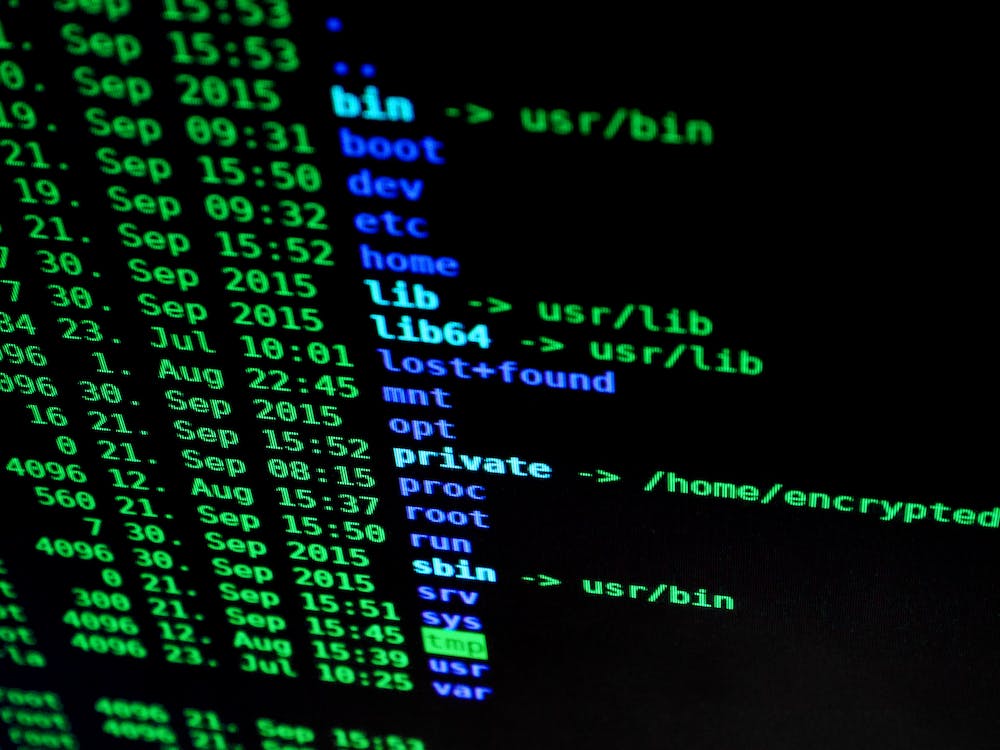
An Introduction to PHP Explode Function
PHP is a popular programming language that is widely used for web development. One of the most powerful features of PHP is its string manipulation functions. In this article, we will explore the PHP explode function and understand its usage.
What is the PHP Explode Function?
The explode function in PHP is used to split a string into an array based on a delimiter. IT takes two parameters – the delimiter and the input string. The delimiter specifies where the string should be split, and the input string is the string to be split.
Let’s take an example:
“`php
$input = “Hello, World!”;
$array = explode(“,”, $input);
“`
In this example, we have a string “Hello, World!” and we want to split IT at the comma separator. The explode function takes the delimiter (“,”) and the input string (“Hello, World!”) as parameters. IT then returns an array with the elements “Hello” and ” World!”.
Usage of the PHP Explode Function
The explode function has a wide range of applications in web development. Here are a few common use cases:
1. Parsing CSV Data
CSV (Comma-Separated Values) is a popular file format for storing tabular data. The explode function can be used to parse CSV data by splitting the string at the comma delimiter. Each element of the resulting array represents a data field.
“`php
$data = “John,Doe,25,New York”;
$fields = explode(“,”, $data);
“`
In this example, the string “John,Doe,25,New York” represents a CSV row with four fields – name, last name, age, and city. The explode function splits the string at each comma, resulting in an array containing the individual fields.
2. Breaking Down URL Parameters
In web development, URLs often contain query parameters. The explode function can be used to split the URL and extract the individual parameters.
“`php
$url = “https://example.com/search?q=php&tutorial=true”;
$parameters = explode(“?”, $url)[1];
$queryParams = explode(“&”, $parameters);
“`
In this example, we have a URL with query parameters – “q=php&tutorial=true”. The explode function is first used to split the URL at the question mark delimiter, resulting in an array with two elements – the base URL and the query parameters. Then, another explode function splits the query parameters into individual key-value pairs.
3. Tokenizing Strings
The explode function can be used to tokenize strings by splitting them based on specific characters. This is often used in text processing to separate words or sentences.
“`php
$text = “This is a sample sentence.”;
$words = explode(” “, $text);
“`
In this example, the string “This is a sample sentence.” is split into an array of words using the space character as a delimiter. The resulting array contains the individual words: “This”, “is”, “a”, “sample”, and “sentence”.
Conclusion
The PHP explode function is a powerful tool for splitting strings into arrays based on delimiters. IT has various applications in web development, such as parsing CSV data, breaking down URL parameters, and tokenizing strings. Understanding how to use this function can greatly enhance your ability to manipulate and process strings in PHP.
FAQs
Q: Can the explode function handle multiple delimiters?
A: No, the explode function in PHP can only handle a single delimiter at a time. If you need to split a string based on multiple delimiters, you can use a combination of string functions or regular expressions.
Q: Is the explode function case-sensitive?
A: Yes, the explode function in PHP is case-sensitive. This means that the delimiter must exactly match the characters in the input string for a successful split.
Q: What happens if the delimiter is not found in the input string?
A: If the delimiter is not found in the input string, the explode function will return an array with a single element containing the original string.
Q: Can I use characters other than commas or spaces as delimiters?
A: Yes, you can use any character as a delimiter in the explode function, including special characters. However, IT is important to choose a delimiter that is not present in the input string to ensure accurate splitting.
Q: Is the explode function available in all versions of PHP?
A: Yes, the explode function is a built-in function in PHP and is available in all versions of PHP.