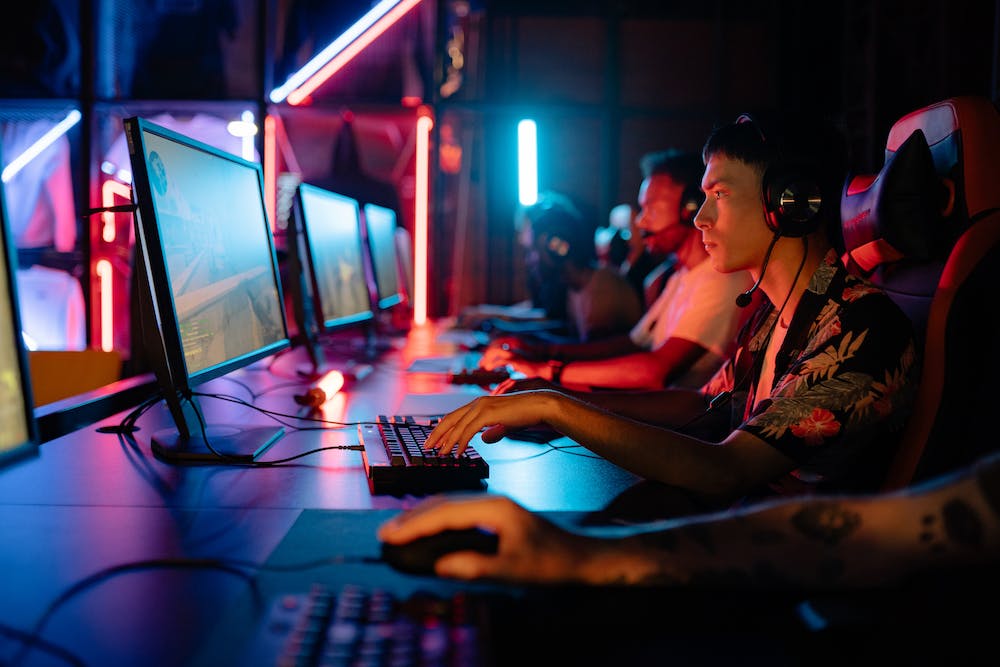
In today’s digital age, social media integration has become an essential part of web development. Facebook, being one of the largest social media platforms, is often integrated into websites and applications to boost user engagement and reach a wider audience. In this article, we will explore advanced techniques for Facebook integration using PHP, a widely used server-side scripting language for web development. We will delve into various aspects such as Facebook Login, Graph API, SDKs, and more to help you understand the intricacies of integrating Facebook into your web projects.
Facebook Login Integration
One of the most common forms of Facebook integration is allowing users to log in to a Website or application using their Facebook credentials. This not only simplifies the login process for users but also provides access to their Facebook profile information, which can be utilized to personalize their experience on the website. To accomplish this, developers can make use of the Facebook Login API provided by Facebook for seamless integration.
Using PHP, developers can utilize the Facebook PHP SDK to implement the Facebook Login feature. The SDK provides the necessary tools and libraries to authenticate users and retrieve their profile information from Facebook. IT also handles the process of obtaining access tokens, which are essential for making requests to the Facebook Graph API on behalf of the user.
Example:
// Include the Facebook PHP SDK
require_once 'facebook-php-sdk/autoload.php';
// Initialize the Facebook SDK
$fb = new Facebook\Facebook([
'app_id' => 'your-app-id',
'app_secret' => 'your-app-secret',
'default_graph_version' => 'v11.0',
]);
// Redirect users to the Facebook Login dialog
$loginUrl = $fb->getLoginUrl(['email', 'public_profile']);
// After the user logs in and approves the requested permissions
$accessToken = $fb->getAccessToken();
By integrating Facebook Login into your website or application, you can streamline the user authentication process and extract valuable user data from Facebook, such as their name, email, profile picture, and more.
Facebook Graph API Integration
Another powerful aspect of Facebook integration is accessing and manipulating Facebook’s social graph through the Graph API. With the Graph API, developers can read and write data to Facebook, such as retrieving user information, posting to a user’s timeline, and more. This enables dynamic interactions between your web application and Facebook, allowing for a seamless user experience.
PHP provides the necessary tools to make requests to the Facebook Graph API using HTTP methods such as GET, POST, DELETE, etc. Developers can use cURL or HTTP libraries such as Guzzle to send HTTP requests to the Graph API endpoints and handle the JSON responses returned by Facebook.
Example:
// Make a GET request to retrieve the user's profile
$response = $fb->get('/me?fields=id,name,email', $accessToken);
$user = $response->getDecodedBody();
// Post a message to the user's timeline
$fb->post('/me/feed', ['message' => 'Check out this article on Facebook integration!',], $accessToken);
By leveraging the Facebook Graph API in conjunction with PHP, developers can create engaging and personalized experiences for users, utilizing their Facebook data to enhance the functionality of the web application.
Webhooks and Real-Time Updates
Webhooks are a powerful tool provided by Facebook to receive real-time updates about user activity on the platform. With webhooks, developers can subscribe to various types of events such as user interactions, page updates, comments, and more. When a subscribed event occurs on Facebook, the platform will send a POST request to the specified endpoint URL, allowing for instant notification and seamless integration with your web application.
Implementing webhooks with PHP involves creating endpoint URLs to receive the webhook events and processing the incoming data to perform the necessary actions. Developers can use PHP frameworks such as Laravel, Symfony, or plain PHP to handle incoming webhook notifications from Facebook.
Example:
// Endpoint URL to receive webhook notifications
https://yourdomain.com/facebook/webhook
// Process incoming webhook event
$payload = json_decode(file_get_contents('php://input'), true);
// Handle the webhook event and perform necessary actions
By leveraging webhooks and real-time updates, developers can create dynamic and interactive experiences on their web applications, keeping users informed and engaged with the latest Facebook activities.
Conclusion
Facebook integration using PHP offers a wide range of possibilities to enhance the functionality and user experience of web applications. From seamless user authentication with Facebook Login to accessing and manipulating Facebook’s social graph with the Graph API, developers can leverage the power of Facebook to create engaging and personalized experiences for their users. By incorporating webhooks and real-time updates, developers can keep users informed and engaged with the latest Facebook activities, further enhancing the integration of the platform with their web applications.
FAQs
Q: Are there any limitations to accessing Facebook data using PHP?
A: While PHP provides powerful tools for accessing Facebook data, developers should be aware of Facebook’s platform policies and API rate limits to ensure compliance and avoid any potential restrictions on data access.
Q: Can I integrate Facebook into my web application without using a PHP SDK?
A: While using a PHP SDK such as the Facebook PHP SDK simplifies the integration process, developers can make direct HTTP requests to the Facebook Graph API using PHP’s built-in functions or HTTP libraries to achieve similar results.
Q: How can I handle errors and exceptions when integrating Facebook with PHP?
A: Developers should implement error handling and exception catching mechanisms to gracefully handle any errors or exceptions that may occur during the Facebook integration process. This ensures a smooth and reliable user experience on the web application.
Q: Is it possible to integrate Facebook integration with other PHP frameworks such as Laravel or Symfony?
A: Yes, developers can seamlessly integrate Facebook into popular PHP frameworks such as Laravel or Symfony by utilizing the built-in features and capabilities of these frameworks to handle authentication, API requests, and webhook notifications.