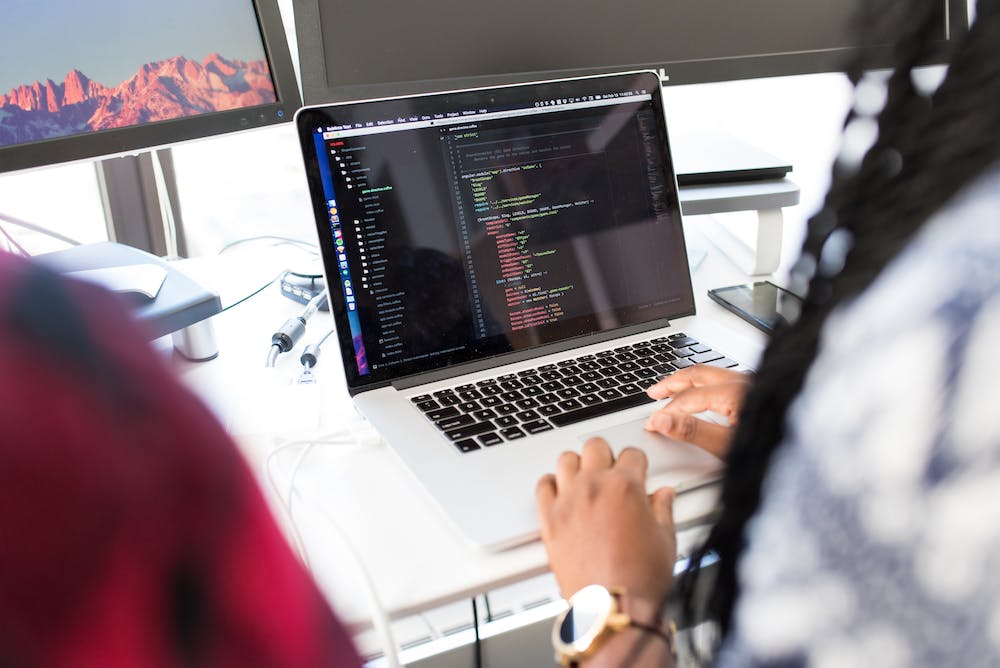
PHP fopen is a powerful function that allows developers to open and manipulate files in PHP. IT provides a simple and intuitive way to read, write, and modify files on a server. This flexibility has made fopen one of the most commonly used functions in PHP programming. In this article, we will explore the different modes of fopen, how to use IT to read and write files, and some frequently asked questions about this function.
fopen Modes
The fopen function can be used to open a file in a variety of modes, depending on the desired functionality. The most commonly used modes are:
- r: Opens the file for reading only. The file pointer is positioned at the beginning of the file.
- w: Opens the file for writing only. If the file does not exist, IT creates a new file. If the file exists, IT truncates the file to zero length.
- a: Opens the file for writing only. If the file does not exist, IT creates a new file. If the file exists, IT appends the new data to the end of the file.
- x: Creates and opens the file for writing only. Returns false if the file already exists.
Opening a File
To open a file using the fopen function, you need to provide the filename and the mode as parameters. For example, to open a file named “example.txt” in read-only mode:
<?php
$file = fopen("example.txt", "r");
?>
This code snippet opens the “example.txt” file and returns a resource variable, $file
, which can be used to perform various operations on the file.
Reading a File
Once a file is open, you can use various methods to read its contents. The most commonly used method is fread
, which allows you to read a specified number of bytes from the file.
<?php
$file = fopen("example.txt", "r");
$data = fread($file, filesize("example.txt"));
echo $data;
fclose($file);
?>
This code opens the “example.txt” file, reads its contents using fread
, and stores the data in the variable $data
. Finally, IT echoes the contents of the file and closes the file using fclose
.
writing to a File
To write to a file, you need to open IT in write-only mode or append mode. If the file does not exist, fopen creates a new file for you. Here’s an example:
<?php
$file = fopen("example.txt", "w");
$data = "Hello, World!";
fwrite($file, $data);
fclose($file);
?>
In this example, the code opens the “example.txt” file in write-only mode, writes the string “Hello, World!” using fwrite
, and closes the file. If the file already exists, IT will be overwritten with the new data.
FAQs about PHP fopen
Q: Can fopen be used to open remote files?
A: Yes, fopen can be used to open remote files if the allow_url_fopen directive is enabled in the server’s php.ini file.
Q: Can fopen handle binary files?
A: Yes, fopen can handle both binary and text files. You just need to specify the appropriate mode (“rb” for binary, “rt” for text).
Q: What happens if you try to open a non-existent file in read-only mode?
A: If you try to open a non-existent file in read-only mode, fopen will return false, indicating that the file could not be opened.
Q: How can I check if a file exists before opening IT?
A: You can use the file_exists function to check if a file exists before opening IT with fopen.
Q: Is IT important to close files after opening them with fopen?
A: Yes, IT is good practice to close files after opening them with fopen. Not closing files can lead to memory leaks and other issues.
To conclude, PHP’s fopen function provides a versatile and efficient way to open and manipulate files. Whether you need to read, write, or append data, fopen has you covered. By understanding the different modes and methods available, you can harness the full potential of this function in your PHP projects.