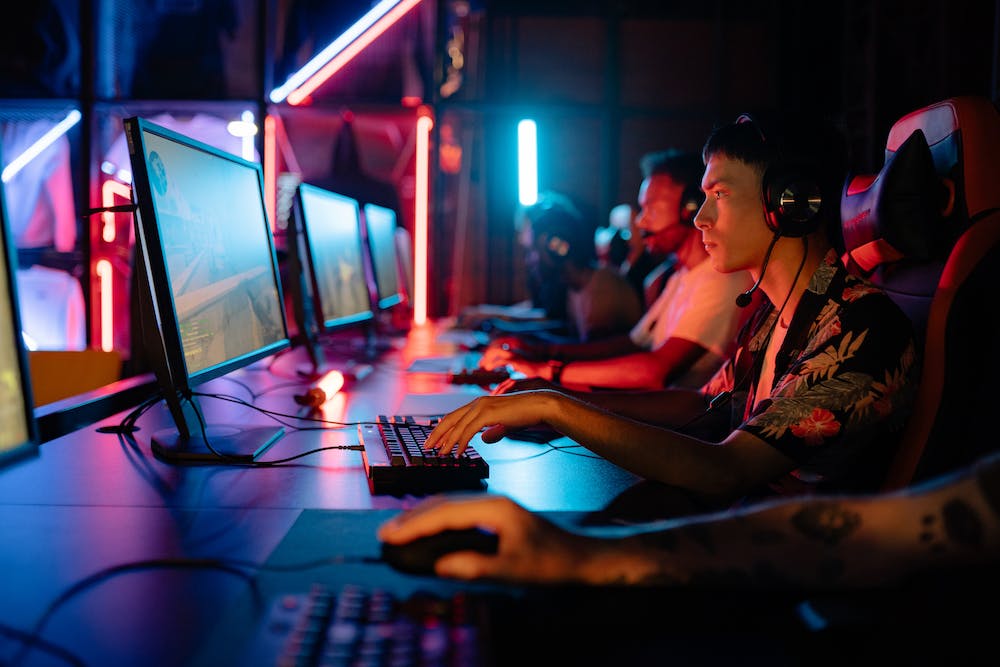
Laravel is a powerful PHP framework that offers numerous features to developers for building robust and scalable web applications. One of its standout features is its database query builder, which provides an expressive and convenient way to interact with relational databases. In this article, we will dive into the Laravel database select method and explore how IT can be used effectively.
The select method in Laravel allows you to retrieve data from one or more database tables. IT is primarily used to fetch specific columns from a table or to retrieve all columns from the table. The select method can be chained with other query builder methods to add additional conditions, sorting, or pagination to your database queries.
Let’s start by exploring the basic usage of the select method. To fetch all columns from a table, you can simply call the select method without any arguments, like this:
$users = DB::table('users')->select()->get();
This will retrieve all rows and columns from the “users” table and return them as a collection of objects. You can also specify the columns you want to fetch by passing an array of column names to the select method, like this:
$users = DB::table('users')->select('name', 'email')->get();
This will retrieve only the “name” and “email” columns from the “users” table. You can pass as many column names as you want to the select method.
In addition to selecting specific columns, you can also perform aggregate functions like count, sum, max, min, etc., using the select method. To perform an aggregate function, you can pass the function name as the first argument to the select method, like this:
$totalUsers = DB::table('users')->select(DB::raw('count(*) as total'))->first()->total;
This will retrieve the total number of rows in the “users” table and store IT in the “totalUsers” variable. Note that we used the DB::raw method to pass a raw expression to the select method.
The select method can also be used to join multiple tables and retrieve data from them. To perform a join, you can chain the join method with the select method, like this:
$users = DB::table('users')
->join('orders', 'users.id', '=', 'orders.user_id')
->select('users.name', 'orders.order_number')
->get();
This will retrieve a collection of users’ names along with their corresponding order numbers from the “users” and “orders” tables.
Now, let’s address some frequently asked questions related to the Laravel database select method:
FAQs
Q1. Can I use the select method with an Eloquent model?
Yes, you can use the select method with Eloquent models as well. Simply call the select method on the model instead of the DB facade, like this:
$users = User::select('name', 'email')->get();
Q2. How can I rename a selected column?
To rename a selected column, you can use the “as” keyword and provide a new name after the column name, like this:
$users = DB::table('users')
->select('name as full_name')
->get();
This will fetch the “name” column from the “users” table and rename IT as “full_name” in the result set.
Q3. Can I use the select method with where conditions?
Yes, you can use the select method in conjunction with where conditions. Simply chain the where method after the select method to add conditions, like this:
$users = DB::table('users')
->select('name', 'email')
->where('role', 'admin')
->get();
This will fetch the “name” and “email” columns from the “users” table for users whose role is “admin”.
In conclusion, the Laravel database select method provides a flexible and intuitive way to retrieve data from relational databases. Whether you want to fetch specific columns, perform aggregate functions, or join multiple tables, the select method has got you covered. With its extensive capabilities and Laravel’s elegant syntax, you can easily construct complex database queries with minimal effort.