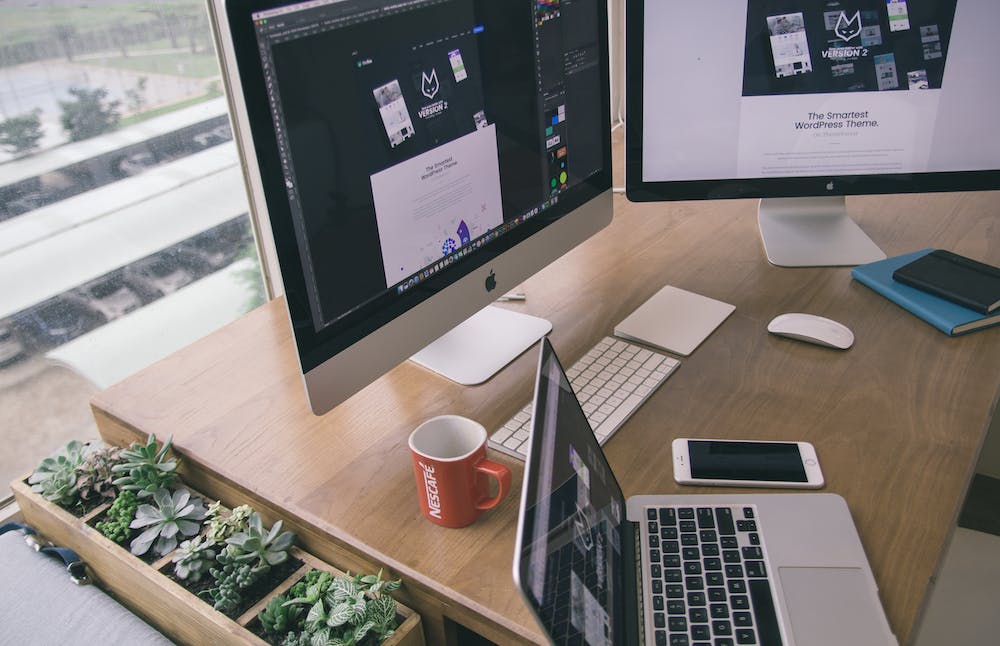
PHP is one of the most widely-used programming languages, especially for web development. One of its key features is the ability to work with strings, which are a fundamental part of any web application. In this article, we will explore everything you need to know about PHP string functions, including their usage, examples, and best practices.
What are PHP String Functions?
PHP string functions are built-in functions that allow developers to manipulate and work with strings in PHP. These functions provide a wide range of capabilities, including string creation, manipulation, and analysis. They can be used to extract substrings, find and replace text, convert case, and much more.
Commonly Used PHP String Functions
There are numerous string functions available in PHP. Some of the most commonly used ones include:
- strlen() – Returns the length of a string
- str_word_count() – Counts the number of words in a string
- str_replace() – Replaces all occurrences of a search string with a replacement string
- strtolower() – Converts a string to lowercase
- strtoupper() – Converts a string to uppercase
- substr() – Returns a part of a string
Usage and Examples
Let’s take a look at some examples of how these string functions can be used in PHP:
Example 1: strlen()
The strlen()
function returns the length of a string. Here’s an example:
$str = "Hello, World!";
echo strlen($str); // Outputs: 13
Example 2: str_replace()
The str_replace()
function replaces all occurrences of a search string with a replacement string. Here’s an example:
$str = "Hello, World!";
echo str_replace("Hello", "Hi", $str); // Outputs: Hi, World!
Example 3: substr()
The substr()
function returns a part of a string. Here’s an example:
$str = "Hello, World!";
echo substr($str, 0, 5); // Outputs: Hello
Best Practices
When working with PHP string functions, there are some best practices to keep in mind:
- Sanitize user input: Always sanitize user input before using string functions to prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS).
- Use appropriate functions: Choose the right string function for the task at hand. For example, if you need to perform a case-insensitive search, use
stripos()
instead ofstrpos()
. - Keep performance in mind: Some string functions can be resource-intensive, especially when working with large strings. Be mindful of performance when using these functions, especially in high-traffic web applications.
Conclusion
PHP string functions are an essential part of web development in PHP. They provide developers with the tools they need to manipulate, analyze, and work with strings effectively. By understanding the usage, examples, and best practices of these functions, developers can enhance the functionality and security of their web applications.
FAQs
Q: Are PHP string functions case-sensitive?
A: Yes, most PHP string functions are case-sensitive by default. For example, strpos()
and str_replace()
are case-sensitive.
Q: Can I create my own custom string functions in PHP?
A: Yes, you can create your own custom string functions in PHP using the function
keyword. This allows you to encapsulate a series of string manipulation operations into a single reusable function.
Q: Are PHP string functions efficient for working with large amounts of data?
A: PHP string functions can be efficient for working with large amounts of data, but IT‘s important to be mindful of performance. Some string functions, such as preg_match()
or str_word_count()
, can be resource-intensive when working with large strings.
Q: How can I improve the performance of PHP string functions?
A: To improve the performance of PHP string functions, consider using algorithms and data structures that are optimized for string manipulation. Additionally, caching and optimizing database queries can improve overall performance when working with string data.
Q: Can string functions be used for data validation?
A: While string functions can be used for some basic data validation, it’s best to use dedicated validation libraries or functions to ensure input validation and prevent security vulnerabilities.