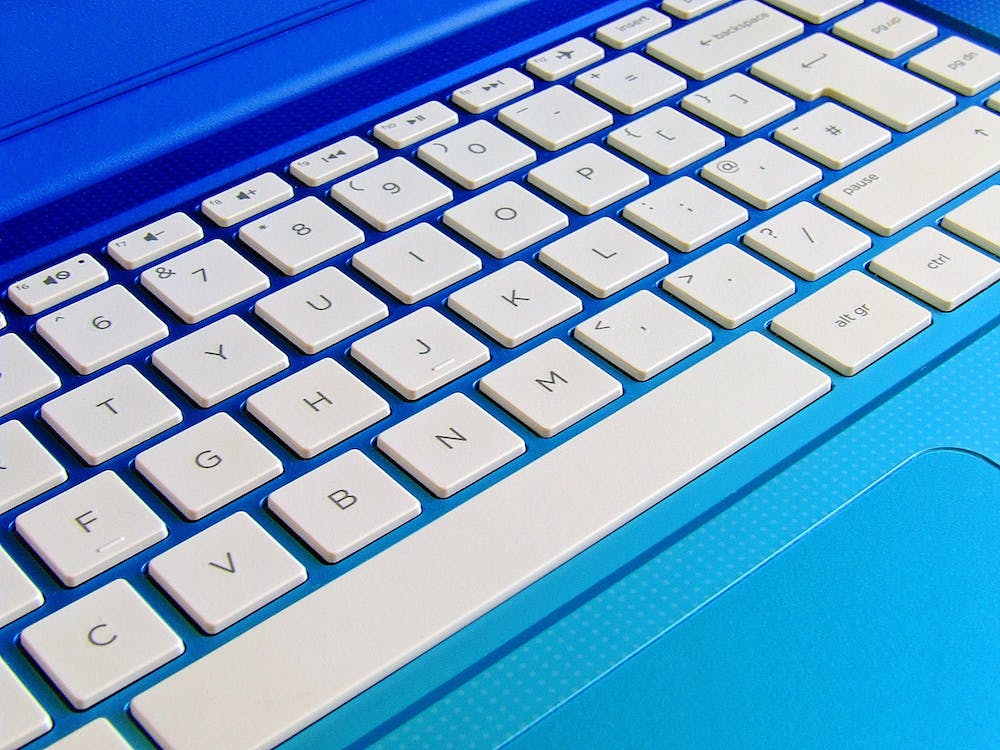
sending emails with PHP is a common practice in web development. Emails are a vital means of communication, whether IT‘s for personal or business purposes. PHP provides convenient and straightforward methods to send emails programmatically. In this guide, we will walk you through everything you need to know about sending email with PHP. From setting up the necessary configurations to handling attachments, we’ve got you covered.
1. PHP Mail Function:
The PHP mail() function is the most basic and straightforward way to send emails using PHP. IT is a built-in function that takes the recipient’s address, subject, message, and additional headers as parameters:
<?php
$to = '[email protected]';
$subject = 'Hello from PHP';
$message = 'This is a test email.';
$headers = 'From: [email protected]' . "\r\n" .
'Reply-To: [email protected]' . "\r\n" .
'X-Mailer: PHP/' . phpversion();
mail($to, $subject, $message, $headers);
?>
By specifying the recipient address, subject, message, and headers, you can send a basic email using the mail() function. However, keep in mind that the PHP mail() function relies on the server’s built-in mail functionality, which may not always be available or properly configured.
2. Using a PHP Mailer Library:
To overcome the limitations of the mail() function, there are various third-party PHP mailer libraries available that provide advanced features, including email templates, SMTP authentication, and improved error handling.
One of the popular PHP mailer libraries is PHPMailer. To use PHPMailer, you need to download the library and include IT in your project:
<?php
require 'PHPMailerAutoload.php';
$mail = new PHPMailer;
$mail->isSMTP();
$mail->Host = 'smtp.gmail.com';
$mail->Port = 587;
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'your-email-password';
$mail->SMTPSecure = 'tls';
$mail->setFrom('[email protected]', 'Webmaster');
$mail->addAddress('[email protected]', 'John Doe');
$mail->Subject = 'Hello from PHPMailer';
$mail->Body = 'This is a test email.';
$mail->send();
?>
With PHPMailer, you can configure an SMTP server and specify the sender and recipient details. IT provides a more reliable and convenient way to send emails compared to the basic PHP mail() function.
3. Sending HTML Email:
While the previous examples sent plain text emails, you can also send HTML-formatted emails using PHP. By setting the appropriate headers and body, you can include HTML tags and CSS styles:
<?php
$to = '[email protected]';
$subject = 'HTML Email Example';
$message = '<html><body><h1>This is an HTML email</h1><p>You can include HTML tags and CSS styles in emails sent with PHP.</p></body></html>';
$headers = 'MIME-Version: 1.0' . "\r\n";
$headers .= 'content-type: text/html; charset=utf-8' . "\r\n";
$headers .= 'From: [email protected]' . "\r\n";
$headers .= 'Reply-To: [email protected]' . "\r\n";
$headers .= 'X-Mailer: PHP/' . phpversion();
mail($to, $subject, $message, $headers);
?>
By setting the content-type header to “text/html” and including the HTML content in the message, you can send visually richer and more interactive emails.
4. Handling Email Attachments:
Adding attachments to emails can be crucial when sending files or documents. PHP allows you to attach files to emails by using the appropriate headers and encoding the file content using base64:
<?php
$file_path = '/path/to/file/document.pdf';
$attachment_content = chunk_split(base64_encode(file_get_contents($file_path)));
$to = '[email protected]';
$subject = 'Email with Attachment';
$message = 'Please find the attached file.';
$headers = 'From: [email protected]' . "\r\n";
$headers .= 'Reply-To: [email protected]' . "\r\n";
$headers .= 'X-Mailer: PHP/' . phpversion();
$headers .= 'MIME-Version: 1.0' . "\r\n";
$headers .= 'content-Type: multipart/mixed; boundary="boundary1"' . "\r\n";
$body = '--boundary1' . "\r\n";
$body .= 'content-Type: text/plain; charset=utf-8' . "\r\n";
$body .= 'content-Transfer-Encoding: 7bit' . "\r\n";
$body .= "\r\n";
$body .= $message . "\r\n";
$body .= '--boundary1' . "\r\n";
$body .= 'content-Type: application/octet-stream; name="document.pdf"' . "\r\n";
$body .= 'content-Transfer-Encoding: base64' . "\r\n";
$body .= 'content-Disposition: attachment' . "\r\n";
$body .= "\r\n";
$body .= $attachment_content . "\r\n";
$body .= '--boundary1--';
mail($to, $subject, $body, $headers);
?>
By specifying the appropriate content-Type header and encoding the file content, you can attach files to emails sent with PHP.
FAQs:
Q: Are PHP’s built-in mail() function and PHPMailer the only options to send emails with PHP?
A: No, there are other libraries available, such as Swift Mailer and Zend Mail, which provide similar functionality and may suit your specific needs.
Q: Can I send emails using an SMTP server without a library?
A: Yes, you can use PHP’s built-in “SMTP” class to establish a connection with an SMTP server and send emails without relying on external libraries. However, this approach requires more manual configuration and coding.
Q: How can I handle email delivery failures or error handling?
A: Using PHP’s mail() function, IT‘s challenging to capture error messages or handle delivery failures effectively. Libraries like PHPMailer provide built-in error handling mechanisms that allow you to handle failures and retrieve detailed error information if necessary.
Q: Can I send bulk emails with PHP?
A: While PHP can send bulk emails, IT is not recommended for sending large volumes of emails due to potential delivery issues and performance limitations. For bulk email sending, IT‘s advisable to utilize specialized service providers or dedicated email marketing platforms that offer efficient delivery and management features.
The process of sending emails with PHP can vary depending on your specific requirements and the tools you choose. The examples provided in this guide should give you a good starting point to explore and implement email functionality within your PHP applications.