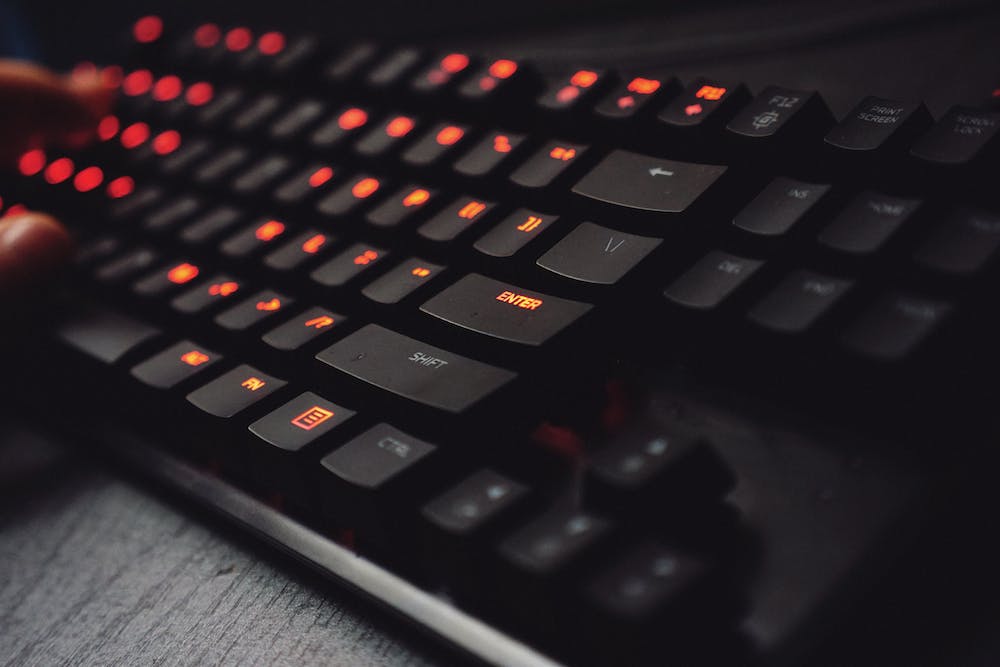
PHPExcel is a powerful tool for working with spreadsheets in PHP. IT allows users to create, read, and manipulate Excel files using PHP code. While PHPExcel provides a wide range of features for basic spreadsheet operations, there are also advanced techniques that can be utilized to enhance its capabilities for data manipulation and analysis. In this article, we will explore some of these advanced techniques for working with PHPExcel, including data import/export, chart generation, and conditional formatting.
Data Import/Export
One of the most common use cases for PHPExcel is importing and exporting data to and from Excel files. PHPExcel provides a convenient API for importing data from CSV, HTML, and other spreadsheet formats into Excel files. For example, the following code snippet demonstrates how to import data from a CSV file into an Excel spreadsheet using PHPExcel:
$inputFileType = 'CSV';
$inputFileName = 'data.csv';
$objReader = PHPExcel_IOFactory::createReader($inputFileType);
$objPHPExcel = $objReader->load($inputFileName);
Similarly, exporting data from Excel to CSV or other formats can be achieved using PHPExcel’s export functionalities. This is particularly useful for generating reports or sharing data with external systems. For example, the following code snippet exports data from an Excel spreadsheet to a CSV file:
$objWriter = PHPExcel_IOFactory::createWriter($objPHPExcel, 'CSV');
$objWriter->save('output.csv');
Chart Generation
Another advanced technique for working with PHPExcel is generating charts and graphs within Excel files. PHPExcel provides a comprehensive API for creating various types of charts, including bar charts, line charts, and pie charts. This can be particularly useful for visualizing data and presenting it in a more understandable format. The following code snippet demonstrates how to create a bar chart in an Excel spreadsheet using PHPExcel:
$chart = new PHPExcel_Chart(
'chart1', // name of the chart
null, // title
null, // legend
$dataSeries, // data series
true, // plot visible cells only
'A1', // data labels location
'A2:B5', // data values
\PHPExcel_Chart::TYPE_BAR // chart type
);
Conditional Formatting
Conditional formatting is another powerful feature in Excel that can be leveraged using PHPExcel. This feature allows users to apply formatting rules based on the cell’s value, making it easier to identify trends and patterns in the data. PHPExcel provides a rich set of APIs for applying conditional formatting to cells in Excel spreadsheets. For example, the following code snippet demonstrates how to apply conditional formatting to a range of cells in an Excel spreadsheet using PHPExcel:
$conditional1 = new \PHPExcel_Style_Conditional();
$conditional1->setConditionType(\PHPExcel_Style_Conditional::CONDITION_CELLIS)
->setOperatorType(\PHPExcel_Style_Conditional::OPERATOR_EQUAL)
->addCondition('0')
->getStyle()->getFill()->setFillType(\PHPExcel_Style_Fill::FILL_SOLID)
->getStartColor()->setARGB(\PHPExcel_Style_Color::COLOR_RED);
$conditionalStyles = $objPHPExcel->getActiveSheet()->getStyle('A1:D10')->getConditionalStyles();
$conditionalStyles[] = $conditional1;
$objPHPExcel->getActiveSheet()->getStyle('A1:D10')->setConditionalStyles($conditionalStyles);
Conclusion
In conclusion, PHPExcel is a powerful tool for working with Excel files in PHP. By leveraging its advanced techniques, such as data import/export, chart generation, and conditional formatting, users can enhance their data manipulation and analysis capabilities. These advanced techniques enable users to perform complex operations on Excel files, making PHPExcel a valuable tool for a wide range of use cases, including reporting, data visualization, and automation.
FAQs
Q: Is PHPExcel still actively maintained?
A: No, PHPExcel is no longer actively maintained. However, its successor, PhpSpreadsheet, is actively developed and maintained. It is recommended to use PhpSpreadsheet for new projects.
Q: Can PHPExcel handle large Excel files?
A: While PHPExcel can handle large Excel files, performance may be an issue for very large files. It is recommended to use PhpSpreadsheet for better performance with large datasets.
Q: Is it possible to add custom functions to PHPExcel?
A: Yes, custom functions can be added to PHPExcel using the built-in PHPExcel functions or by creating custom PHP functions and using them in conjunction with PHPExcel.
Q: Can PHPExcel generate pivot tables?
A: Both PHPExcel and PhpSpreadsheet support the generation of pivot tables. Pivot tables can be created and manipulated using the library’s APIs.
Q: Can PHPExcel be used for automated Excel file generation?
A: Yes, PHPExcel and PhpSpreadsheet can be used to generate Excel files programmatically. This can be useful for automating the creation of reports, data exports, and other tasks that involve Excel files.
By incorporating these advanced techniques into their PHPExcel workflow, users can take full advantage of the library’s capabilities for working with Excel files in PHP.