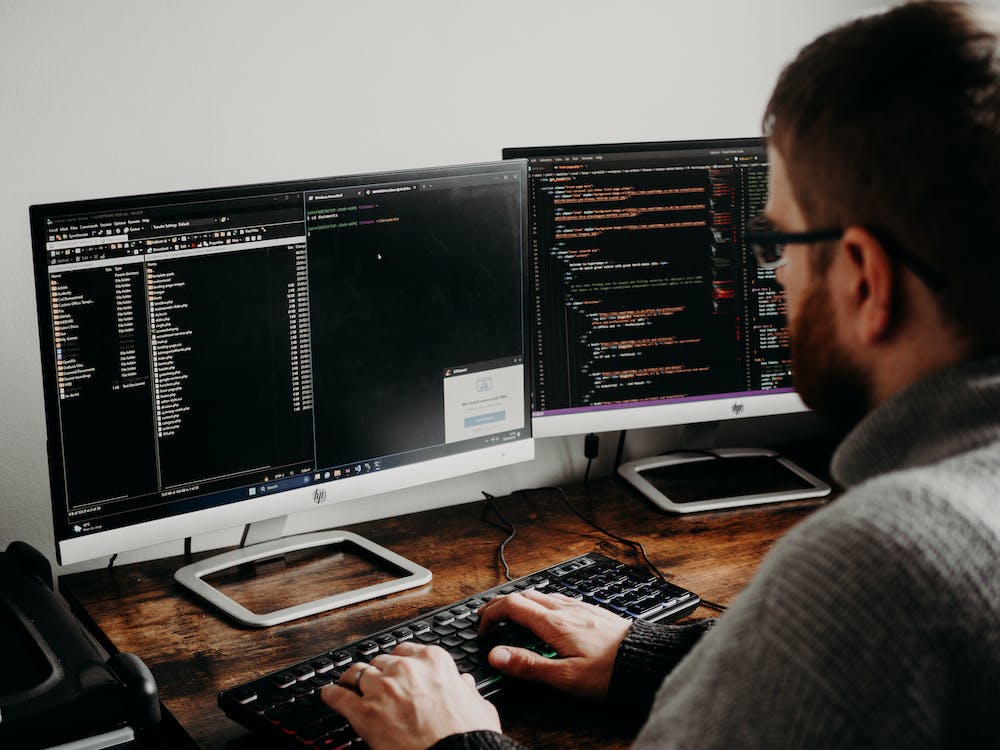
Laravel provides a powerful and elegant way to send emails using its built-in Mail class. Whether you want to send a notification to users, a newsletter to subscribers, or any other type of email, Laravel makes IT easy and convenient. In this step-by-step guide, we will walk through the process of sending emails with Laravel, from setting up your email configurations to actually sending the emails. We will also address some frequently asked questions along the way.
Step 1: Configure your Email
The first step is to configure the email settings of your Laravel application. Laravel makes IT simple to define the email driver you want to use and set up the required credentials. Open the .env file in the root directory of your Laravel application and add the following lines:
MAIL_MAILER=smtp
MAIL_HOST=smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=your-mailtrap-username
MAIL_PASSWORD=your-mailtrap-password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME="${APP_NAME}"
Make sure to replace the placeholder values with your own email service credentials. In this example, we are using Mailtrap as the email driver, but you can use any other email service provider by adjusting the configuration accordingly.
Step 2: Create an Email Class
Next, you need to create an email class that extends Laravel’s built-in Mailable class. This email class will define the content and appearance of the email you want to send. You can generate a new email class using the following Artisan command:
php artisan make:mail WelcomeEmail
This will create a new file named WelcomeEmail.php under the app/Mail directory. Open this file and update the build method to define the email’s subject, view, and any additional data you want to pass to the email view. Here is an example:
public function build()
{
return $this->subject('Welcome to our Website')
->view('emails.welcome')
->with([
'username' => $this->user->name,
'email' => $this->user->email,
]);
}
Step 3: Create the Email View
After defining the email class, you need to create the corresponding email view. Laravel provides a convenient way to build email templates using Blade, Laravel’s template engine. Create a new Blade view file (e.g., welcome.blade.php) under the resources/views/emails directory and add the HTML content of your email:
<h1>Welcome, {{ $username }}!</h1>
<p>Thank you for joining our Website. Your registered email is {{ $email }}.</p>
You can customize the email view to fit your needs, including CSS styling, images, and dynamic data insertion using Blade’s syntax.
Step 4: Sending the Email
Now that you have set up the email configuration, created the email class, and defined the email view, you can proceed to send the email. In your controller or any other appropriate place in your application, use the Mail facade to send the email using the email class and the recipient’s email address. Here is an example:
use App\Mail\WelcomeEmail;
use Illuminate\Support\Facades\Mail;
class UserController extends Controller
{
public function sendWelcomeEmail(User $user)
{
Mail::to($user->email)->send(new WelcomeEmail($user));
return "Welcome email sent successfully!";
}
}
The Mail::to method specifies the recipient’s email address, and the send method sends the email using the WelcomeEmail class. You can pass any necessary data to the email class constructor, allowing you to personalize the email content based on the recipient’s information.
FAQs
Q1: Can I send HTML emails with Laravel?
A1: Yes, you can! Laravel’s email system is designed to support HTML content out of the box. You can use Blade syntax to create HTML templates for your emails, including styling, images, and dynamic data insertion.
Q2: How can I send emails asynchronously in Laravel?
A2: Laravel provides a feature called queues that allows you to dispatch the email sending process to a background worker. By utilizing queues, your application can continue responding to user requests without waiting for the emails to be sent. To send emails asynchronously, you need to set up a queue driver and configure the required queue connection, such as Redis or Beanstalkd.
Q3: How can I handle email attachments with Laravel?
A3: Laravel’s Mailable class provides a simple and convenient way to attach files to your emails. You can use the attach method in your email class to include one or more file attachments. For example, you can attach a PDF invoice or an image to your email just by calling the attach method and passing the file path or a file instance.