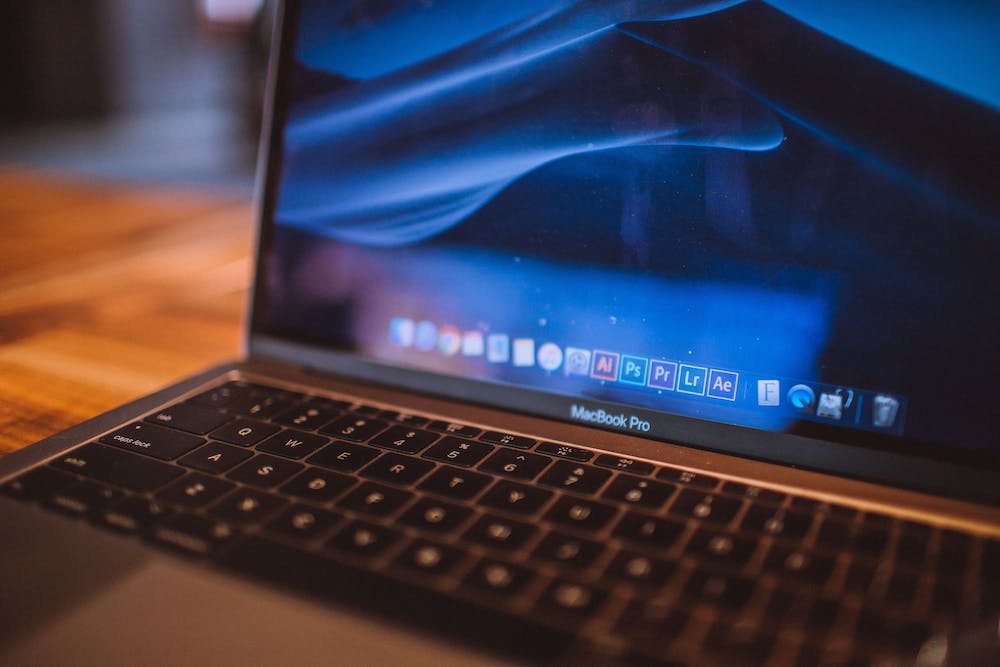
A Comprehensive Guide to PHP Foreach Loops: Explained with Examples
Introduction to PHP Foreach Loops
PHP, also known as Hypertext Preprocessor, is a widely-used programming language that is suited for web development. IT provides a set of powerful features, one of which is the foreach loop. In this comprehensive guide, we will explore the concept of foreach loops in PHP and illustrate IT with various examples.
Understanding Foreach Loops
A foreach loop in PHP is used to iterate over arrays or other iterable objects. IT allows you to access each element in the array and perform specific actions on them. The foreach loop provides an elegant and efficient way to process array elements without the need for manually managing counters or indices.
Here is the basic syntax of a foreach loop:
<?php
foreach ($array as $value) {
// code to be executed
}
?>
In the above code, $array
represents the array or iterable object you want to loop through, and $value
is a variable that holds the value of the current element in each iteration.
Examples of PHP Foreach Loops
Let’s explore some examples to demonstrate how foreach loops can be used in PHP:
Example 1: Iterating over an Array
<?php
$fruits = array("apple", "banana", "orange");
foreach ($fruits as $fruit) {
echo $fruit . "<br>";
}
?>
In this example, we have an array of fruits, and the foreach loop iterates over each element in the array, printing IT on a new line.
Example 2: Accessing Array Keys and Values
<?php
$grades = array("John" => 97, "Jane" => 85, "Alex" => 92);
foreach ($grades as $student => $score) {
echo $student . " -> " . $score . "<br>";
}
?>
Here, we have an array that associates students with their grades. The foreach loop allows us to access both the key (student name) and the value (grade) in each iteration.
Example 3: Modifying Array Elements
<?php
$numbers = array(1, 2, 3, 4, 5);
foreach ($numbers as &$number) {
$number *= 2; // multiply each element by 2
}
print_r($numbers);
?>
In this example, we are multiplying each element of the array by 2. By using the reference (&
) symbol with the $number
variable, we can modify the original array elements within the loop.
Example 4: Iterating over Multidimensional Arrays
<?php
$employees = array(
array("John", 25, "Manager"),
array("Jane", 30, "Developer"),
array("Alex", 28, "Designer")
);
foreach ($employees as $employee) {
foreach ($employee as $detail) {
echo $detail . " ";
}
echo "<br>";
}
?>
In this example, we have a multidimensional array that represents employee details. The outer foreach loop iterates over each employee, while the inner foreach loop accesses the individual details of each employee.
Conclusion
PHP foreach loops are an essential tool when working with arrays and iterable objects. They provide a convenient way to iterate over elements and perform actions on them. By understanding the concept of foreach loops and exploring different examples, you can leverage this powerful feature to enhance your PHP coding skills.
Frequently Asked Questions (FAQs)
Q: Can I use foreach loops with other iterable objects apart from arrays?
A: Yes, foreach loops are not limited to arrays. They can be used with other iterable objects in PHP, such as iterators and generators.
Q: How can I break out of a foreach loop?
A: To prematurely exit a foreach loop, you can use the break
statement. This will immediately terminate the loop execution.
Q: Can I modify array elements directly using foreach loops?
A: Yes, you can modify array elements directly by using the reference (&
) symbol in the foreach loop. This allows you to alter the original array values within the loop.
Q: Are foreach loops faster than traditional for loops?
A: In general, foreach loops have similar performance to traditional for loops. The choice between them depends on the specific use case and personal preference. However, foreach loops provide more concise and readable code when working with arrays.
Q: Can I use foreach loops with associative arrays?
A: Yes, foreach loops can be used with associative arrays. They allow you to iterate over both the keys and values of the associative array.
In conclusion, PHP foreach loops are a powerful feature that simplifies working with arrays and iterable objects. They provide a concise and efficient way to access and manipulate array elements. By understanding the basics and exploring various examples, you can apply foreach loops in your PHP projects for better code readability and maintainability.