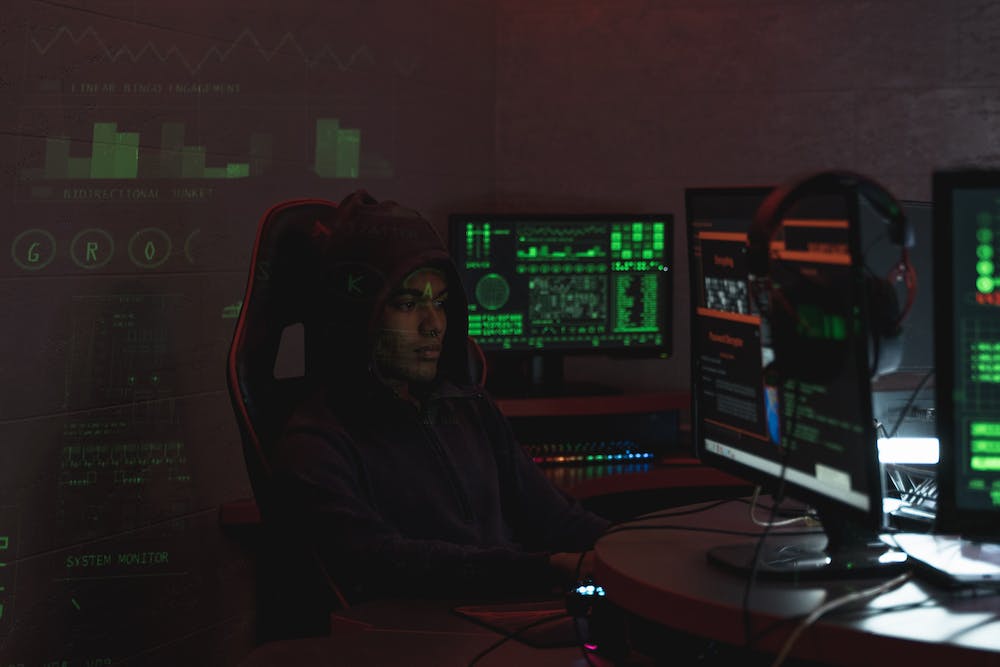
When IT comes to managing and manipulating PDF documents, iTextSharp is a powerful tool that offers advanced techniques for developers and businesses. With its comprehensive features, iTextSharp enables users to perform various operations on PDF files, such as creating, editing, merging, splitting, and securing documents.
In this article, we will delve into the advanced techniques for PDF manipulation using iTextSharp, discussing how developers can leverage its capabilities to enhance document management and streamline workflow processes.
Understanding iTextSharp
iTextSharp is a popular open-source library for creating and manipulating PDF documents in .NET. It provides a wide range of functionalities for reading, writing, and editing PDF files, making it a valuable tool for developers who require extensive control over document management.
With iTextSharp, users can perform complex operations on PDF files, such as adding text, images, and annotations, as well as extracting and manipulating content within the documents. Additionally, iTextSharp supports encryption, digital signatures, and form filling, making it a comprehensive solution for secure PDF management.
Advanced Techniques for PDF Manipulation
1. Creating PDF Documents
With iTextSharp, developers can dynamically create PDF documents by programmatically defining the content, layout, and styling. This allows for the generation of personalized and customizable PDF files, tailored to specific requirements.
using iTextSharp.text;
using iTextSharp.text.pdf;
using System.IO;
public class PdfCreator
{
public void CreatePdf(string filePath)
{
Document document = new Document();
PdfWriter.GetInstance(document, new FileStream(filePath, FileMode.Create));
document.Open();
document.Add(new Paragraph("This is a sample PDF document created using iTextSharp."));
document.Close();
}
}
In the example above, a simple PDF document is created using iTextSharp, demonstrating the basic implementation for generating a new PDF file.
2. Editing PDF Content
iTextSharp provides powerful capabilities for editing PDF content, including text manipulation, image insertion, and page restructuring. By accessing the document structure, developers can modify existing elements or add new content to the PDF file.
using iTextSharp.text.pdf;
using iTextSharp.text;
using System.IO;
public class PdfEditor
{
public void EditPdf(string sourcePath, string targetPath)
{
using (PdfReader reader = new PdfReader(sourcePath))
{
using (PdfStamper stamper = new PdfStamper(reader, new FileStream(targetPath, FileMode.Create)))
{
PdfContentByte content = stamper.GetOverContent(1);
content.BeginText();
content.SetFontAndSize(BaseFont.CreateFont(BaseFont.HELVETICA, BaseFont.CP1252, BaseFont.NOT_EMBEDDED), 12);
content.SetTextMatrix(100, 100);
content.ShowText("Edited text");
content.EndText();
}
}
}
}
In the above code snippet, the PDF content is edited by adding new text to the first page of the document using iTextSharp.
3. Merging and Splitting PDF Files
Using iTextSharp, developers can merge multiple PDF files into a single document or split a PDF document into multiple files. This functionality enables seamless integration of individual PDFs and facilitates efficient organization and distribution of content.
using iTextSharp.text.pdf;
using iTextSharp.text;
using System.IO;
using System.Collections.Generic;
public class PdfManipulator
{
public void MergePdfFiles(List sourceFiles, string targetPath)
{
Document document = new Document();
PdfCopy copy = new PdfCopy(document, new FileStream(targetPath, FileMode.Create));
document.Open();
foreach (string file in sourceFiles)
{
PdfReader reader = new PdfReader(file);
copy.AddDocument(reader);
reader.Close();
}
copy.Close();
document.Close();
}
public void SplitPdfFile(string sourceFile, string outputDirectory)
{
PdfReader reader = new PdfReader(sourceFile);
for (int i = 1; i <= reader.NumberOfPages; i++)
{
Document document = new Document();
PdfCopy copy = new PdfCopy(document, new FileStream(outputDirectory + "\\page_" + i + ".pdf", FileMode.Create));
document.Open();
copy.AddPage(copy.GetImportedPage(reader, i));
document.Close();
}
reader.Close();
}
}
The above code demonstrates the merging and splitting of PDF files using iTextSharp, showcasing how developers can efficiently manipulate PDF documents for various purposes.
4. Securing PDF Documents
iTextSharp allows for the implementation of security measures to protect PDF documents, including password encryption, digital signatures, and access permissions. By applying security settings, developers can safeguard sensitive content and control the accessibility of PDF files.
using iTextSharp.text.pdf;
using iTextSharp.text;
using System.IO;
public class PdfSecurityHandler
{
public void SecurePdf(string sourcePath, string targetPath, string ownerPassword, string userPassword, bool allowPrinting, bool allowCopy, bool allowModify)
{
using (PdfReader reader = new PdfReader(sourcePath))
{
using (PdfStamper stamper = new PdfStamper(reader, new FileStream(targetPath, FileMode.Create)))
{
stamper.SetEncryption(
null,
Encoding.UTF8.GetBytes(userPassword),
PdfWriter.ALLOW_PRINTING,
PdfWriter.ENCRYPTION_AES_128
);
stamper.SetEncryption(
Encoding.UTF8.GetBytes(ownerPassword),
Encoding.UTF8.GetBytes(userPassword),
allowPrinting ? PdfWriter.ALLOW_PRINTING : 0,
PdfWriter.ENCRYPTION_AES_128
);
stamper.Close();
}
}
}
}
The above code snippet demonstrates how PDF documents can be secured using iTextSharp, allowing for customizable encryption settings and access restrictions.
Conclusion
In conclusion, iTextSharp offers advanced techniques for PDF manipulation that empower developers and businesses to effectively manage and customize PDF documents. By leveraging its comprehensive capabilities, users can create, edit, merge, split, and secure PDF files with ease, enhancing document management and workflow processes.
FAQs
Q: Are there any licensing requirements for using iTextSharp?
A: iTextSharp is available under the AGPL license, which requires users to comply with specific terms if they choose to distribute applications that use the library. For commercial use, a commercial license is also available for iTextSharp.
Q: Can iTextSharp handle large PDF documents efficiently?
A: Yes, iTextSharp is designed to handle large PDF documents efficiently, providing optimized performance for working with extensive content and complex file structures.
Q: What are the supported platforms for iTextSharp?
A: iTextSharp is compatible with .NET and can be integrated into applications running on Windows, Linux, and macOS platforms.