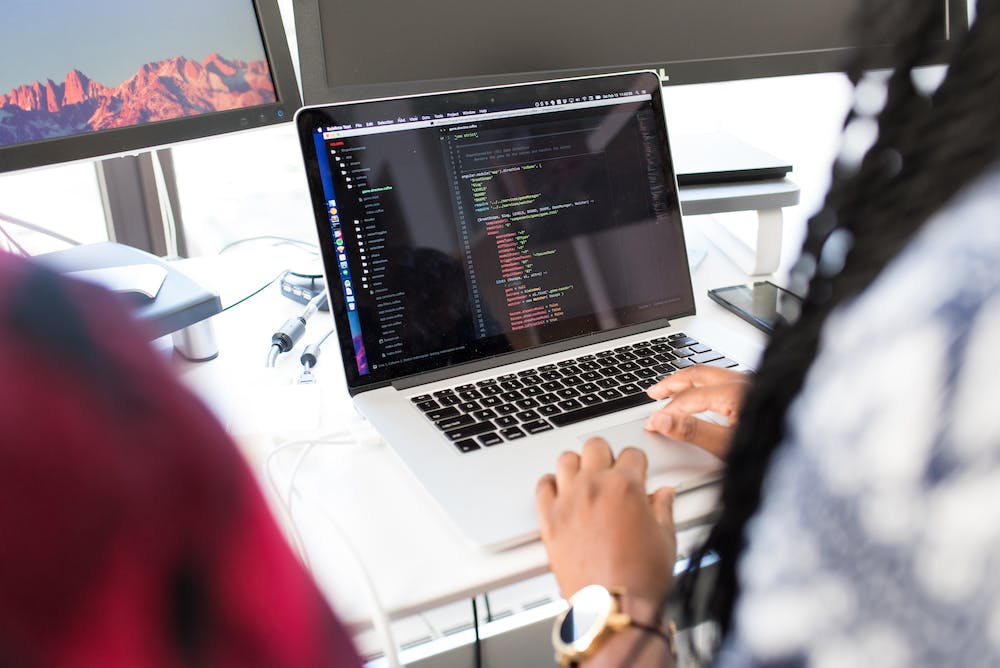
PHP multidimensional arrays are a powerful tool for storing and manipulating complex data structures. However, many developers make a common mistake when working with multidimensional arrays that can lead to inefficient code and unnecessary complexity. In this article, we’ll explore this common mistake and provide a surprising solution that can improve the performance and readability of your PHP code.
The Common Mistake
The common mistake that many developers make when working with PHP multidimensional arrays is using nested loops to access and manipulate the data. While nested loops can certainly get the job done, they often result in redundant and hard-to-read code. Consider the following example:
$users = array(
array("id" => 1, "name" => "John", "age" => 25),
array("id" => 2, "name" => "Jane", "age" => 30),
array("id" => 3, "name" => "Bob", "age" => 28)
);
foreach ($users as $user) {
foreach ($user as $key => $value) {
echo $key . ': ' . $value . '<br/>';
}
}
In this example, we have a multidimensional array representing user data. We use nested foreach loops to iterate over the outer array and then iterate over the inner arrays to access the individual key-value pairs. While this approach works, IT‘s not the most efficient or readable way to work with multidimensional arrays in PHP.
The Surprising Solution
The surprising solution to this common mistake is to use PHP’s array functions to work with multidimensional arrays. PHP provides a set of powerful array functions that can simplify and streamline the manipulation of multidimensional arrays. One such function is array_map(), which applies a callback function to each element of an array and returns a new array with the modified elements.
Using array_map() allows us to avoid nested loops and achieve the same result with cleaner and more concise code. Here’s how we can rewrite the previous example using array_map():
function printKeyValue($item) {
foreach ($item as $key => $value) {
echo $key . ': ' . $value . '<br/>';
}
}
array_map('printKeyValue', $users);
In this example, we define a callback function printKeyValue() that takes an array as its argument and prints out the key-value pairs. We then use array_map() to apply this function to each element of the $users array, effectively achieving the same result as the nested foreach loops but with much cleaner code.
Benefits of Using Array Functions
Using array functions to work with multidimensional arrays in PHP offers several benefits:
- Improved readability: Array functions can make your code more concise and easier to understand, reducing the need for nested loops and conditional statements.
- Performance improvements: Array functions are often more efficient than nested loops, resulting in faster execution times for your code.
- Code reusability: By encapsulating array manipulation logic in callback functions, you can reuse the same logic in multiple places in your code, promoting a more modular and maintainable codebase.
Conclusion
Working with multidimensional arrays in PHP doesn’t have to be complicated or inefficient. By leveraging PHP’s array functions, you can simplify your code and improve its performance while maintaining readability and reusability. The surprising solution of using array functions instead of nested loops can lead to more elegant and maintainable code that will benefit both you and your fellow developers.
FAQs
Q: Can I use array functions with associative arrays?
A: Yes, array functions can be used with both indexed and associative arrays. The key is to define callback functions that work with the specific structure of your multidimensional arrays.
Q: Are there any performance trade-offs when using array functions?
A: In general, array functions offer performance improvements over nested loops due to their internal optimizations. However, as with any optimization, it’s important to measure and profile your code to ensure that the benefits outweigh any potential trade-offs.
Q: Can I combine array functions with other PHP features, such as closures and anonymous functions?
A: Absolutely! PHP’s array functions can be combined with closures and anonymous functions to create powerful and flexible code that can handle a wide range of use cases.
Q: Are there any resources or tutorials for learning more about array functions in PHP?
A: Yes, there are many resources available online for learning more about array functions in PHP. backlink works offers a comprehensive guide to PHP array functions that can help you master these powerful tools.