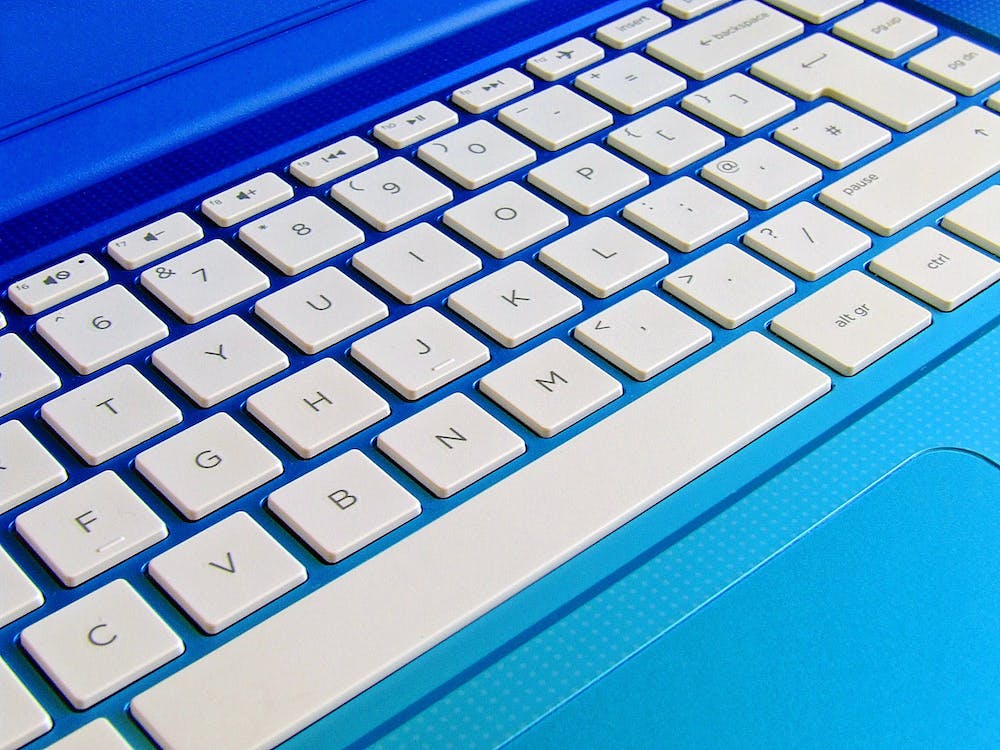
Search functionality is a crucial element of many websites and applications. Whether you are building an e-commerce platform, a blog, or a knowledge base, having a robust and efficient search feature can greatly enhance the user experience. This comprehensive guide will walk you through the process of implementing search functionality in PHP, covering everything from basic text searches to advanced filtering options.
Getting Started
Before diving into the technical details, IT‘s important to understand the different components involved in setting up search functionality. Here’s a brief overview:
- Data Source: This is the pool of data you want to search through. IT could be a database, a JSON file, or any other structured format.
- Indexing: Before you can perform searches, you need to create an index of your data. This involves extracting relevant information and storing IT in a format optimized for quick lookups.
- Search Algorithm: The algorithm determines how searches are performed. There are various approaches, including keyword matching, fuzzy searching, and relevance ranking.
- Query Processing: Once a user enters a search query, IT needs to be processed to determine the best matching results. This typically involves tokenizing the query, applying filters, and matching against the index.
Basic Text Search
The simplest form of search functionality involves matching a user’s query against a set of keywords. In PHP, you can achieve this by using regular expressions or string manipulation functions. Here’s a basic example:
$searchQuery = $_GET['q']; // Assume the query is passed as a URL parameter
$keywords = explode(' ', $searchQuery); // Split the query into individual keywords
$results = []; // Initialize an empty array to store the matching results
// Loop through each keyword and search in your data source
foreach ($keywords as $keyword) {
// Perform a search and store the matching results in the $results array
// You can use appropriate PHP functions or SQL queries depending on your data source
}
// Display the search results to the user
foreach ($results as $result) {
// Output each result to the HTML page
}
This is a basic implementation, and you can build upon IT to handle more complex scenarios. For instance, you can improve search relevancy by giving more weight to matches in titles or descriptions, or you can implement pagination to limit the number of results shown per page.
Advanced Filtering
As your data grows, you might need to provide more advanced filtering options to narrow down search results. For example, if you are building an e-commerce platform, users might want to filter products by category, price range, or availability. Here’s how you can implement advanced filtering:
$filterCategory = $_GET['category']; // Assume the category filter is passed as a URL parameter
$filterPriceMin = $_GET['price_min']; // Assume the minimum price filter is passed as a URL parameter
$filterPriceMax = $_GET['price_max']; // Assume the maximum price filter is passed as a URL parameter
$results = []; // Initialize an empty array to store the matching results
// Loop through each keyword and search in your data source
foreach ($keywords as $keyword) {
// Perform a search and store the matching results in the $results array
// Apply additional filters based on category and price range
// You can use appropriate PHP functions or SQL queries depending on your data source
}
// Display the search results to the user
foreach ($results as $result) {
// Output each result to the HTML page
}
By incorporating filters into your search functionality, you provide users with a more refined way of finding what they’re looking for. Remember to validate and sanitize user input to prevent any security vulnerabilities.
Frequently Asked Questions (FAQs)
Q: How can I improve search performance?
A: Search performance can be improved through strategies such as creating indexes, implementing caching mechanisms, and optimizing database queries. Additionally, consider using a search engine library like Elasticsearch or Solr for more advanced search requirements.
Q: Is IT possible to implement a real-time search feature?
A: Yes, IT is possible to implement a real-time search feature using technologies like AJAX. By sending asynchronous requests to your backend and dynamically updating search results without page reload, you can achieve a real-time search experience.
Q: How can I handle misspelled search queries?
A: Handling misspelled search queries can be challenging. You can consider implementing fuzzy search algorithms like Levenshtein distance or using external libraries such as SymSpell or SpellChecker to suggest corrected search terms based on similarity.
Q: Can I integrate search functionality with a third-party service?
A: Yes, many services provide search functionality as a service (SaaS). Examples include Algolia, Amazon CloudSearch, and Google Custom Search Engine. These services often offer advanced features, scalability, and ease of integration.
Q: How can I make my search results more relevant?
A: Relevance can be improved by incorporating techniques like term frequency-inverse document frequency (TF-IDF), weighting search terms based on their importance, implementing synonym dictionaries, or utilizing machine learning algorithms to personalize search results.
Implementing search functionality in PHP can be a complex task, but with some planning and careful consideration of your specific requirements, you can create a powerful search experience that will greatly benefit your users. Remember to continuously monitor and optimize the performance of your search functionality to ensure IT meets your expectations.