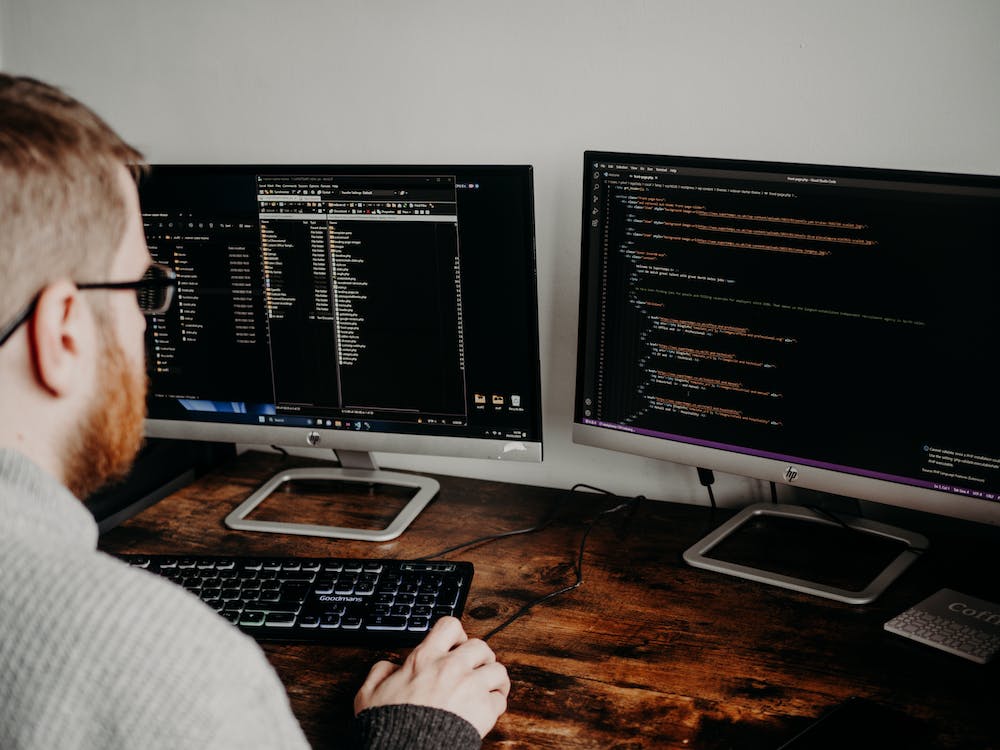
Python is one of the most widely used programming languages in the world. Its simplicity and versatility have made IT a favorite among developers. However, writing clean and error-free code can be a challenging task, especially for beginners. That’s where Python linters come in. In this comprehensive guide, we will explore what linters are, how they work, and how they can enhance your code quality.
What are Python linters?
Linters are tools that analyze your source code to identify potential errors and issues. They check your code for syntax errors, style violations, and common programming mistakes. Linters provide valuable feedback to help you write cleaner and more maintainable code. They can catch simple mistakes that might otherwise go unnoticed, such as misspellings, incorrect indentation, or unused variables.
Python linters are specifically designed to analyze Python source code. They parse your code and apply a set of predefined rules to ensure compliance with coding conventions and best practices. Linters can be integrated with code editors, IDEs, or run as standalone command-line tools.
Popular Python linters
There are several popular Python linters available, each with its own set of features and capabilities. Let’s explore some of the most widely used ones:
1. Flake8
Flake8 is a combination of several Python linting tools: Pylint, McCabe, and pycodestyle. IT provides a unified interface for all these tools and allows you to run them simultaneously. Flake8 checks for syntax errors, coding style violations, and cyclomatic complexity. IT also supports plugins and can be easily customized to fit your specific needs.
2. Pylint
Pylint is a powerful and highly configurable Python linter. IT analyzes your code against a set of predefined rules and generates a detailed report highlighting any issues found. Pylint not only enforces PEP 8 coding style guidelines but also performs more advanced checks such as code complexity, error handling, and potential bugs.
3. Pyflakes
Pyflakes is a lightweight and fast linter that focuses on detecting errors at compile time. IT analyzes your code and reports any undefined names, unused imports, or syntax errors. Unlike some other linters, Pyflakes does not perform complex style checks but rather focuses on identifying potential programming errors and mistakes.
4. Black
Black is unique among Python linters in that IT automatically formats your code according to a strict set of formatting rules. IT enforces consistent style and eliminates debates over formatting choices. Black applies changes in place, which means your code will be reformatted without altering its functionality. This makes IT a great choice for team projects with multiple contributors.
Getting started with Python linters
Now that you have an understanding of what linters are and why they are important, let’s see how you can start using them in your Python projects:
Step 1: Installing a linter
The first step is to install a Python linter of your choice. Most linters can be easily installed using the Python package manager, pip. For example, to install Flake8, you can run the following command in your terminal:
pip install flake8
Step 2: Integrating with your code editor or IDE
Linters are most effective when integrated into your code editor or IDE. This allows them to provide real-time feedback as you write code. Most popular code editors, such as Visual Studio Code, Atom, and Sublime Text, have extensions or plugins available for various linters. Install the relevant extension for your linter of choice and configure IT to enable linting.
Step 3: Running linters as command-line tools
If you prefer to run linters as standalone tools, you can use the command-line interface. Simply navigate to your project’s root directory and run the appropriate linter command. For example, to run Flake8 on your project, use the following command:
flake8
This will analyze your code and display any issues or errors found.
Frequently Asked Questions (FAQs)
Q: Can linters fix code issues automatically?
A: Linters are primarily designed to identify code issues and provide recommendations. While some linters, like Black, can automatically format your code, they generally do not fix issues automatically.
Q: Can linters replace code reviews?
A: Linters complement code reviews by catching common mistakes, enforcing coding standards, and improving code quality. However, they cannot replace the human aspect of code reviews, which involve critical thinking and expertise.
Q: Are linters only useful for large projects?
A: Linters are beneficial for projects of any size. They help maintain code quality, improve readability, and catch errors early on. Even for small projects, using a linter can save time and reduce the likelihood of introducing bugs.
Q: Can I customize the rules enforced by linters?
A: Yes, most linters allow you to customize the rules according to your preferences or project requirements. You can configure which rules to enable, disable, or modify their severity levels.
Q: Are linters only for Python?
A: No, linters exist for many programming languages. Each language has its own set of linters that cater to its specific syntax, conventions, and best practices.
Q: Are linters suitable for beginners?
A: Yes, linters are great tools for beginners as they provide instant feedback and help establish good coding habits from the start. They guide beginners towards writing cleaner, more readable code.
Now that you have a comprehensive understanding of Python linters, IT‘s time to start using them in your projects. Choose a linter that suits your needs, integrate IT into your workflow, and enjoy the benefits of cleaner, error-free code.