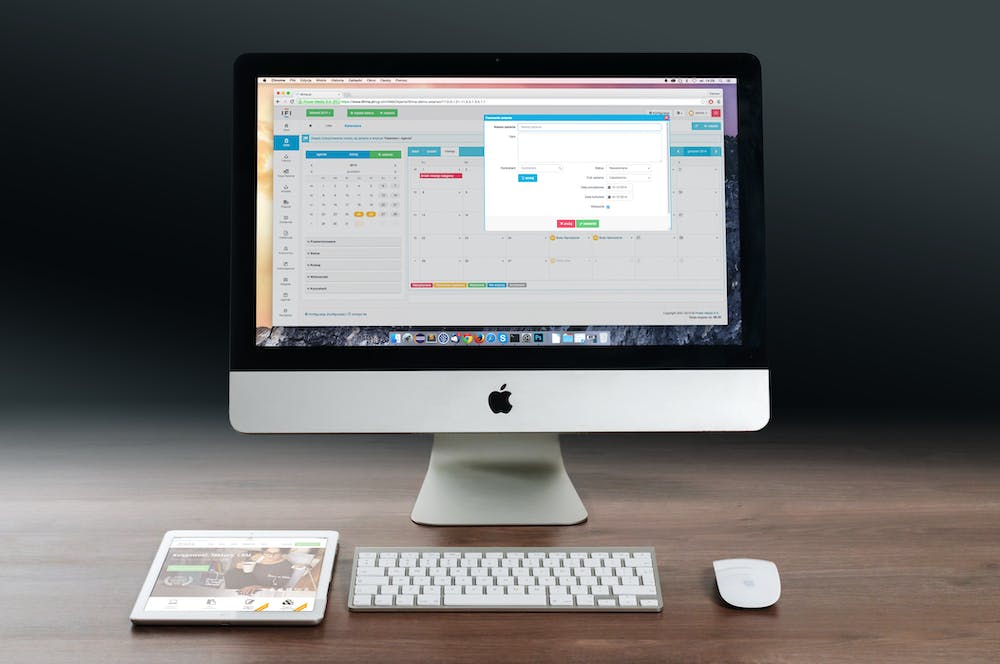
The PHP GET method is an essential tool for developers who want to retrieve data that is sent to the server. IT is widely used in web development to pass information from the client-side to the server-side. This comprehensive guide will walk you through the basics of the PHP GET method, its usage, benefits, and provide valuable insights through an FAQ section. Let’s dive in!
Understanding the PHP GET Method
The GET method is one of the two methods used to send data to the server in web development, the other being the POST method. IT allows you to pass data via the URL, making IT visible to the user. For example, when a user submits a form, the data entered is appended to the URL as query parameters.
The PHP GET method retrieves these query parameters from the URL and enables you to access and use them within your PHP script. IT is an excellent method for passing small amounts of data or retrieving data from external sources like APIs.
Using the PHP GET Method
Utilizing the PHP GET method is quite simple. Here’s an example to illustrate its usage:
<?php
if (isset($_GET['name'])) {
$name = $_GET['name'];
echo "Hello, " . $name . "!";
} else {
echo "Please provide your name.";
}
?>
In the above code snippet, we check if the ‘name’ parameter is present in the URL’s query string using the ‘isset’ function. If IT exists, we assign its value to the variable ‘$name’ using the superglobal variable ‘$_GET’. Then, we simply echo a greeting, including the user’s name if IT was provided. If the ‘name’ parameter is not present, a message requesting the user’s name is displayed.
You can pass multiple parameters using the PHP GET method simply by adding them to the URL. For example, if you have a form with ‘name’ and ‘age’ fields, the URL will look like this:
http://example.com/script.php?name=John&age=25
To access the ‘age’ parameter, you can follow the same process as above, replacing ‘name’ with ‘age’. This allows you to retrieve and manipulate various data sent through the URL.
Benefits of the PHP GET Method
The PHP GET method offers several advantages that contribute to its popularity:
- Simplicity: The GET method is straightforward to understand and implement, making IT ideal for beginners. Its simplicity extends to passing data via the URL, eliminating the need for complex data structures.
- Compatibility: The PHP GET method is compatible with all web browsers since IT relies on basic URL manipulation. This ensures that your applications can be easily accessed and used by a wide range of users.
- Flexibility: GET requests can be easily bookmarked, shared, or stored as they are represented in the URL. This enables the creation of dynamic, customizable, and easily accessible web applications.
- Data retrieval: The GET method is particularly useful when retrieving data from APIs or external sources. By passing parameters through the URL, you can easily fetch and process the required data.
- Caching: Since GET requests are idempotent, meaning they do not modify server data, they can be cached by web browsers. This can significantly improve performance by reducing the need for repeated requests.
Common PHP GET Method FAQs
Q: Can the PHP GET method be used to send sensitive data?
A: IT is recommended to avoid using the PHP GET method for sending sensitive data. Since the parameters are visible in the URL, sensitive information like passwords or personal data can be exposed. Instead, the POST method should be used, as IT sends data discreetly via the request headers.
Q: Are there any limitations to the PHP GET method?
A: The most significant limitation of the PHP GET method is the length restriction of a URL. Different browsers and servers enforce varying maximum URL lengths, typically ranging from 2,048 to 8,192 characters. If the URL exceeds this limit, data may be truncated. Additionally, certain characters or special characters may need to be encoded to ensure correct data transmission.
Q: Can I use an array as a parameter in the PHP GET method?
A: While the PHP GET method does not directly support sending an array as a parameter, you can achieve similar functionality by using array notation in the parameter names. For example, appending ‘items[]’ multiple times in the URL will allow you to retrieve an array-like structure of items on the server-side.
Q: How do I handle spaces or special characters in the URL parameters?
A: Spaces and special characters in URL parameters should be encoded to ensure correct transmission. The ‘urlencode’ function in PHP can be used to URL-encode strings, and the ‘urldecode’ function can be used to decode them back to their original form.
With this comprehensive guide to the PHP GET method, you are now equipped with the knowledge to utilize its power in your web development projects. Explore its uses, experiment with different parameter combinations, and develop dynamic applications that harness the GET method’s versatility and simplicity. Happy coding!